Using AI to Help Finally Understand How to Use Rails Action Cable
Updated on June 26, 2024

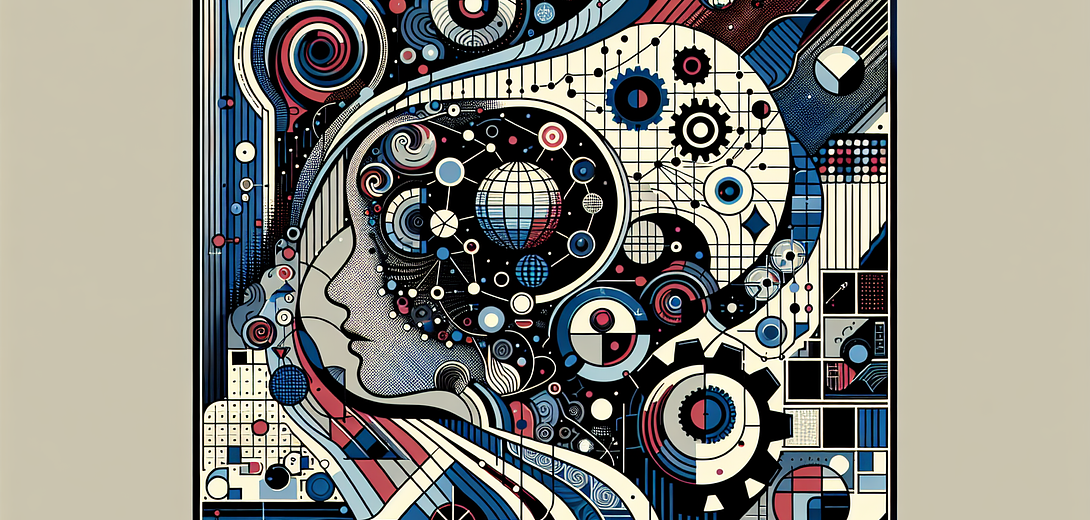
The ever-evolving landscape of web development continually presents us with new tools and libraries to master. One such tool is Rails Action Cable, which offers seamless WebSocket integration for your Ruby on Rails applications.
Understanding how to use Action Cable to add real-time features to your app can be daunting. Enter cloving—the integration of human creativity and intuition with the processing capabilities of AI, specifically GPT.
In this blog post, you’ll learn how to incorporate GPT into your workflow to understand and effectively use Rails Action Cable finally.
What is Cloving?
Cloving combines the best of human and machine intelligence to solve problems more efficiently. It’s about using AI tools not just for automation but for augmenting your skills and enhancing your workflow.
1. Getting Started with Basic Concepts
Before diving into implementation, it’s crucial to understand the foundational concepts of Action Cable. GPT can help clarify any confusion you might have.
Example:
If you’re unsure about the basic components of Action Cable, you can ask GPT:
Explain the basic components of Rails Action Cable and how they interact.
GPT will provide a detailed explanation, covering the key parts such as channels, connection objects, and subscription management in Action Cable, enabling you to grasp the fundamental concepts faster.
2. Setting Up Action Cable in Your Rails Project
Setting up Action Cable can involve multiple steps, which can be initially overwhelming. GPT can guide you through this process.
Example:
If you’re starting a new Rails project and need to set up Action Cable, prompt GPT:
Provide the steps to set up Action Cable in a new Rails application.
GPT will outline the necessary steps, including:
-
Add Action Cable configurations in your
cable.yml
:development: adapter: redis url: redis://localhost:6379/1 channel_prefix: your_app_development production: adapter: redis url: redis://localhost:6379/1 channel_prefix: your_app_production
-
Update your JavaScript to connect to Action Cable:
import consumer from "./cable"; const channel = consumer.subscriptions.create("RoomChannel", { connected() { console.log("Connected to the room channel"); }, received(data) { console.log("Received:", data); } });
-
Generate a new channel:
rails generate channel Room
-
Define actions in your channel:
class RoomChannel < ApplicationCable::Channel def subscribed stream_from "room_channel" end def unsubscribed # Any cleanup needed when channel is unsubscribed end end
3. Implementing Real-Time Features
Once you’ve set up Action Cable, you can start implementing real-time features. GPT can help by providing specific code examples and guidance.
