Using GPT for Advanced JavaScript Code Snippet Generation
Updated on December 25, 2024

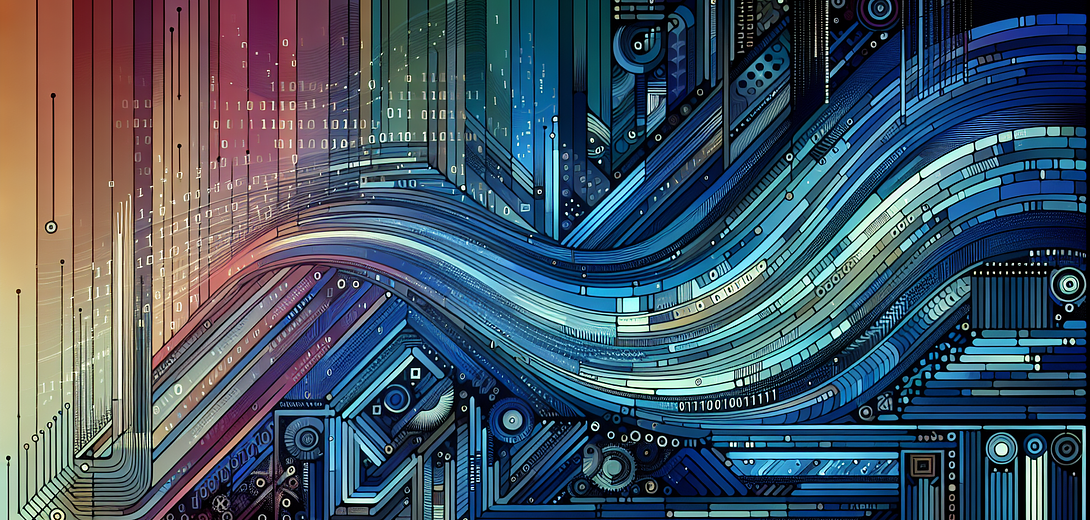
In the modern software development landscape, leveraging AI to automate routine coding tasks is increasingly becoming essential. The Cloving CLI, powered by GPT, offers developers an advanced tool for generating high-quality JavaScript code snippets rapidly and efficiently. In this tutorial blog post, we will guide you on how to utilize Cloving CLI to supercharge your JavaScript coding workflow by generating precise code snippets, saving time and enhancing code quality.
Getting Started with Cloving CLI
Before we begin crafting JavaScript snippets, let’s ensure Cloving is properly set up on your machine.
Step 1: Installation
To start using Cloving CLI, you need to install it globally via npm:
npm install -g cloving@latest
Step 2: Configuration
Configure Cloving to use your preferred AI model:
cloving config
Follow the prompts to input your API key and select the models you’ll be working with.
Step 3: Initialize Your Project
To enable Cloving to understand the intricacies of your project’s context, initialize it in your project’s root directory:
cloving init
This command reads your project structure and sets the necessary configuration in cloving.json
.
Generating JavaScript Code Snippets
Example: Creating a Function to Flatten an Array
Suppose you need a utility to flatten a deeply nested array. With Cloving, you can generate this function with ease:
cloving generate code --prompt "Create a JavaScript function to flatten an array of arrays" -f src/utils/arrayUtils.js
Upon execution, Cloving analyzes your prompt and can output something like:
function flattenArray(arr) {
return arr.reduce((flat, toFlatten) => {
return flat.concat(Array.isArray(toFlatten) ? flattenArray(toFlatten) : toFlatten);
}, []);
}
The output is a concise and effective JavaScript function that can now be saved into your file.
Best Practices with Code Generation
- Review Understandability: Always review the generated code to verify logic consistency and performance efficiency.
- Interactive Mode: Use the
--interactive
flag to iteratively refine your code with real-time feedback from Cloving. - Contextual Files: For more contextual relevance, add files using the
--files
option, providing Cloving additional context.
Utilizing the Interactive Chat
For more interactive and precise coding tasks, Cloving offers a chat feature. This can be particularly beneficial for complex JavaScript logic or when exploring new libraries and APIs.
cloving chat -f src/components/ExampleComponent.js
In the chat session, you can request, revise, and gain insights into various JavaScript implementations:
cloving> Can you write a function to debounce an event handler in JavaScript?
Certainly! Here's an example of a debounce function:
```javascript
function debounce(func, wait) {
let timeout;
return function(...args) {
const context = this;
clearTimeout(timeout);
timeout = setTimeout(() => func.apply(context, args), wait);
};
}
This chat feature provides an interactive platform to collaborate with Cloving for your coding tasks, making it an invaluable tool for complex scenarios.
Generating Unit Tests
Cloving also supports generating unit tests, which are essential for ensuring code reliability:
cloving generate unit-tests -f src/utils/arrayUtils.js
This automatically generates unit tests tailored to your code, enhancing your project’s robustness.
import flattenArray from './arrayUtils';
describe('flattenArray', () => {
it('should flatten a nested array', () => {
const input = [1, [2, [3, 4], 5], 6];
expect(flattenArray(input)).toEqual([1, 2, 3, 4, 5, 6]);
});
});
Conclusion
Integrating Cloving CLI for JavaScript code snippet generation harnesses the power of GPT to accelerate your development process significantly. By automating repetitive coding tasks, you can focus more on problem-solving and designing intuitive user experiences. Whether it’s unit tests, utility functions, or interacting with complex algorithms, Cloving enables you to optimize your workflow with AI-driven insights and capabilities.
Elevate your JavaScript projects with Cloving CLI and enjoy the productivity boost that comes with AI augmentation. Remember, as AI helps with code generation, human oversight is key to maintaining creativity and ensuring quality in coding practices. Embrace Cloving CLI today and redefine how you build with JavaScript!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.