Using GPT and Cloving to Get Started with the Google Business Profile API
Updated on April 01, 2025

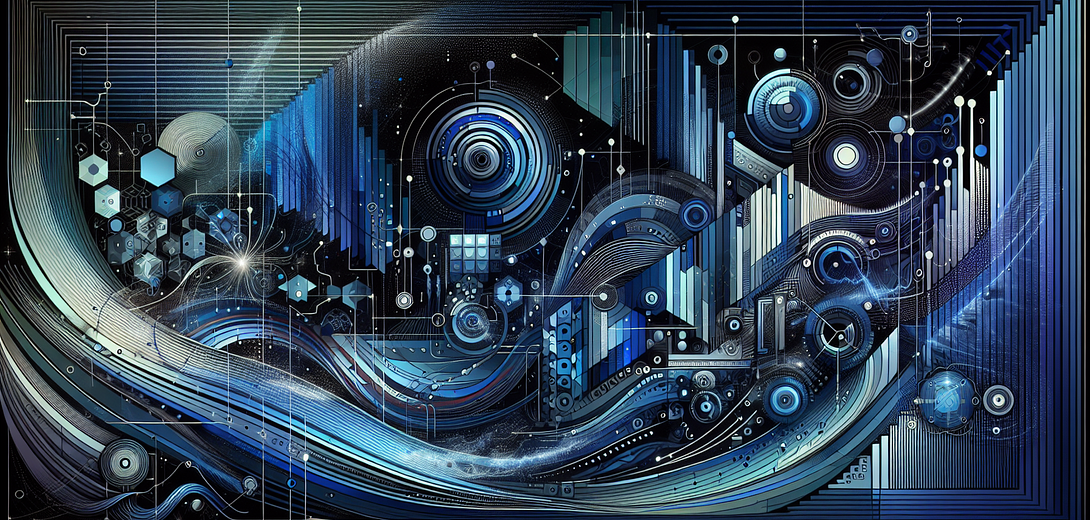
In today’s digital age, leveraging APIs effectively can provide immense value, especially when managing business information through platforms such as Google Business Profile. Integrating AI tools like Cloving with GPT capabilities can streamline your development process, allowing you to focus less on boilerplate code and more on building meaningful features. In this blog post, we’ll explore how to use Cloving CLI to get started with the Google Business Profile API, enabling you to manage business data with ease.
Step 1: Setting Up Your Environment
Before you start coding, you need to set up your environment with Cloving CLI. This step is crucial for enabling AI-driven features tailored to your project.
Install Cloving
Install Cloving globally using npm:
npm install -g cloving@latest
Configure Cloving
To access API-related features, you’ll need to configure Cloving with your API key and preferred models:
cloving config
Follow the interactive prompts to set up your API key and select your preferred AI model.
Step 2: Initializing Your Project
Once Cloving is configured, initialize it in your project to provide context about the Google Business Profile API integration:
cloving init
This command analyzes your project directory and prepares it for effective AI-assisted development.
Step 3: Understanding the Google Business Profile API
The Google Business Profile API allows you to manage multiple aspects of business details like locations, attributes, and user reviews. Having a clear understanding of its endpoints and data structure is essential for effective integration.
Step 4: Generating Request Handlers
With Cloving, you can efficiently generate code to handle common API requests. Let’s see how Cloving can help you write a GET request to retrieve business locations.
cloving generate code --prompt "Create a Node.js function to retrieve business locations using the Google Business Profile API" --files src/api.js
The AI will analyze and understand the context of the Google Business Profile API and create a function for you:
import axios from 'axios';
async function getBusinessLocations(apiKey) {
const url = `https://mybusiness.googleapis.com/v4/accounts/{accountId}/locations?key=${apiKey}`;
try {
const response = await axios.get(url);
return response.data;
} catch (error) {
console.error('Error fetching business locations:', error);
throw error;
}
}
Step 5: Reviewing and Modifying Generated Code
After Cloving generates code, you can review, modify, or save it to your files. If you want to refine the generated function to include error handling or logging, you can do so via the interactive prompt, allowing real-time collaboration with AI:
Add logging for successful API response
This modification can enhance the reliability and supportability of your code.
Step 6: Crafting Unit Tests
Ensuring that your functions work correctly is crucial. Use Cloving to generate unit tests for the getBusinessLocations
function:
cloving generate unit-tests -f src/api.js
This command creates pertinent unit tests in a dedicated test file, ensuring robustness.
import { getBusinessLocations } from './api';
import axios from 'axios';
jest.mock('axios');
describe('getBusinessLocations', () => {
it('should fetch business locations successfully', async () => {
const data = { locations: [{ name: 'Business 1' }] };
axios.get.mockResolvedValue({ data });
const result = await getBusinessLocations('testApiKey');
expect(result).toEqual(data);
});
it('should handle errors', async () => {
const errorMessage = 'Network Error';
axios.get.mockRejectedValue(new Error(errorMessage));
try {
await getBusinessLocations('testApiKey');
} catch (e) {
expect(e.message).toBe(errorMessage);
}
});
});
Step 7: Interactive Code Assistance
Whenever you encounter complex problems or need interactive support, Cloving’s chat functionality can be invaluable:
cloving chat -f src/api.js
This opens a session where you can work interactively on challenges, leveraging AI’s context-aware support.
Step 8: Committing Your Changes
Once your integration is complete, use Cloving to generate meaningful commit messages:
cloving commit
This helps you maintain a clean, informative commit history.
Conclusion
Integrating AI-powered tools like Cloving in your API development enhances productivity and code quality. By taking advantage of Cloving’s capabilities, such as code generation, interactive chat sessions, and meaningful commit generation, you can efficiently integrate the Google Business Profile API and focus on delivering business value. Remember, Cloving serves as a supplementary resource to enrich your development acumen. Embrace it in your workflow for a more seamless and productive programming experience.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.