Using GPT to Generate Microservices with Java Spring Boot
Updated on December 23, 2024

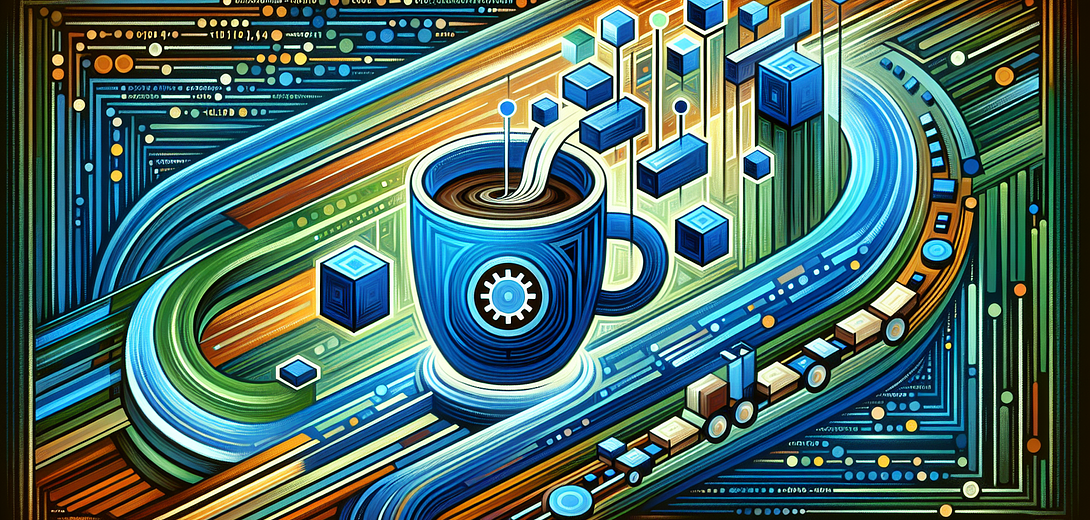
In the world of software development, microservices have become a key architectural style, offering scalability, maintainability, and productivity. As developers, we continually seek methods to streamline our workflows and elevate the quality of our code. The Cloving CLI tool provides a seamless way to incorporate AI into your Java Spring Boot microservices development, harnessing the power of GPT to generate code, automate unit tests, and enhance code quality. In this blog post, we’ll explore how to use Cloving CLI to generate microservices with Java Spring Boot, making your development process more efficient and enjoyable.
Setting Up Cloving CLI
Before we start generating Spring Boot microservices, let’s ensure Cloving is properly set up in your development environment.
Installation
Install Cloving globally using npm to get started:
npm install -g cloving@latest
Configuration
Configure Cloving to use your preferred AI model and API key:
cloving config
During the configuration process, you’ll be prompted to enter your API key and select the models you wish to use.
Initializing Your Project
For Cloving to effectively assist you, initialize it within your project directory:
cloving init
This command will analyze your project and generate a cloving.json
file, storing metadata and context information.
Generating Microservices with Cloving
Now, let’s explore how Cloving can help you generate Java Spring Boot microservices.
Example: Creating a Simple User Service
Suppose we need to create a microservice that manages user information. We can use Cloving to speed up this process.
cloving generate code --prompt "Create a Java Spring Boot microservice for managing users"
Cloving will analyze your project context and generate relevant Java Spring Boot code. An example code snippet for a basic UserService might include:
package com.example;
import org.springframework.web.bind.annotation.*;
import java.util.ArrayList;
import java.util.List;
@RestController
@RequestMapping("/users")
public class UserService {
private List<User> users = new ArrayList<>();
@GetMapping
public List<User> getAllUsers() {
return users;
}
@PostMapping
public void addUser(@RequestBody User user) {
users.add(user);
}
}
Reviewing and Revising Generated Code
Once generated, you can review and revise the microservice code. For example, if you’d like to add functionality for deleting a user by ID, Cloving can assist with that request. Here’s how you might enhance the generated code:
cloving generate code --prompt "Revise the UserService to include a method for deleting a user by ID." --files src/main/java/com/example/UserService.java
In response, Cloving might generate the following updated code:
@DeleteMapping("/{id}")
public void deleteUser(@PathVariable int id) {
users.removeIf(user -> user.getId() == id);
}
Cloving will take your prompt and adjust the code or offer explanations based on your requests, making it an interactive aid in refining and expanding generated code solutions.
Generating Unit Tests
To ensure that your service functions as intended, Cloving can help you generate unit tests:
cloving generate unit-tests -f src/main/java/com/example/UserService.java
This command will produce tailored unit tests for the UserService, strengthening code quality and reliability. Example of a generated test:
package com.example;
import org.junit.jupiter.api.Test;
import org.springframework.boot.test.context.SpringBootTest;
import static org.junit.jupiter.api.Assertions.*;
@SpringBootTest
public class UserServiceTests {
@Test
void shouldAddUserSuccessfully() {
UserService userService = new UserService();
User user = new User("John", "Doe");
userService.addUser(user);
assertTrue(userService.getAllUsers().contains(user));
}
}
Utilizing Cloving Chat for Ongoing Development
For more complex development tasks, consider using Cloving’s interactive chat feature:
cloving chat -f src/main/java/com/example/UserService.java
Engage in a dialogue where you can request code modifications, explanations, or even help with error handling.
Crafting Better Git Commit Messages
Cloving can assist in generating insightful commit messages directly within your version control workflow:
cloving commit
This command analyzes your modifications and suggests a contextual commit message, allowing you to review and edit as needed.
Conclusion
Leveraging the Cloving CLI to generate microservices with Java Spring Boot demonstrates the power of merging AI within your development framework. From generating boilerplate code to writing unit tests and crafting meaningful commit messages, Cloving offers a holistic solution to enhance productivity and code quality in your Java development projects.
As you integrate Cloving into your workflow, remember that it’s designed to complement your skills, augmenting your capabilities and accelerating your projects. By embracing this AI-powered tool, you’re opening the door to a more efficient and elevated coding experience.
Explore the possibilities with Cloving CLI and transform the way you approach microservices development in Java Spring Boot.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.