Troubleshooting Memory Leaks in JavaScript with GPT's Assistance
Updated on April 14, 2025

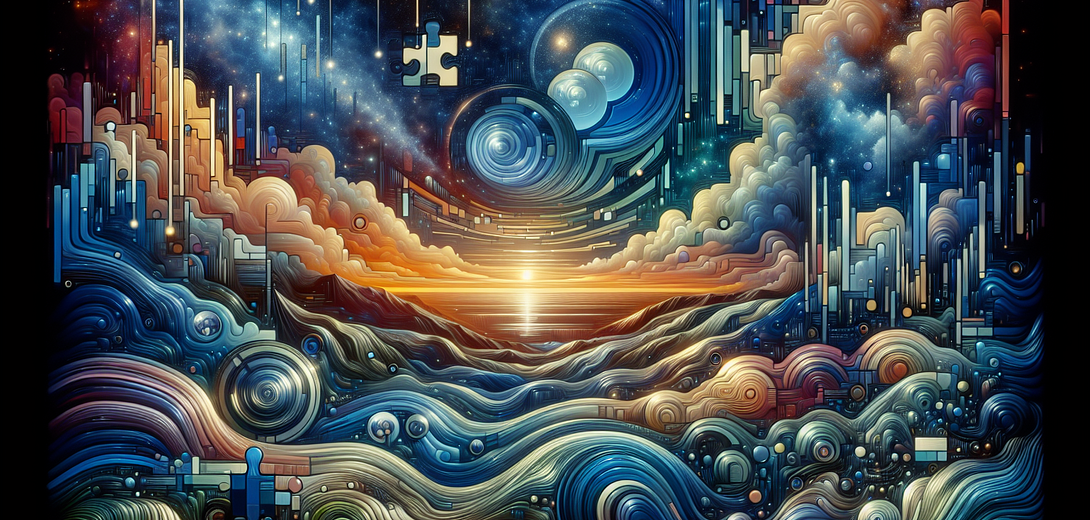
Dealing with memory leaks in JavaScript can be a daunting task, especially in complex applications. Memory leaks occur when your application holds onto memory that is no longer needed, causing the application to consume more memory over time. This can lead to performance degradation and even crashes. Fortunately, the Cloving CLI, with its AI-powered capabilities, can assist in identifying and addressing memory leaks efficiently. In this post, we’ll explore how to leverage Cloving’s functionality to troubleshoot memory leaks in JavaScript.
Understanding Memory Leaks
Before diving into using Cloving, it’s important to understand what memory leaks are and how they occur. Memory leaks happen when allocated memory is not released after it is no longer needed. In JavaScript, common causes include:
- Circular references within closures or the DOM.
- Unreferenced libraries or event listeners that are not removed.
- Unintentional global variables.
Getting Started with Cloving
First, you need to set up the Cloving CLI tool in your development environment. Follow these steps:
1. Installation
Install Cloving globally through npm:
npm install -g cloving@latest
2. Configuration
Once installed, configure Cloving with your API key and preferred models:
cloving config
Follow the prompts to enter your API key and select the AI model that best suits your needs.
3. Project Initialization
Initialize Cloving in the project directory where you want to troubleshoot memory leaks:
cloving init
This setup provides Cloving with context about your project, helping it generate more relevant and accurate insights.
Using Cloving to Troubleshoot Memory Leaks
Now, let’s see how Cloving can assist in identifying and resolving memory leaks in JavaScript applications.
4. Interactive Chat for Debugging Assistance
One of the most powerful features of Cloving is the chat
command, which allows you to interact live with the AI to get guidance and debugging help.
cloving chat -f path/to/your/javascript/file.js
This command opens an interactive session. You can describe the memory issue you’re facing:
cloving> I've noticed the application slows down over time, suspecting a memory leak. Can you help diagnose this?
The AI will provide targeted advice or point out potential areas in the code where memory leaks could occur.
5. Identifying Memory Leaks using Tokens Command
Another helpful command is the tokens
, which allows you to estimate the amount of memory-consuming code by analyzing the number of tokens:
cloving tokens -f src/app.js
This command provides insights on which parts of your code might have complex structures leading to potential leaks.
6. Analyzing Code for Best Practices
Use the generate review
feature to allow the AI to review your code for potential memory leak issues and suggest improvements:
cloving generate review -f path/to/your/javascript/file.js
Watch out for suggestions on how to manage references, handle global variables, and remove unnecessary event listeners:
Example output:
# Code Review: Detecting Memory Leaks
## Observations
1. **Large Objects and Arrays:** Identified unused properties in several objects.
2. **Event Listeners:** Detected event listeners not being removed, potentially causing memory leaks.
3. **Closure Scopes:** Noted closures capturing large-scope variables unnecessarily.
## Recommendations
- Regularly clean up unused objects and references.
- Employ WeakMap and WeakSet for managing non-essential objects.
- Ensure proper management of event listeners using `addEventListener` and `removeEventListener`.
7. Generating Code and Fix Proposals
Cloving can also generate code to help fix identified issues, such as revising event binding code to ensure proper removal of listeners:
cloving generate code --prompt "Refactor to prevent memory leaks by properly managing event listeners" --files src/app.js
Example code snippet:
// Existing Code
button.addEventListener('click', function() {
// Function code
}, false);
// Suggested Refactor
function handleClick() {
// Function code
}
button.addEventListener('click', handleClick, false);
button.removeEventListener('click', handleClick, false);
8. Continuous Learning with Cloving
Keep improving and refining your memory management skills with ongoing feedback from Cloving. Utilize the chat mode for complex questions, generate unit tests to validate memory management, and ask for advice on best practices regularly.
Conclusion
Using Cloving CLI as an AI-powered assistant significantly aids the process of diagnosing and fixing memory leaks in JavaScript applications. Not only does it provide immediate insights and fixes, but it also helps in learning best practices to prevent such issues from arising in the first place. Integrate Cloving into your workflow and transform your development experience by making memory leak troubleshooting more efficient and effective.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.