Supercharge Your WebAssembly Projects with Code Generation by GPT
Updated on March 05, 2025

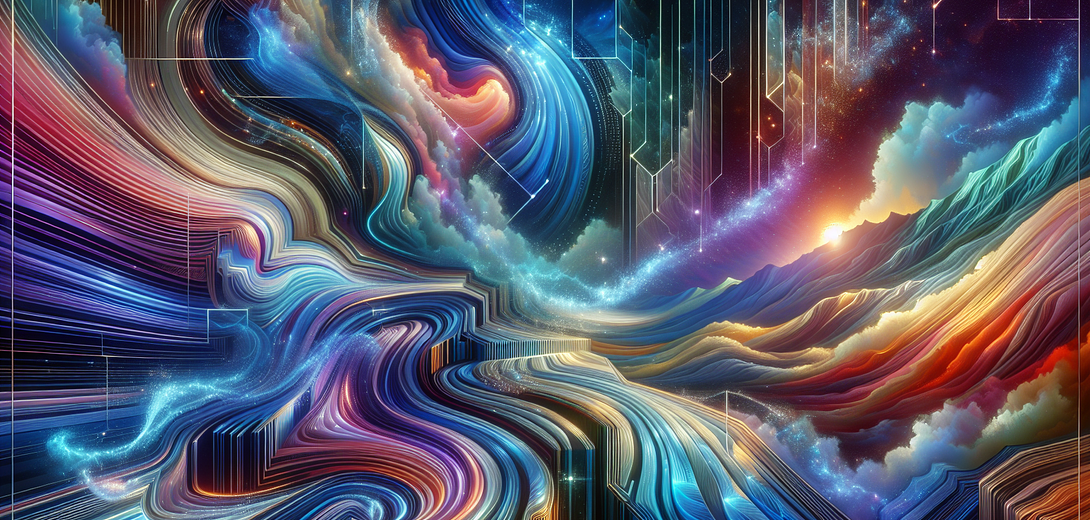
Supercharging WebAssembly Projects with Cloving CLI
WebAssembly (Wasm) enables high-performance, low-level code written in languages like Rust, C/C++, and more to run in the browser. While Wasm opens up a world of possibilities for web development, generating, testing, and integrating this code can be time-consuming. Cloving CLI, an AI-powered command-line tool, streamlines these tasks by automating code generation, testing stubs, and providing interactive development assistance. In this post, we’ll guide you through using Cloving with a WebAssembly project, showcasing how AI can take your workflow to the next level.
1. Introduction to Cloving CLI
Cloving CLI is a command-line interface that uses GPT-based models to help you:
- Generate new code or refactor existing code in various languages
- Review and test your code for best practices
- Interact via a chat-based environment for iterative improvements
- Craft commit messages automatically for clarity in version control
By analyzing your project’s context, Cloving tailors its suggestions to your codebase, reducing the time you spend on boilerplate or repetitive tasks.
2. Setting Up Cloving
2.1 Installation
Install Cloving globally with npm:
npm install -g cloving@latest
2.2 Configuration
Configure Cloving by specifying your API key and AI model (e.g., GPT-3.5, GPT-4):
cloving config
Follow the prompts to finalize your environment. Once set up, Cloving will connect to the AI backend to generate code and run advanced tasks for you.
3. Initializing Your WebAssembly Project
3.1 Project Setup
To allow Cloving to understand your codebase structure, run:
cloving init
Cloving analyzes the files and creates a cloving.json
containing metadata about your WebAssembly project. This context helps the AI produce relevant code, from Rust or C/C++ modules to TypeScript bindings or other Wasm-related utilities.
3.2 Typical WebAssembly Workflow
While the specifics can vary (e.g., using wasm-pack for Rust, emscripten for C/C++), most Wasm projects include:
- Source code in Rust/C/C++
- Build scripts or config (e.g., Cargo.toml for Rust)
- JavaScript or TypeScript glue code to load and interact with the .wasm binary
Cloving can assist in generating or refining each piece of this puzzle.
4. Leveraging Cloving’s Powerful Code Generation
4.1 Example: Rust-based WebAssembly
Let’s say you want a Rust function computing the Fibonacci sequence, which you then compile to Wasm. Prompt Cloving:
cloving generate code --prompt "Implement a WebAssembly-compatible Rust function for calculating Fibonacci numbers" --files src/lib.rs
Sample Output:
// src/lib.rs
#[no_mangle]
pub extern "C" fn fibonacci(n: u32) -> u32 {
match n {
0 => 0,
1 => 1,
_ => fibonacci(n - 1) + fibonacci(n - 2),
}
}
Notes:
- Cloving references standard patterns, such as applying
#[no_mangle]
and anextern "C"
function signature for compatibility with WebAssembly exports. - You can add more context to your prompt if you need advanced features (e.g., iterative approach, memoization, etc.).
4.2 Enhancing and Saving Generated Code
Refine or optimize the generated code by specifying additional details:
cloving generate code --prompt "Improve the Fibonacci function with memoization for better performance" --files src/lib.rs --interactive
Cloving might produce an optimized version that uses a global or static cache. You can finalize and save the changes or iterate more in interactive mode.
5. Generate Contextual Unit Tests
Once you have your Rust code, you need tests to ensure correctness, especially for performance-critical code. Run:
cloving generate unit-tests -f src/lib.rs
Cloving will produce a test module verifying the fibonacci
function:
// src/lib.rs
#[cfg(test)]
mod tests {
use super::*;
#[test]
fn test_fibonacci() {
assert_eq!(fibonacci(0), 0);
assert_eq!(fibonacci(1), 1);
assert_eq!(fibonacci(10), 55);
}
}
Notes:
- If your code uses advanced features (e.g., parallel computations), mention them so Cloving can generate more suitable tests.
- Expand coverage to negative or edge cases as needed.
6. Using Cloving Chat for Complex Tasks
6.1 Interactive Chat Sessions
For advanced or iterative tasks (like bridging JavaScript with Rust-based Wasm or debugging build scripts), Cloving chat is invaluable. Open a session:
cloving chat -f src/lib.rs
Here you can:
- Ask for help hooking up the compiled
.wasm
in a TypeScript or Node.js environment. - Request code adjustments or deeper explanations of the AI’s suggestions.
- Refine performance aspects or memory usage in the function.
Example:
cloving> Could you provide JavaScript glue code to load this Fibonacci function from the compiled .wasm?
Cloving may respond with a snippet that uses the WebAssembly.instantiate
API or higher-level tools like wasm-bindgen
.
7. Streamlining Commits
Good commit messages are essential for teamwork and version control. Let Cloving generate them:
cloving commit
Cloving analyzes changes (like new Rust code or test files) and proposes a commit message, for instance:
Add Rust-based Fibonacci function and associated tests for WebAssembly
You can accept or refine as needed.
8. Additional Examples and Use Cases
8.1 C++ WebAssembly
If you’re building .wasm
from C++ with Emscripten, you could prompt:
cloving generate code --prompt "Implement a WebAssembly-compatible C++ function to multiply matrices" --files src/matrix.cpp
Cloving might generate a function with an extern "C"
signature, taking pointers to arrays. Tests could be in a separate .test.cpp
or use Emscripten’s test harness.
8.2 JavaScript or TypeScript Bindings
You could also have Cloving produce TypeScript glue code to import your .wasm
module:
cloving generate code --prompt "Create TypeScript code to load and call the Rust fibonacci wasm function" --files wasm/fib.ts
Sample Output:
// wasm/fib.ts
export async function loadFibWasm(): Promise<(n: number) => number> {
const response = await fetch('fibonacci.wasm');
const bytes = await response.arrayBuffer();
const result = await WebAssembly.instantiate(bytes);
const { fibonacci } = result.instance.exports as { fibonacci: (n: number) => number };
return fibonacci;
}
9. