Supercharging Django App Development with AI-Driven Code Generation
Updated on April 01, 2025

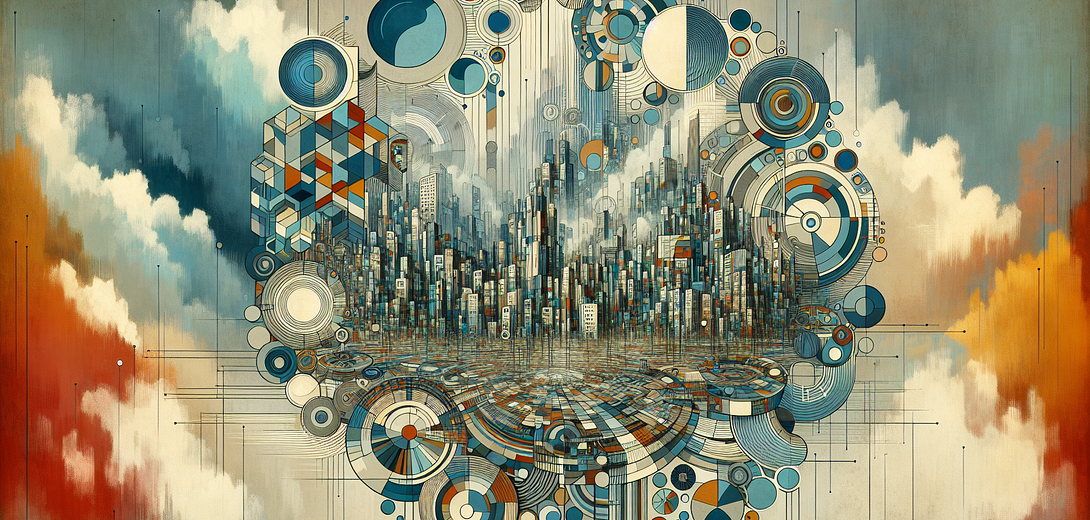
Django is a popular web framework for building scalable and secure web applications quickly. However, developing a Django application can be a repetitive and time-consuming process. To alleviate this, the Cloving CLI tool offers a unique AI-powered solution to supercharge your Django app development, enhancing productivity and code quality. In this tutorial, we will explore how Cloving can be integrated into your Django workflow to boost efficiency and streamline your development process.
Getting Started with Cloving
Before diving into Django-specific use cases, let’s ensure you have Cloving set up in your environment.
Step 1: Install Cloving
Install Cloving globally via npm:
npm install -g cloving@latest
Step 2: Configure Cloving
Set up Cloving with your API key and preferred AI model:
cloving config
Follow the interactive prompts to configure your preferences.
Step 3: Initialize Your Django Project
To get started with Cloving in your Django project directory, initialize it by running:
cloving init
This will create a cloving.json
file containing metadata about your application.
Utilizing AI-Driven Code Generation with Cloving
Cloving provides several features that can significantly enhance your Django app development workflow.
Generating Django Models
Suppose you need to create models for an ecommerce application. You can use Cloving to generate models in your Django project:
cloving generate code --prompt "Create Django models for Product, Category, and Order" --files ecommerce/models.py
Here’s a sample output of what Cloving might generate:
from django.db import models
class Category(models.Model):
name = models.CharField(max_length=255)
created_at = models.DateTimeField(auto_now_add=True)
def __str__(self):
return self.name
class Product(models.Model):
name = models.CharField(max_length=255)
price = models.DecimalField(max_digits=10, decimal_places=2)
category = models.ForeignKey(Category, on_delete=models.CASCADE)
created_at = models.DateTimeField(auto_now_add=True)
def __str__(self):
return self.name
class Order(models.Model):
product = models.ForeignKey(Product, on_delete=models.CASCADE)
quantity = models.PositiveIntegerField()
created_at = models.DateTimeField(auto_now_add=True)
def __str__(self):
return f"Order #{self.id} for {self.product.name}"
Generating Views and URL Patterns
To create basic views and URL patterns, you can use Cloving’s code generation capabilities. For example:
cloving generate code --prompt "Create views and URL patterns for the ecommerce app" --files ecommerce/views.py ecommerce/urls.py
Adding Unit Tests
Cloving can also generate unit tests for your models to ensure robustness and code quality. Simply run:
cloving generate unit-tests -f ecommerce/models.py
This will create corresponding test files with predefined tests:
from django.test import TestCase
from ecommerce.models import Category, Product, Order
class CategoryModelTest(TestCase):
def test_string_representation(self):
category = Category(name="Electronics")
self.assertEqual(str(category), category.name)
class ProductModelTest(TestCase):
def test_string_representation(self):
product = Product(name="Laptop", price=1599.99)
self.assertEqual(str(product), product.name)
class OrderModelTest(TestCase):
def test_string_representation(self):
category = Category.objects.create(name="Electronics")
product = Product.objects.create(name="Laptop", price=1599.99, category=category)
order = Order.objects.create(product=product, quantity=2)
self.assertEqual(str(order), f"Order #{order.id} for {product.name}")
Engaging in Interactive Development with Cloving Chat
For complex tasks or when you require ongoing AI assistance, Cloving Chat is invaluable. Start an interactive session:
cloving chat -f ecommerce/models.py
In the chat session, you can ask Cloving to:
- Refactor code
- Add comments
- Provide explanations
- Optimize performance
Smart Commit Messages
Cloving can help craft meaningful commit messages, ensuring better commit history documentation. Use:
cloving commit
After analyzing changes, Cloving may generate a descriptive commit message like:
Add models and initial tests for ecommerce app
Conclusion
Integrating Cloving into your Django development workflow provides a significant boost to productivity and code quality. By automating repetitive tasks and leveraging AI-powered insights, you can focus on higher-level design and decision-making, reducing errors and saving time.
Remember, the true power of Cloving lies in augmenting your existing skills, not replacing them. Experiment with Cloving in your projects and see the transformation in your development process first-hand! Embrace this AI-powered tool and explore the future of AI-driven app development.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.