Utilizing AI to Simplify Debugging in PHP Applications
Updated on November 27, 2024

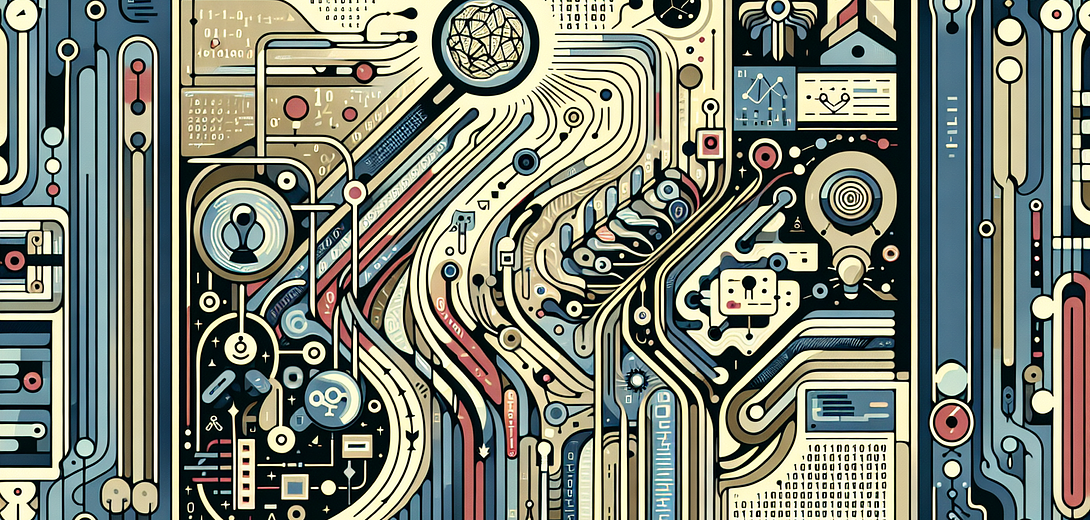
Debugging PHP applications can be a challenging task, especially when dealing with complex codebases or mysterious bugs. Fortunately, the Cloving CLI tool offers a powerful solution by integrating AI into your developer workflow to make debugging more intuitive and efficient. In this blog post, we’ll explore how to effectively use Cloving CLI to streamline your PHP debugging process, leveraging real-world examples and best practices.
Getting Started with Cloving CLI
1. Installation and Configuration
To get started with Cloving, you first need to install the CLI tool. Use npm to install it globally:
npm install -g cloving@latest
After installation, configure Cloving with your API key and desired AI model to ensure optimal functionality:
cloving config
Follow the interactive prompts to provide necessary details like your API key and preferred model.
2. Project Initialization
Initialize Cloving in your PHP project directory to prepare a context for debugging tasks:
cloving init
This command analyzes your project and generates a cloving.json
file with metadata, making it easier to understand the structure of your codebase.
Simplifying Debugging with Cloving CLI
3. Interactive Debugging Assistance
One of the most powerful features of Cloving is the interactive chat for real-time debugging assistance. Start a chat session and load the file you’re focused on:
cloving chat -f src/Controller/UserController.php
In the chat session, you can ask Cloving for help with specific bugs or coding issues:
cloving> Can you help me figure out why the user registration action is not saving data to the database?
Example Bug and AI Analysis
Consider the following PHP code snippet in a UserController.php
file:
<?php
namespace App\Controller;
class UserController
{
public function registerUser($requestData)
{
$user = new User();
$user->setName($requestData['name']);
$user->setEmail($requestData['email']);
// Missing database save method
return $user;
}
}
// Assuming somewhere a User model is defined but save method is not invoked.
AI Analysis:
“The registerUser
function is missing a step to actually save the User object to the database. This is likely why the user registration data is not being persisted. To resolve this issue, ensure that the user model’s save
method is called after setting the user data.”
Suggested Fix:
<?php
namespace App\Controller;
class UserController
{
public function registerUser($requestData)
{
$user = new User();
$user->setName($requestData['name']);
$user->setEmail($requestData['email']);
// Added save method to persist user data
$saved = $user->save();
return $saved ? $user : null;
}
}
Cloving provides you with an fix to your borked code that will allow you to quickly move forward with a fix as easily as typing Save into the chat session.
4. Automated Code Reviews
Sometimes, recurring bugs stem from deeper code issues. Use Cloving to automatically review your PHP code for potential pitfalls:
cloving generate review
Here’s an example output you might receive:
# Code Review: User Registration Enhancement
## Identified Issues
- The `saveUser` function does not handle database exceptions properly, which might lead to data loss.
- The validation steps are not consistent; consider using a validation library to streamline the process.
## Suggested Improvements
- Implement `try-catch` blocks around database operations to safely manage exceptions.
- Use Laravel's native validation features to ensure consistent input validation throughout the application.
Regularly incorporating AI-powered code reviews into your workflow can help eliminate latent bugs and improve code quality.
5. Token Estimation for Efficient Debugging
While debugging, estimating the size and complexity of your code can guide where to initiate investigations. Use the tokens
command to gauge token usage in your PHP files:
cloving tokens -f src/Controller/UserController.php
This provides insights into the complexity of your code, allowing you to target dense areas that may harbor issues.
6. Generating Test Cases for Reliable Code
Tests are crucial for reliable applications. Cloving excels at generating unit tests for your PHP application:
cloving generate unit-tests -f src/Model/User.php
This command will yield tailored test cases for your model, ensuring robust and error-free operations:
// tests/Model/UserTest.php
class UserTest extends TestCase
{
public function testUserCreation()
{
$user = new User(['name' => 'Alice']);
$this->assertInstanceOf(User::class, $user);
$this->assertEquals('Alice', $user->name);
}
public function testDuplicateUserCheck()
{
$user = new User(['name' => 'Bob']);
$result = $user->checkForDuplicates();
$this->assertFalse($result);
}
}
7. AI-Enhanced Git Operations
Finally, Cloving helps create meaningful commit messages that reflect coding changes, especially after debugging sessions:
cloving commit
Cloving will analyze your commits and propose a detailed message that captures the essence of your changes, streamlining the record-keeping aspect of version control.
Conclusion
By leveraging Cloving CLI’s AI-driven capabilities, PHP developers can simplify and expedite the debugging process, boosting efficiency and code quality. With features like interactive chat, automated code reviews, and intelligent token estimation, Cloving provides indispensable support for debugging and maintaining complex PHP applications.
Embrace AI in your PHP coding endeavors and unlock new levels of productivity and precision with the Cloving CLI tool!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.