Using GPT for Facilitating Ruby Gem Development
Updated on November 19, 2024

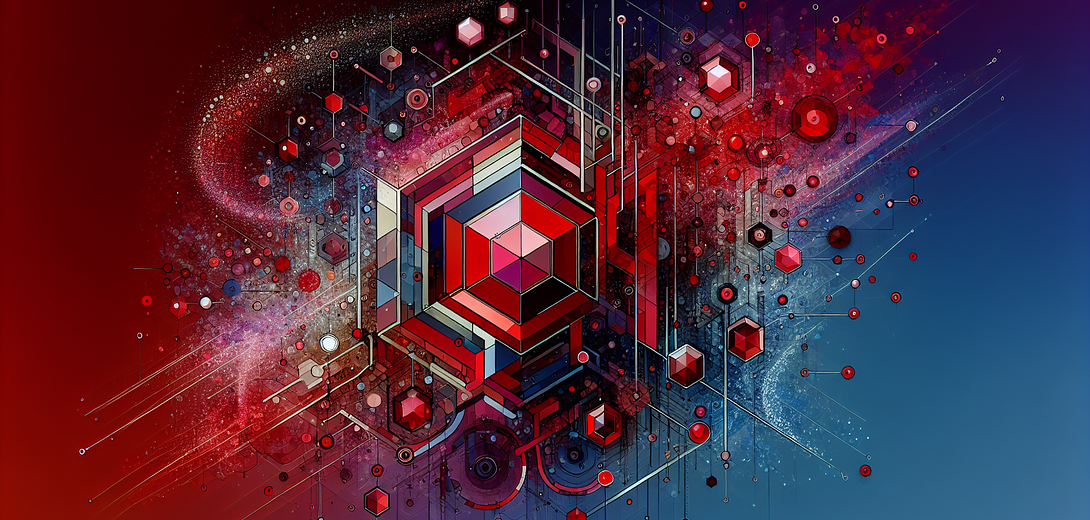
In the realm of Ruby development, creating a Ruby Gem can be a rewarding yet complex task. Fortunately, the Cloving CLI tool is here to simplify the process, leveraging AI to assist developers in generating, reviewing, and refining their code. In this post, we’ll explore how to use Cloving CLI effectively to facilitate Ruby Gem development, thereby enhancing both code quality and productivity.
Setting Up Cloving for Ruby Gem Development
Before we begin crafting a Ruby Gem with Cloving, let’s ensure the environment is properly configured.
Installation and Configuration
Installation:
You can install Cloving globally from npm with the following command:
npm install -g cloving@latest
Configuration:
Set up Cloving to utilize your preferred AI model and API credentials:
cloving config
Follow the interactive setup to configure your API key and select the appropriate AI model.
Initializing Your Ruby Gem Project
To get started with your Ruby Gem project, you must initialize your project directory with Cloving:
cloving init
This step adds essential metadata to your project, allowing Cloving to understand your project’s context better.
Using Cloving to Develop a Ruby Gem
Let’s explore how Cloving CLI can assist you in developing a Ruby Gem with practical examples.
1. Generating Boilerplate Code
You can quickly generate the basic structure and object interface for your Ruby Gem. For instance, if you wish to create a gem named “SuperGem,” you can prompt Cloving:
cloving generate code --prompt "Create a Ruby gem called SuperGem with basic structure and an example module."
Cloving will interpret the context and generate necessary components such as directories, Rakefile
, gem_spec.rb
, and an initial module template. Here’s a piece of the generated Ruby module:
# lib/super_gem.rb
module SuperGem
class Error < StandardError; end
def self.greet(name)
"Hello, #{name}"
end
end
2. Enhancing Gem Functionality
To start expanding your Gem’s capabilities, let’s build upon our “SuperGem” by integrating functionality that connects to the Leonardo.ai API. This API interaction can allow our gem to generate images based on specified parameters.
Connecting to Leonardo.ai API
To interface with the Leonardo.ai API via our gem, let’s incorporate a method that sends a request to generate an image.
Here’s some sample code that the Cloving CLI might generate for making a request.
# lib/super_gem.rb
require 'net/http'
require 'json'
require 'uri'
module SuperGem
class Error < StandardError; end
API_ENDPOINT = "https://cloud.leonardo.ai/api/rest/v1/generations"
# Method to generate an image using Leonardo.ai API
def self.generate_image(prompt, width = 512, height = 512)
uri = URI.parse(API_ENDPOINT)
request = Net::HTTP::Post.new(uri)
request.content_type = "application/json"
request["Authorization"] = "Bearer #{ENV['LEONARDO_API_KEY']}"
request.body = JSON.dump({
"height" => height,
"modelId" => "6bef9f1b-29cb-40c7-b9df-32b51c1f67d3", # Example model ID
"prompt" => prompt,
"width" => width
})
req_options = {
use_ssl: uri.scheme == "https"
}
response = Net::HTTP.start(uri.hostname, uri.port, req_options) do |http|
http.request(request)
end
response.body
rescue StandardError => e
raise SuperGem::Error, "Image generation failed: #{e.message}"
end
end
3. Generating Unit Tests
Unit testing is crucial in maintaining the reliability of your Ruby Gem. Cloving can generate RSpec test cases for the methods in your gem:
# spec/super_gem_spec.rb
RSpec.describe '::generate_image' do
it 'generates an image with the specified prompt' do
allow(Net::HTTP).to receive(:start).and_return(double("response", body: "Image data"))
response = SuperGem.generate_image("An oil painting of a cat")
expect(response).to eq("Image data")
end
end
end
4. Facilitating Code Reviews
A thoughtful code review can highlight areas of improvement and potential errors. Use Cloving to conduct an AI-assisted code review:
cloving generate review
This analysis provides insights into code efficiency, adherence to conventions, and potential refactoring opportunities.
6. Streamlining Commits with AI
Lastly, ensure meaningful documentation of changes using Cloving for crafting commit messages:
cloving commit
Cloving can auto-generate commit messages reflecting the nature of changes, streamlining your Git workflow.
Conclusion
Building a Ruby Gem from scratch can be a daunting prospect but Cloving CLI can bolster this development process, from generating initial code structures to iteratively improving functionality. With Cloving, you’ll boost productivity, quality, and project scalability, creating gems that are both efficient and enriching to your development experience.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.