Using GPT for Seamless Java Code Integration and Generation
Updated on January 28, 2025

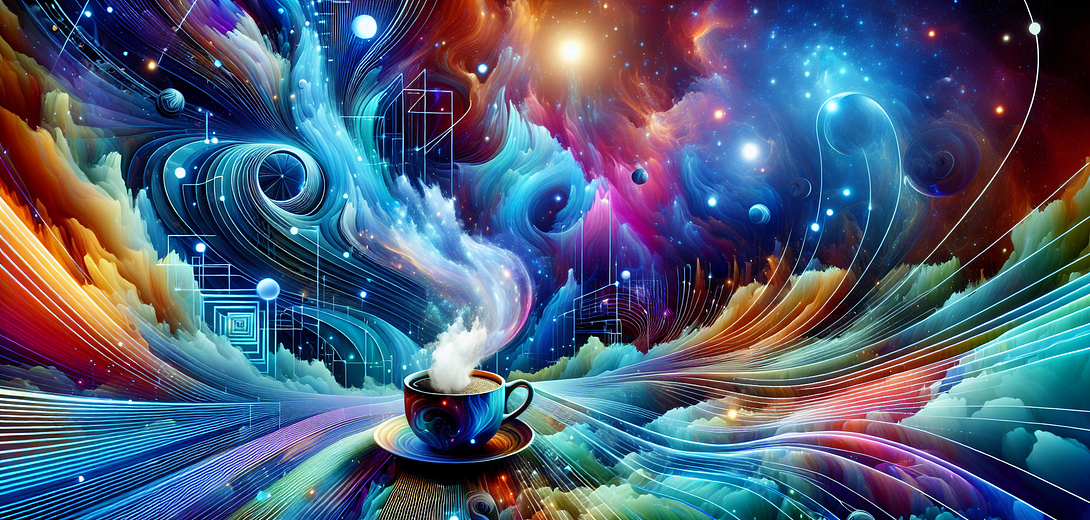
Enhancing your Java development workflow with AI has never been easier thanks to Cloving CLI. This AI-powered tool leverages GPT models to streamline your code creation process, whether it’s generating snippets, unit tests, or even writing insightful commit messages. In this tutorial, we’ll walk you through integrating Cloving CLI into your Java-based projects and show you how to maximize its potential.
Getting Started with Cloving CLI for Java
Before diving into code generation, ensure you have Cloving correctly installed and configured.
Installation:
Firstly, install the Cloving CLI via npm:
npm install -g cloving@latest
Configuration:
Set up Cloving with your API key and preferred AI model:
cloving config
Follow provided prompts to enter your API key and select the model optimal for Java development.
Initializing Cloving in Your Java Project
To allow Cloving to tailor its output to your project-specific context, it’s crucial to initialize it in your working directory:
cloving init
This command creates a cloving.json
file containing metadata to guide Cloving during code generation.
Generating Java Code
Let’s illustrate Cloving’s power in code generation with a practical Java example.
Scenario:
Suppose you’re working on a RESTful API and need to generate a Java service layer to handle user authentication. Use the following command:
cloving generate code --prompt "Create a Java service class for user authentication" --files src/main/java/com/example/service/UserService.java
Cloving will scrutinize the existing files in your project to generate a compatible code snippet, such as:
// src/main/java/com/example/service/UserService.java
package com.example.service;
import com.example.model.User;
import com.example.repository.UserRepository;
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User authenticate(String username, String password) {
User user = userRepository.findByUsername(username);
if (user != null && user.getPassword().equals(password)) {
// Authenticated successfully
return user;
}
return null; // Authentication failed
}
}
This output is directly aligned with your project setup and Java norms.
Revising and Saving Generated Code
Upon creating the code snippet, Cloving offers several options: review, revise, or save the output. To modify the generated code, you can engage the interactive chat feature:
Revise the UserService class to log unsuccessful authentication attempts.
Refine your Java class to add logging functionality or any other necessary revisions directly through Cloving.
Generating Java Unit Tests
Cloving also excels in producing unit tests—critical for validating code functionality.
Example:
Generate unit tests for the newly created UserService
:
cloving generate unit-tests -f src/main/java/com/example/service/UserService.java
This command results in relevant unit tests:
// src/test/java/com/example/service/UserServiceTest.java
package com.example.service;
import com.example.model.User;
import com.example.repository.UserRepository;
import org.junit.jupiter.api.Test;
import static org.mockito.Mockito.*;
import static org.junit.jupiter.api.Assertions.*;
class UserServiceTest {
private final UserRepository userRepository = mock(UserRepository.class);
private final UserService userService = new UserService(userRepository);
@Test
void authenticate_successful() {
User user = new User("testuser", "password");
when(userRepository.findByUsername("testuser")).thenReturn(user);
User result = userService.authenticate("testuser", "password");
assertNotNull(result);
}
@Test
void authenticate_failed() {
when(userRepository.findByUsername("invalidUser")).thenReturn(null);
User result = userService.authenticate("invalidUser", "password");
assertNull(result);
}
}
These unit tests mirror your class’s business logic, ensuring robustness and reducing bugs.
Leveraging Cloving for Effective Commit Messages
Cloving can also streamline your version control by suggesting contextual commit messages. Instead of manually writing a commit message, use Cloving:
cloving commit
This automation takes into account recent changes and outputs an apt message, such as:
Implement basic user authentication service with successful and unsuccessful login handling; add unit tests for validation.
Conclusion
Incorporating Cloving CLI into your Java development workflow empowers you to enhance productivity, maintain high code quality, and automate routine tasks. Master its commands and customize its outputs to suit your project requirements, turning AI into an invaluable ally in your development arsenal.
Use the strategies outlined here to unlock the full potential of Cloving, making your Java programming more efficient and enjoyable. Happy coding!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.