Using GPT for Cleaner and More Maintainable Swift Code
Updated on November 17, 2024

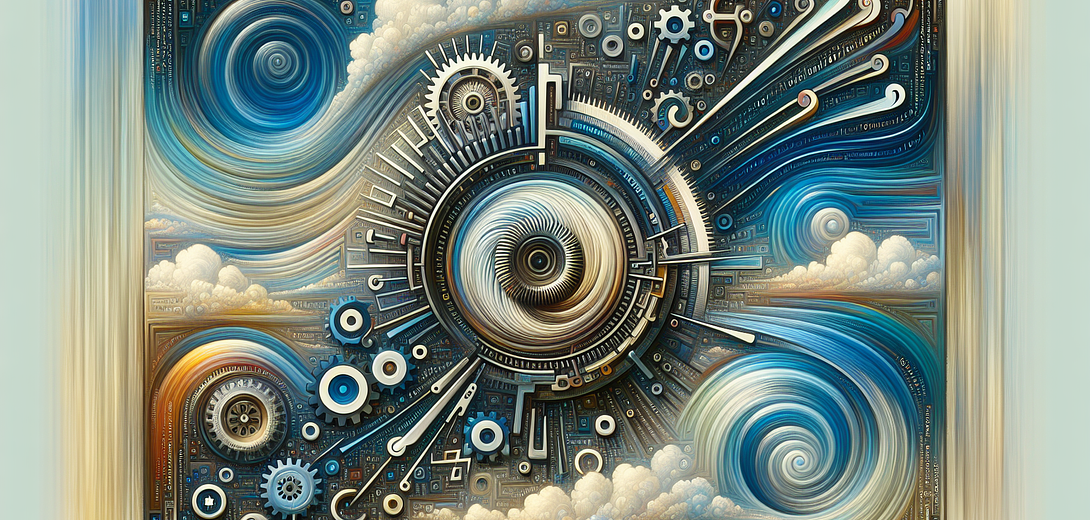
In the world of software development, writing clean and maintainable code is crucial for the long-term success of any project. Integrating Cloving CLI into your Swift development workflow can drastically improve your team’s productivity by automating mundane tasks and enhancing code quality with AI assistance. This post will guide you through harnessing the power of Cloving CLI to produce cleaner and more maintainable Swift code.
Getting Started with Cloving CLI
Before diving into using Cloving CLI for Swift development, let’s set it up in your environment.
Installation:
To use the Cloving CLI, install it globally through npm:
npm install -g cloving@latest
Configuration:
Set up Cloving with your API key and the model you prefer:
cloving config
This command will prompt you to enter your API key and select the desired AI model.
Setup Cloving in Your Swift Project
For Cloving to effectively enhance your code, it must understand your project’s context. Initialize Cloving in your Swift project:
cloving init
Running this command in your project’s directory will create a cloving.json
file, providing context about your application’s structure and settings.
Harnessing Cloving to Generate Swift Code
Cloving CLI doesn’t just assist in Python or JavaScript development; it’s equally proficient in generating Swift code. Let’s explore how you can use Cloving to improve your Swift code quality.
Example: Generating a Model Class
Suppose you are working on an iOS app and need a model class for user data. Instead of writing it manually, Cloving’s code generation tool can help:
cloving generate code --prompt "Generate a Swift class for a User with properties for id, name, and email" --files Models/User.swift
Generated Code:
class User {
var id: String
var name: String
var email: String
init(id: String, name: String, email: String) {
self.id = id
self.name = name
self.email = email
}
func displayName() -> String {
return "User: \(name)"
}
}
Iterative Refinement
Once you’ve generated code, you might want to refine it. Let’s say you’d like to add a method for validating the email format:
cloving chat --files Models/User.swift
You can instruct Cloving to iterate over the current User class and add the desired functionality:
Add a method to validate the email format in the User class
Refined Code:
class User {
var id: String
var name: String
var email: String
init(id: String, name: String, email: String) {
self.id = id
self.name = name
self.email = email
}
func displayName() -> String {
return "User: \(name)"
}
func validateEmail() -> Bool {
let emailRegEx = "[A-Z0-9a-z._%+-]+@[A-Za-z0-9.-]+\\.[A-Za-z]{2,}"
let emailPred = NSPredicate(format:"SELF MATCHES %@", emailRegEx)
return emailPred.evaluate(with: self.email)
}
}
Cloving automatically makes changes for you, letting you simply save the changes, test them in your environment, and commit them if you are satisfied. This streamlines the development process, allowing developers to focus more on high-level logic and less on boilerplate code.
Improving Code Maintenance with Unit Tests
Unit tests are essential for maintaining your code. Cloving can help generate unit tests for your Swift classes:
cloving generate unit-tests -f Models/User.swift
Generated Unit Tests:
import XCTest
@testable import YourApp
class UserTests: XCTestCase {
func testUserInitialization() {
let user = User(id: "1", name: "John Doe", email: "[email protected]")
XCTAssertEqual(user.name, "John Doe")
XCTAssertEqual(user.email, "[email protected]")
}
func testEmailValidation() {
let user = User(id: "1", name: "John Doe", email: "[email protected]")
XCTAssertTrue(user.validateEmail(), "Email validation should pass")
}
}
Real-Time Assistance with Cloving Chat
For real-time problem-solving and code improvement suggestions, use the Cloving chat function. It acts as an AI pair programmer for complex Swift problems:
cloving chat -f Models/User.swift
Through the interactive chat, you can ask Cloving to optimize your code for performance or follow Swift’s best practices, thereby making your codebase more maintainable.
Improving Commit Messages
Using informative commit messages is crucial for maintaining a clear project history. Cloving can assist in generating more descriptive commit messages:
cloving commit
This command analyzes your changes and suggests a well-structured commit message that you can review and adjust.
Conclusion
Leveraging Cloving CLI for generating and maintaining Swift code brings efficiency and sophistication to your development process. It helps automate repetitive tasks, enhance code quality, and keep the codebase clean and maintainable. By integrating Cloving into your daily workflow, you’ll significantly improve your productivity and the quality of code you deliver.
Embrace this tool to ensure your Swift projects are built on a foundation of cleanliness, maintainability, and scalability.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.