Using GPT to Optimize Memory Usage in Python Programs
Updated on July 17, 2024

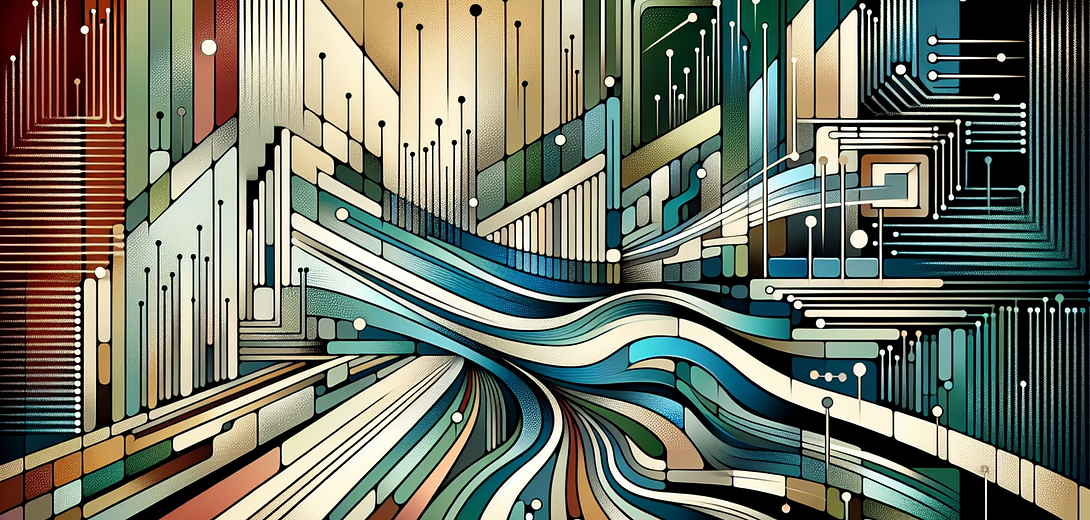
Optimizing memory usage in Python programs can be a challenging task, especially when dealing with large datasets or complex algorithms. However, by integrating human creativity and intuition with the powerful processing capabilities of AI—particularly GPT—we can streamline this process and achieve better results faster.
This is the essence of “cloving,” the synergistic relationship between human and machine. In this blog post, we’ll explore practical ways to incorporate GPT into your daily programming workflow to enhance memory optimization in Python applications.
Understanding Cloving
Cloving isn’t just about using AI tools; it’s about creating a harmonious relationship where human creativity and AI’s analytical strengths combine to solve problems more efficiently. By leveraging GPT’s capabilities, we can enhance our programming workflow, especially in optimizing memory usage.
1. Identifying Memory Leaks
One of the first steps in optimizing memory usage is identifying memory leaks. GPT can help by analyzing your code and pointing out potential areas where memory is not being managed correctly.
Example:
Suppose you have a Python script that processes large datasets, and you suspect there’s a memory leak. You can describe the issue to GPT:
I have a Python script that processes large datasets, but the memory usage keeps increasing over time. Here's a snippet of my code:
```python
def process_data(data):
results = []
for item in data:
processed = complex_operation(item)
results.append(processed)
return results
data = load_large_dataset()
result = process_data(data)
How can I fix potential memory leaks in this code?
GPT will analyze the code and might suggest using generators instead of lists to minimize memory usage or ensuring that large objects are properly deleted when no longer needed.
### 2. **Optimizing Data Structures**
Choosing the right data structures can significantly impact memory usage. GPT can provide recommendations for efficient data structures based on your specific needs.
**Example:**
If you're using a list to store millions of records and want to know if there's a more memory-efficient way, you can ask GPT:
```plaintext
I have a list in Python that contains millions of records. Is there a more memory-efficient data structure I can use?
GPT might suggest using a deque
from the collections
module or a numpy
array for lower memory overhead while maintaining performance.
3. Code Refactoring for Memory Efficiency
Refactoring your code can help reduce memory usage. GPT can assist by analyzing your code and recommending efficient practices and optimizations.
Example:
If you have a function that creates large temporary objects, you can prompt GPT for an optimized version:
Here's a Python function that creates large temporary objects. How can I refactor it for better memory efficiency?
```python
def create_large_objects(data):
temp_objects = [complex_process(item) for item in data]
return temp_objects
GPT might suggest in-place processing or using more memory-efficient objects to achieve the same result without consuming as much memory.
4. Generating Memory Profiling Scripts
Profiling your program’s memory usage can help identify bottlenecks and areas for improvement. GPT can generate scripts to profile memory usage effectively.
Example:
If you want to profile the memory usage of your functions, you can ask GPT for a script:
Generate a Python script to profile memory usage of functions in my code.
GPT will provide you with a memory profiling script using tools like memory_profiler
or guppy
:
from memory_profiler import profile
@profile
def process_data(data):
results = []
for item in data:
processed = complex_operation(item)
results.append(processed)
return results
data = load_large_dataset()
result = process_data(data)
5. Documentation and Explanation
Understanding and documenting memory optimization techniques can be complex. GPT can assist by generating clear explanations and documentation for memory-related improvements in your code.
Example:
When you need to document the memory optimization changes you’ve made, you can ask GPT:
Generate documentation for the memory optimizations applied to this Python function:
```python
def process_data(data):
results = []
for item in data:
processed = complex_operation(item)
results.append(processed)
return results
GPT will create detailed documentation, explaining the changes and their impact on memory usage, making it easier for you and your team to understand and maintain the optimizations.
Conclusion
Using GPT to optimize memory usage in Python programs exemplifies the power of cloving—combining human creativity and intuition with AI’s analytical capabilities. Integrating GPT into your optimization workflow can enhance your productivity, reduce memory overhead, and help you keep up with best practices. Embrace cloving and discover how this synergistic approach can transform your programming experience.
Bonus Follow-Up Prompts
Here are a few extra bonus prompts you could use to refine the prompts you see above:
How can I configure GitHub Actions to run these memory profiling scripts automatically for me?
And here is another:
Generate accompanying performance tests to benchmark memory usage.
And one more:
What are other GPT prompts I could use to make memory optimization more efficient?
By leveraging these prompts and the power of cloving, you can optimize memory usage more effectively in your Python programs, ensuring they run efficiently and perform reliably.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.