Utilizing GPT for Seamless Integration of Cloud APIs in Python Applications
Updated on April 01, 2025

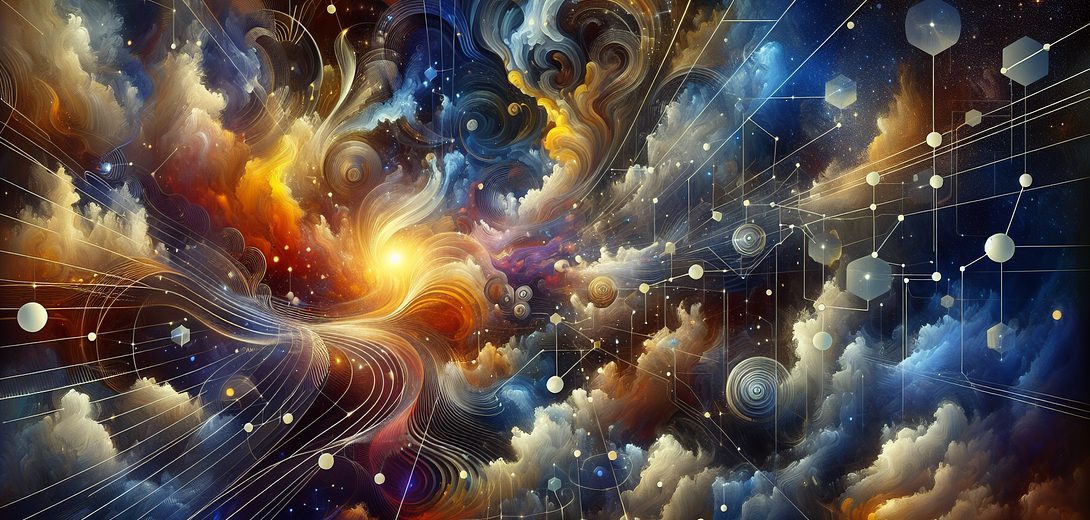
In the ever-evolving landscape of software development, integrating cloud APIs efficiently into applications is a skill that can significantly enhance functionality and scalability. Leveraging GPT models to facilitate this integration process can streamline development by automating the generation of boilerplate code, contextualizing API calls, and improving code quality. In this blog post, we will explore how to use the Cloving CLI tool to harness the power of GPT for seamless integration of cloud APIs into your Python applications.
Setting Up Cloving CLI
To start using Cloving to enhance your development workflow, you’ll first need to install and configure it.
Installation:
First, install Cloving globally using npm with the following command:
npm install -g cloving@latest
Configuration:
After installation, configure Cloving to work with your preferred AI model and set it up with your API key:
cloving config
Follow the on-screen instructions to enter your API key and select the AI model you would like to use. This step ensures Cloving is ready to generate relevant code snippets and interact with cloud APIs in your project.
Integrating Cloud APIs with Cloving
Initializing Your Project
Set up Cloving in your project directory to enable context-awareness and optimal code generation:
cloving init
This command analyzes your project and prepares it for AI-powered enhancements.
Generating API Code Snippets
One of the most efficient ways to integrate cloud APIs is to generate code snippets using Cloving’s AI:
Example:
Suppose you need to integrate the AWS S3 service into your Python application. You can generate a code snippet for uploading files to S3 as follows:
cloving generate code --prompt "Integrate AWS S3 API to upload files in Python" --files src/aws_interaction.py
The generated Python code might look like this:
import boto3
from botocore.exceptions import NoCredentialsError
def upload_to_s3(file_name, bucket, object_name=None):
"""Upload a file to an S3 bucket"""
s3_client = boto3.client('s3')
try:
response = s3_client.upload_file(file_name, bucket, object_name or file_name)
return True
except NoCredentialsError:
print("Credentials not available")
return False
Revising and Saving the Code
After generating the code, you have several options to refine and use it in your project. You can review, request explanations, revise, or save the generated code directly.
Generating Unit Tests for API Integrations
To ensure your API integrations are robust, it’s vital to test them. Cloving can help generate unit tests for your API code.
Example:
Generate unit tests for the S3 integration code:
cloving generate unit-tests -f src/aws_interaction.py
The resulting test code might be:
import unittest
from unittest.mock import patch
from src.aws_interaction import upload_to_s3
class TestAWSIntegration(unittest.TestCase):
@patch('src.aws_interaction.boto3.client')
def test_upload_to_s3_success(self, mock_s3_client):
mock_instance = mock_s3_client.return_value
mock_instance.upload_file.return_value = True
result = upload_to_s3('test.txt', 'mybucket')
self.assertTrue(result)
@patch('src.aws_interaction.boto3.client')
def test_upload_to_s3_no_credentials(self, mock_s3_client):
mock_s3_client.side_effect = NoCredentialsError
result = upload_to_s3('test.txt', 'mybucket')
self.assertFalse(result)
if __name__ == '__main__':
unittest.main()
Interactive Chat for Ongoing Integration Tasks
When dealing with complex API integrations, you might benefit from interactive chat sessions with Cloving:
cloving chat -f src/aws_interaction.py
This opens an interactive session where you can ask questions, request additional code for other AWS services, or get help troubleshooting specific API issues.
Best Practices for Integration
- Ensure Security: Always manage your API keys and secrets securely. Consider using environment variables or secret management services.
- Test Thoroughly: Generate and execute unit tests to verify that your API integration behaves as expected under different scenarios.
- Optimize Requests: Understand API limits and design your integration to handle potential failures gracefully.
- Documentation: Keep documentation up-to-date for any API endpoints used, including expected inputs and outputs.
Conclusion
Utilizing the Cloving CLI to integrate cloud APIs into your Python applications can greatly enhance your productivity and code quality. By automating code generation and facilitating interactive assistance, Cloving empowers developers to efficiently manage cloud integrations without compromising on quality. Embrace the power of AI in your development workflow and transform the way you build applications for the cloud.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.