Using GPT for Efficient Vue.js Form Validation Logic
Updated on March 31, 2025

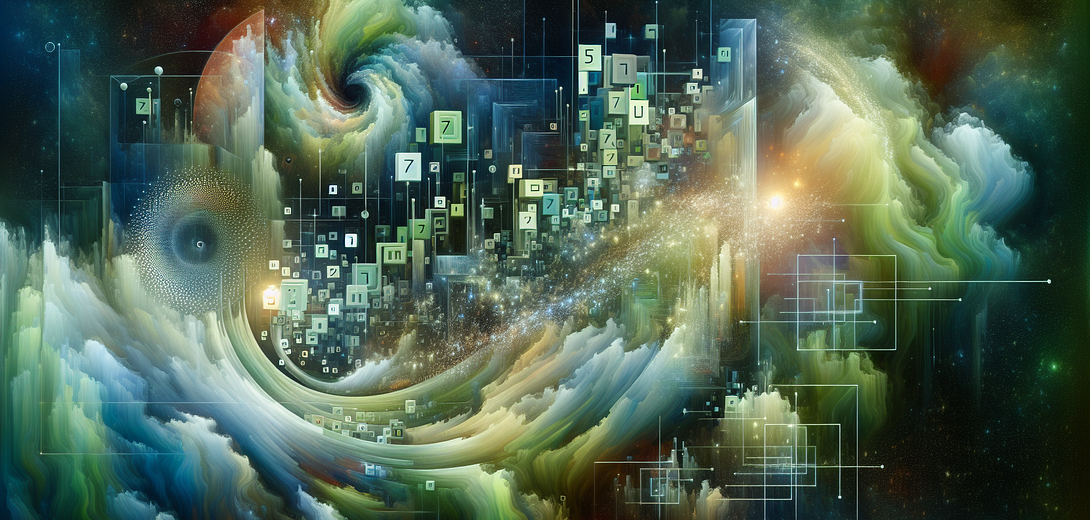
In the modern development world, creating robust front-end form validations can significantly enhance the user experience and maintain data integrity on web applications. Vue.js, a progressive JavaScript framework, simplifies the development of interactive web interfaces. However, writing custom validation logic can sometimes become a tedious task. This is where the Cloving CLI—a powerful AI augmentation tool—comes into play. Cloving leverages GPT models to help you generate efficient form validation logic, allowing you to integrate AI into your Vue.js projects effectively.
Getting Started with Cloving CLI
Before diving into generating form validation logic, let’s set up the Cloving CLI in your development environment.
Installation and Configuration
Install Cloving:
First, ensure you have Cloving CLI installed globally:
npm install -g cloving@latest
Configure Cloving:
Once installed, configure Cloving with your API key and preferred models:
cloving config
Follow the prompts to enter your API key and select the models best suited for your needs.
Setting Up a Vue.js Project
To integrate Cloving with your Vue.js form validation, initialize your project using the Cloving initialization command:
cloving init
This command will create a cloving.json
file with necessary metadata, helping Cloving understand your project’s context better.
Generating Form Validation Logic
Let’s explore how Cloving can assist in generating efficient and reliable form validation logic using Vue.js.
Example: Validating a Registration Form
Suppose you need to add validation to a registration form requiring a username, email, and password. You can use Cloving’s generate code
command tailored for this scenario.
Generate Validation Logic:
cloving generate code --prompt "Generate Vue.js form validation for username, email, and password in a registration form"
Here’s a sample output of the generated validation logic:
<template>
<form @submit.prevent="validateForm">
<div>
<label>Username</label>
<input v-model="username" @blur="validateUsername"/>
<span v-if="errors.username">{{ errors.username }}</span>
</div>
<div>
<label>Email</label>
<input v-model="email" @blur="validateEmail"/>
<span v-if="errors.email">{{ errors.email }}</span>
</div>
<div>
<label>Password</label>
<input type="password" v-model="password" @blur="validatePassword"/>
<span v-if="errors.password">{{ errors.password }}</span>
</div>
<button type="submit">Register</button>
</form>
</template>
<script>
export default {
data() {
return {
username: '',
email: '',
password: '',
errors: {}
}
},
methods: {
validateUsername() {
this.errors.username = this.username.length < 3 ? 'Username must be at least 3 characters.' : '';
},
validateEmail() {
const emailPattern = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
this.errors.email = !emailPattern.test(this.email) ? 'Invalid email address.' : '';
},
validatePassword() {
this.errors.password = this.password.length < 6 ? 'Password must be at least 6 characters.' : '';
},
validateForm() {
this.validateUsername();
this.validateEmail();
this.validatePassword();
if (!this.errors.username && !this.errors.email && !this.errors.password) {
alert('Form is valid!');
// Proceed with form submission logic
}
}
}
}
</script>
Improving and Revising the Validation Logic
Once generated, you can review and improve the validation logic using Cloving’s interactive command options. If needed, request further revisions:
Add validation for password complexity: at least one uppercase letter and one special character
Cloving will refine the validation logic accordingly based on your additional requirements.
Utilizing Cloving Chat for Complex Validation
For more detailed or complex scenarios, use the Cloving chat feature, providing a continuous interactive session:
cloving chat -f src/components/RegistrationForm.vue
In this session, you can interact with the AI to tailor the validation logic further, ask for explanations, or make adjustments to meet specific project needs.
Best Practices for AI-Assisted Form Validation Logic
- Use Clear Prompts: Provide clear and specific prompts for more accurate code generation.
- Review and Test: Always review the generated code and conduct thorough testing to ensure it meets your application’s requirements.
- Interactive Sessions: Utilize Cloving’s interactive chat sessions for refining and improving generated code.
- Leverage Unit Tests: Use Cloving to generate unit tests for your validation logic, ensuring it performs accurately and reliably.
Conclusion
Integrating AI into your Vue.js workflow using the Cloving CLI enables more efficient development practices, especially for commonly repeated tasks like form validation. By leveraging GPT models, you can streamline your form validation process, focusing more on creating dynamic web applications. Embrace Cloving and discover its potential to not only enhance your productivity but also improve the overall quality of your code.
By following these guidelines and practices, you’ll be well on your way to mastering AI-powered form validation in Vue.js applications, ensuring a seamless user experience and maintaining data integrity across your web projects.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.