Using GPT to Troubleshoot Deployment Issues in Docker Containers
Updated on June 26, 2024

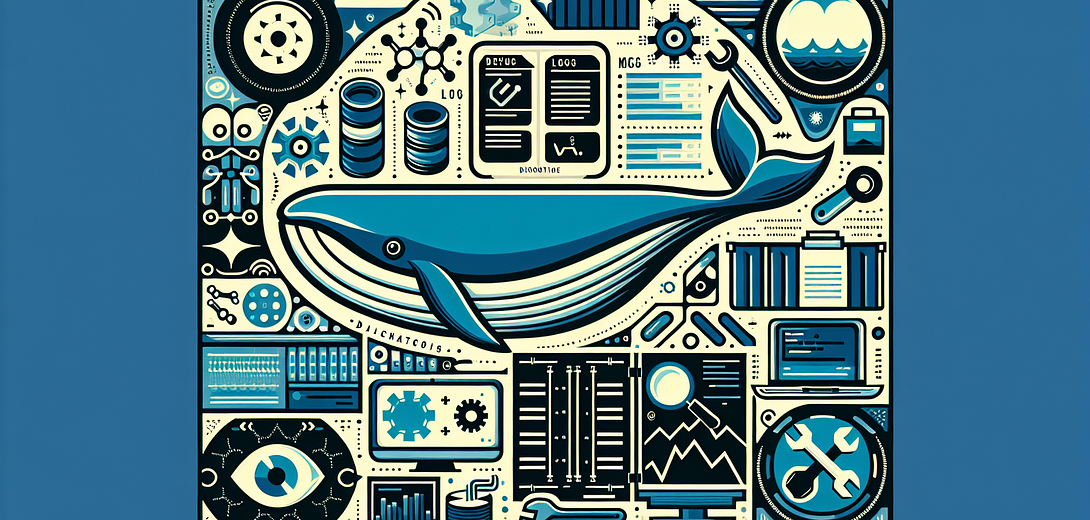
In the evolving landscape of software development, deployment issues can bring your project to a standstill, costing valuable time and resources. Deploying applications in Docker containers comes with its own set of challenges, but by leveraging cloving—integrating human creativity and intuition with the processing power of AI—we can effectively tackle these challenges.
This blog post will guide you on how to integrate GPT into your daily workflows to make troubleshooting Docker deployment issues more efficient and effective.
Understanding Cloving
Cloving marries human intuition and creativity with AI’s analytical prowess to achieve common goals. The synergy between human and machine strengths enables us to solve problems more effectively than ever before.
1. Diagnosing Common Deployment Issues
One of the first steps in troubleshooting Docker deployment issues is identifying the root cause. This is where GPT can be invaluable. By providing context and specifics about your problem, GPT can analyze the information and offer insightful diagnostics.
Example:
Suppose you’re encountering an issue where a Docker container exits unexpectedly. You can describe the problem to GPT:
My Docker container exits immediately with code 127 when trying to run a Node.js application. Here's my Dockerfile and the command I'm using to build and run the container:
FROM node:20
WORKDIR /root
COPY . .
RUN npm install
CMD ["node", "app.js"]
Build and Run Command:
docker build -t mynodeapp .
docker run mynodeapp
Example GPT Response:
The issue seems to be related to the command specified in your Dockerfile. A common reason for a container to exit with code 127 is that the executable specified in the CMD instruction is not found. Ensure that 'node' and 'app.js' are both available at runtime. Here's a diagnostic checklist:
1. Verify that 'node' is installed in the Docker image by running: `docker run -it mynodeapp node -v`.
2. Ensure 'app.js' is in the correct location. The COPY . . command places all files in /app, so 'app.js' should be present.
3. Try changing the CMD to `["node", "/app/app.js"]` to explicitly specify the path.
2. Optimizing Dockerfile and Compose Configurations
An optimal Dockerfile or docker-compose.yml
configuration is crucial for smooth deployments. GPT can help streamline your Dockerfile and docker-compose configurations by identifying inefficiencies and suggesting improvements.
Example:
If you’re looking to optimize your docker-compose.yml
for a multi-container application setup:
Here's my current docker-compose.yml. Can you suggest any improvements?
version: '3'
services:
app:
build: .
ports:
- "3000:3000"
database:
image: postgres:latest
environment:
POSTGRES_USER: user
POSTGRES_PASSWORD: password
POSTGRES_DB: mydb
Example GPT Response:
To optimize your docker-compose setup, consider the following enhancements:
1. Use build caches to speed up repeated builds:
```yaml
services:
app:
build:
context: .
dockerfile: Dockerfile
2. Add health checks to ensure services are running before dependent services start:
```yaml
database:
image: postgres:latest
environment:
POSTGRES_USER: user
POSTGRES_PASSWORD: password
POSTGRES_DB: mydb
healthcheck:
test: ["CMD-SHELL", "pg_isready -U user -d mydb"]
interval: 30s
timeout: 10s
retries: 5
3. Debugging Logs and Errors
Reading through logs to debug deployment issues can be daunting. GPT can assist in interpreting log files, identifying patterns, and providing possible solutions.
Example:
If you encounter cryptic error messages in your logs:
I'm seeing these errors in my container logs when attempting to connect to the database. Can you help me identify the issue and provide a solution?
Error logs:
2023-10-12 08:30:01,123 [ERROR] Connection refused: mydb:5432
2023-10-12 08:30:01,123 [INFO] Retrying in 3 seconds...
2023-10-12 08:30:04,123 [ERROR] Connection refused: mydb:5432
Example GPT Response:
The error "Connection refused: mydb:5432" suggests that the application cannot connect to the PostgreSQL database. Here are possible root causes and solutions:
1. Ensure the database container is running: Use `docker ps` to list running containers and verify `mydb` is active.
2. Verify network settings: Ensure the app service can access the database service. If using a custom network, both services should be on the same network.
3. Check environment variables: Ensure POSTGRES_USER, POSTGRES_PASSWORD, and POSTGRES_DB match what you’re trying to connect to.
4. Inspect firewall settings that might be blocking port 5432.
4. Writing Deployment Scripts
Automating deployment processes can eliminate many manual errors. GPT can help you write scripts to automate your Docker deployments.
Example:
To automate the Docker build and push process:
Write a bash script to build and push a Docker image to Docker Hub.
Script:
Example GPT Response:
Here's a bash script to build and push a Docker image to Docker Hub:
#!/bin/bash
# Variables
IMAGE_NAME="mynodeapp"
VERSION="latest"
DOCKER_USER="username"
DOCKER_PASSWORD="password"
DOCKER_REPO="docker.io/$DOCKER_USER/$IMAGE_NAME"
# Build the Docker image
docker build -t $DOCKER_REPO:$VERSION .
# Login to Docker Hub
echo $DOCKER_PASSWORD | docker login --username $DOCKER_USER --password-stdin
# Push the image
docker push $DOCKER_REPO:$VERSION
5. Generating Documentation and Best Practices
Maintaining well-documented deployment processes ensures clarity and consistency. GPT can generate comprehensive documentation for your deployment setups and suggest best practices.
Example:
For documenting your Docker deployment setup:
Can you generate documentation for my Docker deployment setup, including building, running, and troubleshooting steps?
Documentation:
Example GPT Response:
# Docker Deployment Setup
## Building the Docker Image
To build the Docker image, run the following command:
```bash
docker build -t mynodeapp .
Running the Docker Container
To run the Docker container, use:
docker run -d -p 3000:3000 mynodeapp
Common Issues and Solutions
Issue: Container exits immediately with code 127
Solution:
- Ensure that your
CMD
in the Dockerfile points to the correct executable. - Check for missing dependencies.
Issue: Connection refused on database port
Solution:
- Verify that the database container is running.
- Check network settings and environment variables.
## Conclusion
By integrating GPT into your troubleshooting workflow, you can harness the power of cloving to make deploying Docker containers a smoother and more efficient process. This AI-assisted approach empowers you to diagnose issues faster, optimize configurations, and automate repetitive tasks, allowing you to focus on high-level problem-solving and innovation. Embrace cloving and discover how it can transform your deployment experience.
---
Feel free to ask GPT follow-up questions to further refine your prompts, explore additional use cases, and enhance your efficiency in troubleshooting Docker deployments. Here are a few bonus prompts to get you started:
```plaintext
How can I configure GitHub Actions to automate these Docker deployment tests?
Example GPT Response:
To automate Docker deployment tests using GitHub Actions, you can use the following `workflow.yml`:
```yaml
name: Docker CI
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Docker Buildx
uses: docker/setup-buildx-action@v1
- name: Login to Docker Hub
uses: docker/login-action@v1
with:
username: ${{ secrets.DOCKER_USERNAME }}
password: ${{ secrets.DOCKER_PASSWORD }}
- name: Build and push Docker image
uses: docker/build-push-action@v2
with:
context: .
push: true
tags: ${{ secrets.DOCKER_USERNAME }}/mynodeapp:latest
Generate accompanying `docker-compose` configurations for staging and production environments.
Example GPT Response:
Here are example `docker-compose` configurations for staging and production environments:
# docker-compose.staging.yml
version: '3'
services:
app:
image: mynodeapp:staging
ports:
- "3000:3000"
database:
image: postgres:latest
environment:
POSTGRES_USER: staging_user
POSTGRES_PASSWORD: staging_password
POSTGRES_DB: staging_db
healthcheck:
test: ["CMD-SHELL", "pg_isready -U staging_user -d staging_db"]
interval: 30s
timeout: 10s
retries: 5
# docker-compose.production.yml
version: '3'
services:
app:
image: mynodeapp:latest
ports:
- "3000:3000"
database:
image: postgres:alpine
environment:
POSTGRES_USER: prod_user
POSTGRES_PASSWORD: prod_password
POSTGRES_DB: prod_db
healthcheck:
test: ["CMD-SHELL", "pg_isready -U prod_user -d prod_db"]
interval: 30s
timeout: 10s
retries: 3
What are other GPT prompts I could use to make this more efficient?
Example GPT Response:
Here are some additional prompts you might find useful:
1. "How can I create a Docker image using best practices for security?"
2. "Can you generate a Docker Compose setup for a LAMP stack?"
3. "Provide a Helm chart for deploying this Docker container in Kubernetes."
4. "What are some common security vulnerabilities in Docker containers and how to mitigate them?"
5. "Generate a Makefile for common Docker commands like build, run, and push."
By incorporating these strategies, you can unlock the full potential of AI to tackle even the most challenging deployment issues effectively.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.