Unit Testing Vue Components with GPT
Updated on June 26, 2024

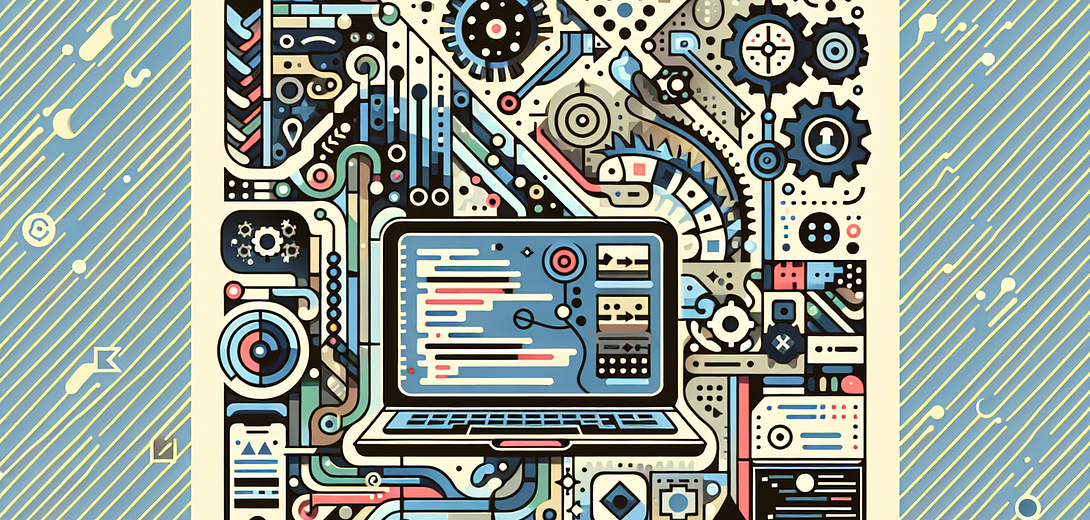
As front-end developers, we know how vital it is to maintain robust unit tests for our Vue components. But writing these tests can often be a meticulous and time-consuming task.
By adopting cloving—integrating human creativity and intuition with the analytical and processing capabilities of artificial intelligence (AI)—you can streamline your unit testing workflow and enhance your productivity.
In this blog post, we’ll explore how you can leverage GPT to assist in unit testing Vue components, helping you become more efficient and effective at your job.
Understanding Cloving
Cloving is a synergistic approach that combines the best of human and machine capabilities. By leveraging AI tools like GPT, we can push the boundaries of our programming efficiency and creativity, leading to smarter and faster development workflows.
1. Generating Test Cases Quickly
Writing test cases for Vue components can be repetitive and tedious. GPT can quickly generate meaningful test cases, allowing you to focus on more complex tasks.
Example:
Suppose you have a Vue component UserCard
that displays user information. To generate test cases for this component, you can prompt GPT:
Generate unit test cases for the following Vue component:
```vue
<template>
<div class="user-card">
<h2>{{ user.name }}</h2>
<p>{{ user.email }}</p>
</div>
</template>
<script>
export default {
name: 'UserCard',
props: {
user: {
type: Object,
required: true
}
}
};
</script>
<style scoped>
.user-card {
border: 1px solid #ccc;
padding: 1em;
border-radius: 5px;
}
</style>
GPT might generate the following test case using Jest and Vue Test Utils:
import { shallowMount } from '@vue/test-utils';
import UserCard from '@/components/UserCard.vue';
describe('UserCard.vue', () => {
it('renders user name and email', () => {
const user = { name: 'John Doe', email: '[email protected]' };
const wrapper = shallowMount(UserCard, {
propsData: { user }
});
expect(wrapper.find('h2').text()).toBe(user.name);
expect(wrapper.find('p').text()).toBe(user.email);
});
});
2. Refactoring Existing Test Cases
As our applications evolve, so do our test cases. GPT can help refactor existing test cases to improve readability, performance, or to adapt to new requirements.
