Using GPT to Simplify OAuth2 Integration in APIs
Updated on October 13, 2024

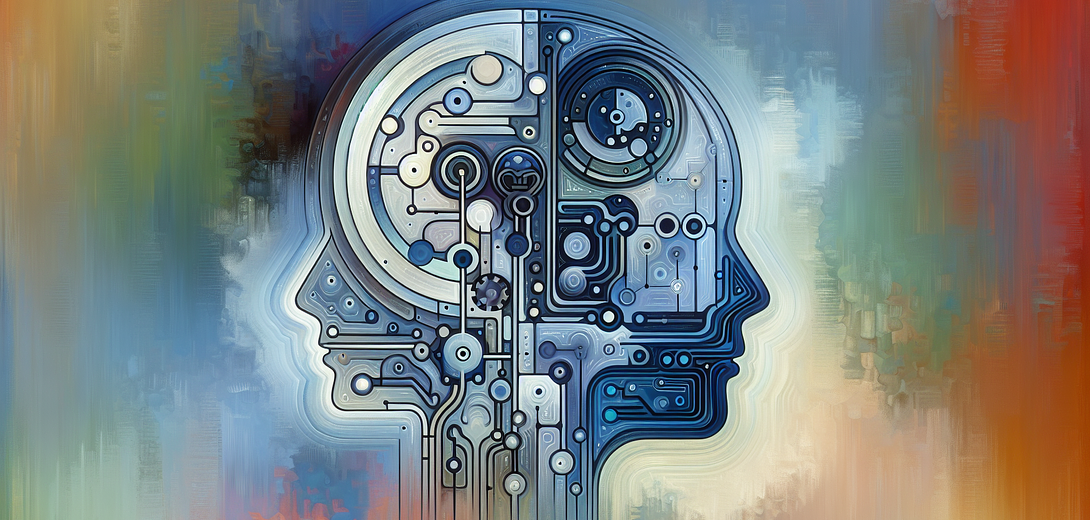
As a developer, integrating OAuth2 into your APIs is a crucial step towards securing user authentication and authorization. However, the process can be complex and time-consuming, especially if you’re new to the intricacies of OAuth2 protocols. This is where the Cloving CLI, an AI-powered command-line tool, comes in to simplify and enhance the integration process by generating code snippets and offering implementation assistance.
In this blog post, we’ll guide you through leveraging the Cloving CLI to streamline OAuth2 integration into your APIs, improving both efficiency and code quality.
Getting Started with Cloving CLI
Before diving into OAuth2 integration, ensure that the Cloving CLI is set up in your project environment.
1. Installation and Configuration
Installation:
Install Cloving globally on your machine using npm:
npm install -g cloving@latest
Configuration:
Set up Cloving with your ideal AI model:
cloving config
Follow the prompts to configure your AI model and API key preferences.
2. Initializing Your Project with Cloving
To allow Cloving to understand your project context, initialize it in your API project directory:
cloving init
This command analyzes your project setup and creates a cloving.json
file to store your application metadata and configuration.
Integrating OAuth2 with Cloving CLI
With Cloving set up, let’s proceed with using its AI capabilities to aid in OAuth2 integration.
3. Generating Boilerplate Code for OAuth2
Suppose you need to set up a Node.js Express server with OAuth2. Use Cloving to generate the initial setup code:
cloving generate code --prompt "Set up OAuth2 in a Node.js Express API" --files src/app.js
Cloving will analyze your project and generate a code skeleton that includes setting up middleware, endpoints, and configuration for OAuth2.
Example Output:
const express = require('express');
const { passport } = require('./oauth2-config'); // Assuming oauth2-config.js is generated
const app = express();
// Middleware for OAuth2
app.use(passport.initialize());
// Your existing route setups
app.listen(3000, () => {
console.log('Server running on http://localhost:3000');
});
4. Customizing and Revising the Code
Cloving also allows you to refine the generated code. Suppose you need to add a route for OAuth2 callback:
Add an OAuth2 callback route to handle authentication responses
Cloving will assist by generating or revising the necessary route handler code.
5. Generating OAuth2 Configuration
Cloving can assist in generating the OAuth2 configuration file:
cloving generate code --prompt "Create a configuration for OAuth2 using passport" --files src/oauth2-config.js
This command will output a configuration file tailored to your OAuth2 provider and environment.
Example Configuration:
const passport = require('passport');
const OAuth2Strategy = require('passport-oauth2');
// Define OAuth2 Strategy
passport.use(new OAuth2Strategy({
authorizationURL: process.env.AUTHORIZATION_URL,
tokenURL: process.env.TOKEN_URL,
clientID: process.env.CLIENT_ID,
clientSecret: process.env.CLIENT_SECRET,
callbackURL: "http://localhost:3000/auth/callback"
},
function(accessToken, refreshToken, profile, cb) {
// Find or create user logic here
return cb(null, profile);
}));
module.exports = {
passport
};
6. Interacting with Cloving Chat for Guidance
For detailed explanations or complex tasks, use Cloving’s chat feature. Start an interactive chat session to discuss OAuth2-related queries:
cloving chat -f src/app.js
During your chat session, you can ask GPT various prompts to aid your understanding or implementation of OAuth2:
- “Explain the OAuth2 authorization code flow.”
- “How do I handle token refresh in OAuth2?”
- “What are the best practices for securing OAuth2 tokens?”
- “Can you provide a code example for validating OAuth2 access tokens?”
- “How do I integrate Google OAuth2 with my Node.js app?”
- “What are the differences between OAuth2 and OpenID Connect?”
This opens an AI-guided session, helping you with explanations and code snippets relevant to OAuth2 in your context.
7. Testing and Debugging OAuth2 Integration
Once the integration is complete, Cloving can help generate tests ensuring the OAuth2 setup works as expected:
cloving generate unit-tests -f src/oauth2-config.js
This generates tests for your OAuth2 configuration, instilling confidence in your setup.
Conclusion
By integrating Cloving CLI into your development workflow, you can significantly simplify OAuth2 integration in your APIs, allowing AI to take on repetitive tasks and focus your attention on refining and enhancing the application logic. Thorough understanding and careful customization of generated content can lead to efficient, secure APIs in less time.
With tools like Cloving AI, emphasize not replacing your code-writing skills but augmenting them for more productive, effective development experiences.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.