Using GPT for Constructing Scalable Backend Systems in Node.js
Updated on April 01, 2025

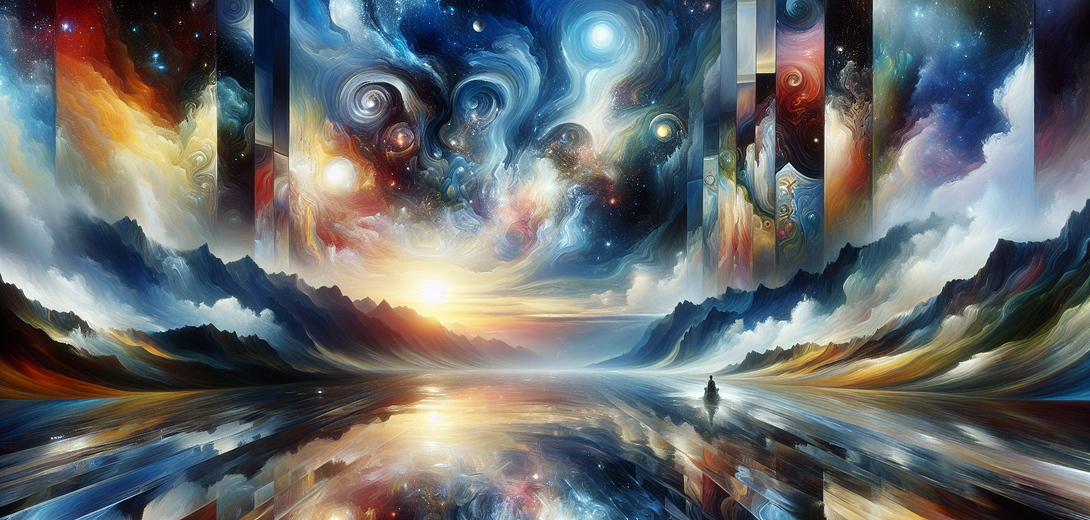
Building scalable backend systems is a critical component of modern software engineering, especially when using a platform like Node.js. With the integration of AI-powered tools like the Cloving CLI, developers can streamline their coding processes, ensuring efficiency and accuracy. This tutorial will explore how you can leverage the Cloving CLI tool, utilizing GPT, to construct scalable backend systems in Node.js.
Setting Up the Environment
Before diving into code generation and backend design, it’s crucial to set up Cloving CLI within your Node.js environment.
Installation
First, ensure you have Cloving installed globally:
npm install -g cloving@latest
Configuration
Configure Cloving to work with your preferred AI model:
cloving config
This command will guide you through setting up your API key and selecting the AI model that suits your needs.
Project Initialization
To utilize Cloving effectively, initialize it within your Node.js project directory:
cloving init
This command will analyze your project and prepare it for context-aware code generation, creating a cloving.json
file to store metadata and settings.
Code Generation with Cloving
Constructing a REST API
One of the popular tasks in Node.js is creating RESTful APIs. Suppose you need to generate boilerplate code for an Express.js REST API. Cloving makes this straightforward:
cloving generate code --prompt "Create an Express.js REST API for user management" --files src/api/users.js
This command will utilize your project context to create a basic structure for the API, which might look like the following in the src/api/users.js
file:
const express = require('express');
const router = express.Router();
// GET users
router.get('/', (req, res) => {
res.send('GET all users');
});
// POST user
router.post('/', (req, res) => {
res.send('POST new user');
});
// PUT user
router.put('/:id', (req, res) => {
res.send(`PUT user with ID ${req.params.id}`);
});
// DELETE user
router.delete('/:id', (req, res) => {
res.send(`DELETE user with ID ${req.params.id}`);
});
module.exports = router;
Scaling with Microservices
For creating a microservice architecture, you can generate separate service layers for different functionalities using the same approach:
cloving generate code --prompt "Set up a microservice for handling orders" --files src/services/orderService.js
This will generate a new service layer dedicated to order handling that you can incorporate into your microservice architecture.
Enhancing Code with Cloving Chat
For complex coding tasks or when you need real-time assistance, Cloving chat is your go-to tool. To start an interactive chat session with code context, run:
cloving chat -f src/api/users.js
Engage in a robust Q&A session, asking for code modifications or explanations:
cloving> How do I add middleware for user authentication in this API?
The AI will generate the necessary code snippets and steps to include user authentication middleware.
Unit Testing with Cloving
Ensuring your code is robust requires comprehensive tests. Generate test cases for your REST API using Cloving:
cloving generate unit-tests -f src/api/users.js
This will draft test cases for each endpoint, essential for maintaining a scalable system.
const request = require('supertest');
const app = require('../app');
describe('GET /users', () => {
it('should return all users', async () => {
const res = await request(app).get('/users');
expect(res.statusCode).toEqual(200);
expect(res.body).toHaveProperty('users');
});
});
Version Control with AI-Powered Commits
Streamline your Git workflow by leveraging Cloving for generating contextual commit messages:
cloving commit
This command will generate insightful commit messages based on recent changes, helping your team understand project evolution at a glance.
Conclusion
Integrating AI tools like the Cloving CLI into your Node.js projects can significantly enhance productivity and code quality. By automating repetitive tasks, generating scalable system components, assisting with real-time coding queries, and providing intelligent commit messages, Cloving helps developers focus on building robust systems rather than managing mundane details.
Remember, while AI can automate and assist, your expertise in crafting thoughtful, scalable architectures remains invaluable. Use Cloving as a powerful extension of your development toolkit for a more efficient and effective coding experience.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.