Example:
If you need to refactor an old test case for the same UserCard
component, you can describe the changes needed to GPT:
Refactor this unit test case to check if a CSS class is applied when the user is active:
```javascript
import { shallowMount } from '@vue/test-utils';
import UserCard from '@/components/UserCard.vue';
describe('UserCard.vue', () => {
it('renders user name and email', () => {
const user = { name: 'John Doe', email: '[email protected]' };
const wrapper = shallowMount(UserCard, {
propsData: { user }
});
expect(wrapper.find('h2').text()).toBe(user.name);
expect(wrapper.find('p').text()).toBe(user.email);
});
});
GPT might produce a refactored test like this:
import { shallowMount } from '@vue/test-utils';
import UserCard from '@/components/UserCard.vue';
describe('UserCard.vue', () => {
it('renders user name and email', () => {
const user = { name: 'John Doe', email: '[email protected]', active: true };
const wrapper = shallowMount(UserCard, {
propsData: { user }
});
expect(wrapper.find('h2').text()).toBe(user.name);
expect(wrapper.find('p').text()).toBe(user.email);
expect(wrapper.classes()).toContain('active');
});
});
3. Providing Best Practices and Guidelines
AI can assist you in keeping up with best practices for writing tests, helping you avoid common pitfalls and adhere to industry standards.
Example:
You can ask GPT for best practices on testing Vue components:
What are the best practices for unit testing Vue components in 2024?
GPT may suggest best practices like:
- Isolate Components: Mock dependencies to focus on the component being tested.
- Use Shallow Mounting: To avoid rendering child components unnecessarily.
- Test Data Transitions: Ensure that component state updates correctly based on user interactions or props changes.
4. Debugging Test Failures
Debugging test failures can sometimes be more difficult than debugging the actual application code. GPT can help you decipher cryptic error messages and suggest potential fixes.
Example:
When you encounter a failing test case, you can describe the error to GPT:
I am getting the following error in my Vue unit test: `TypeError: Cannot read property 'text' of undefined`. Here's the test code:
```javascript
import { shallowMount } from '@vue/test-utils';
import UserCard from '@/components/UserCard.vue';
describe('UserCard.vue', () => {
it('renders user name and email', () => {
const user = { name: 'John Doe', email: '[email protected]' };
const wrapper = shallowMount(UserCard, {
propsData: { user }
});
expect(wrapper.find('h2').text()).toBe(user.name);
expect(wrapper.find('p').text()).toBe(user.email);
});
});
GPT might suggest:
The error indicates that the element you're trying to find doesn't exist in the wrapper. This can happen if the `user` prop is not properly passed to the component or if the structure of the template has changed. Ensure that the `UserCard` component's template has <h2> and <p> tags and that the `user` prop is correctly set:
import { shallowMount } from '@vue/test-utils';
import UserCard from '@/components/UserCard.vue';
describe('UserCard.vue', () => {
it('renders user name and email', () => {
const user = { name: 'John Doe', email: '[email protected]' };
const wrapper = shallowMount(UserCard, {
propsData: { user }
});
// Add checks to ensure elements are found before accessing their text content
const nameWrapper = wrapper.find('h2');
const emailWrapper = wrapper.find('p');
expect(nameWrapper.exists()).toBe(true);
expect(nameWrapper.text()).toBe(user.name);
expect(emailWrapper.exists()).toBe(true);
expect(emailWrapper.text()).toBe(user.email);
});
});
Conclusion
Unit testing Vue components with AI assistance perfectly embodies the essence of cloving—merging human expertise with the computational power of AI. By incorporating GPT into your testing workflow, you can improve productivity, write better test cases, and stay up-to-date with the latest best practices. Embrace the cloving approach and watch your unit testing process transform, providing more reliable and maintainable code for your applications.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.