Example:
Suppose you want to implement a real-time chat feature. You can prompt GPT:
How can I implement a real-time chat feature using Rails Action Cable?
GPT will provide you with a sample implementation:
-
Create a Chat Room Model:
rails generate model Room name:string rails db:migrate
-
Update the Room Channel to Broadcast Messages:
class RoomChannel < ApplicationCable::Channel def subscribed stream_from "room_#{params[:room]}" end def unsubscribed # Any cleanup needed when channel is unsubscribed end def speak(data) ActionCable.server.broadcast "room_#{params[:room]}", message: data['message'] end end
-
Update JavaScript to Handle Incoming Messages:
const roomChannel = consumer.subscriptions.create({ channel: "RoomChannel", room: "BestRoom" }, { connected() { console.log("Connected to the room channel"); }, received(data) { console.log("Received:", data.message); }, speak(message) { this.perform('speak', { message: message }); } }); document.querySelector('input[name="send"]').addEventListener('click', () => { const message = document.querySelector('textarea[name="message"]').value; roomChannel.speak(message); });
4. Debugging Common Issues
Real-time features can introduce new challenges and bugs. GPT can assist in troubleshooting and debugging these issues.
Example:
If you encounter a problem with your Action Cable connection, you can describe the issue to GPT:
My Action Cable connection is not working. I see a 'websocket connection failed' error. How can I fix this?
GPT will provide potential solutions, such as checking your Redis server status, ensuring your browser supports WebSockets, or verifying Action Cable configurations.
5. Generating Tests for Your Realtime Features
Testing real-time features can be tricky, but it’s essential for ensuring reliability. GPT can help you create test cases for your Action Cable implementation.
Example:
To generate RSpec tests for your Action Cable channels, ask GPT:
Generate RSpec tests for RoomChannel in Rails.
GPT will provide sample test cases:
```ruby
require 'rails_helper'
RSpec.describe RoomChannel, type: :channel do
it "subscribes to a stream" do
subscribe(room: 'BestRoom')
expect(subscription).to be_confirmed
expect(subscription).to have_stream_from("room_BestRoom")
end
it "broadcasts a message" do
subscribe(room: 'BestRoom')
ActionCable.server.broadcast "room_BestRoom", message: "Hello, World!"
expect(subscription).to have_broadcasted_to("room_BestRoom").with(message: "Hello, World!")
end
end
```
6. Documenting Your Action Cable Implementations
Proper documentation is vital for maintaining and sharing your work. GPT can assist you in writing clear and comprehensive documentation for your Action Cable implementations.
Example:
To document your RoomChannel
, you can prompt GPT:
Generate documentation for my RoomChannel in Rails.
GPT will create detailed documentation:
```markdown
# RoomChannel
The `RoomChannel` handles real-time communication for chat rooms. It streams messages from a specific room channel and broadcasts messages sent by users.
## Methods
- `subscribed`: Called when the consumer has successfully become a subscriber to the channel. Streams messages from the specified room.
- `unsubscribed`: Called when the consumer unsubscribes from the channel. Used for cleanup.
- `speak`: Broadcasts messages from users to the room channel.
## Usage
To subscribe to a room channel:
```javascript
const roomChannel = consumer.subscriptions.create({ channel: "RoomChannel", room: "BestRoom" }, {
connected() {
console.log("Connected to the room channel");
},
received(data) {
console.log("Received:", data.message);
},
speak(message) {
this.perform('speak', { message: message });
}
});
document.querySelector('input[name="send"]').addEventListener('click', () => {
const message = document.querySelector('textarea[name="message"]').value;
roomChannel.speak(message);
});
```
Conclusion
Understanding and implementing Rails Action Cable can be complex, but by embracing cloving and integrating AI tools like GPT into your workflow, you can simplify the learning process, streamline implementation, and enhance your overall productivity. Whether you’re setting up Action Cable, implementing real-time features, debugging, testing, or documenting, GPT serves as a powerful ally, amplifying your creativity and intuition in web development. Start leveraging this synergistic approach today and see how AI can transform your programming experience.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.