Using GPT for AI-Driven Code Synthesis in Kotlin Applications
Updated on January 02, 2025

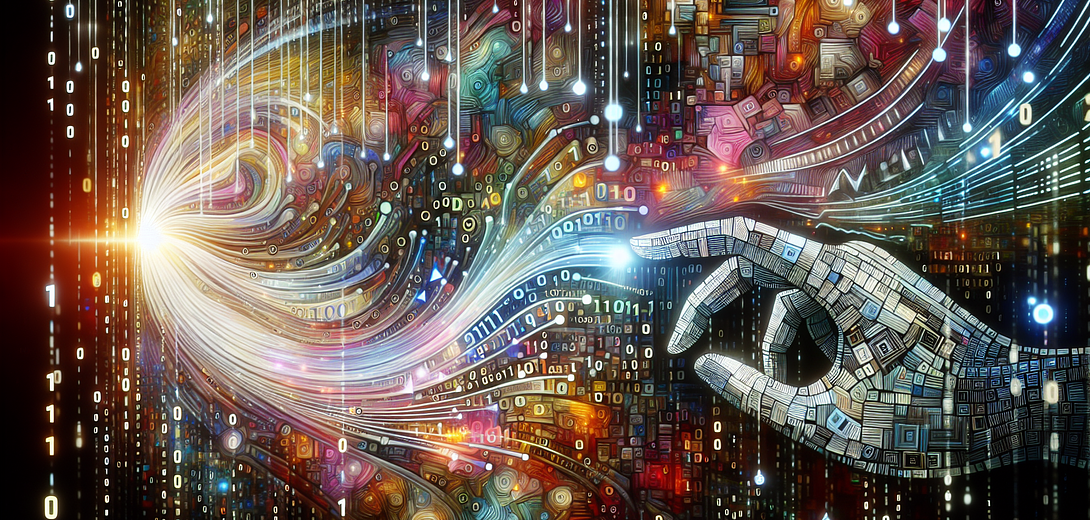
The advent of AI in software development has not only changed the way we write code but also accelerated the speed at which we deliver high-quality software. With Cloving CLI, a powerful tool for integrating AI into your Kotlin development workflow, you can transform your coding process by using AI-driven code synthesis powered by GPT. In this post, we’ll explore how to incorporate Cloving CLI into your Kotlin applications to enhance productivity and ensure code quality.
Getting Started with Cloving CLI
Cloving CLI acts as your intelligent assistant, allowing you to leverage AI for code generation, unit testing, and more. To make the most of Cloving, follow these steps to set up your environment.
Installation
You can install Cloving globally using npm:
npm install -g cloving@latest
Configuration
Before you begin using Cloving, configure it with your API key and preferred AI model to ensure optimal performance:
cloving config
Follow the interactive prompts to set up your API key and preferred models. This setup allows Cloving to tap into the power of AI effectively.
Initializing Your Project
To let Cloving understand the context of your project, initialize it in your Kotlin project’s directory:
cloving init
This command creates a cloving.json
file containing metadata about your application, which Cloving uses to generate context-aware code.
AI-Driven Code Synthesis in Kotlin
Once your project is set up, you can leverage Cloving to generate Kotlin code seamlessly. Let’s dive into practical examples and best practices to integrate AI-generated code into your Kotlin applications.
Generating Kotlin Code
For instance, if you need to create a function that adds two numbers in Kotlin, you can ask Cloving to handle this:
cloving generate code --prompt "Create a Kotlin function to add two numbers"
Cloving will automatically analyze the project context and provide a relevant code snippet:
fun addNumbers(a: Int, b: Int): Int {
return a + b
}
Generating Unit Tests for Kotlin
Ensuring your code is robust requires thorough testing. Cloving can assist you in generating unit tests for your Kotlin functions:
cloving generate unit-tests -f src/AddNumbers.kt
For the addNumbers
function, Cloving might generate:
// src/AddNumbersTest.kt
import org.junit.Test
import kotlin.test.assertEquals
class AddNumbersTest {
@Test
fun testAddition() {
assertEquals(5, addNumbers(2, 3))
assertEquals(0, addNumbers(-1, 1))
}
}
These unit tests help verify that your function behaves as expected under different scenarios.
Interactive Chat for Problem Solving
For more complex tasks or when you need continuous assistance, utilize Cloving’s interactive chat mode:
cloving chat -f path/to/your/KotlinFile.kt
In this chat, you can interact with Cloving’s AI to ask questions, request code snippets, or obtain clarifications:
Generate a Kotlin data class for storing user information
Cloving generates:
data class User(
val id: Int,
val name: String,
val email: String
)
You can further request revisions or explanations within this dynamic interface.
Efficient Code Reviews and Commit Messages
Cloving aids in the review process as well. By using AI-powered code reviews, you can get a second opinion on your code quality. Additionally, Cloving helps you generate insightful commit messages, aligning them with the code changes:
cloving commit
This might produce a commit message like:
Implement Kotlin data class for user information handling
Such contextual messages ensure clarity and traceability in your version control system.
Best Practices for AI-Driven Development
-
Prompt Clarity: When generating code, ensure your prompts are precise to receive the most accurate results.
-
Iterative Feedback: Use Cloving’s interactive features to iterate over the generated code, providing feedback to improve AI-generated results.
-
Unit Testing: Always generate and run unit tests for AI-synthesized code to verify correctness and prevent potential errors.
-
Integration with Version Control: Regularly use Cloving’s commit functionalities for well-documented version control practices.
-
Exploration and Learning: Treat Cloving as a learning tool, understanding the AI’s reasoning behind code suggestions and gaining insights into Kotlin best practices.
Conclusion
Integrating AI into your Kotlin workflow through Cloving CLI is an exciting way to enhance productivity and code quality. By leveraging AI-driven code synthesis, you can streamline your development process, focus on critical tasks, and deliver high-quality software efficiently. Embrace the power of AI and see how Cloving can redefine your development experience in Kotlin applications.
Remember, the aim of Cloving is to complement your skills, not replace them. Use it as a potent ally in your coding toolkit to boost your productivity and enhance your software development practices.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.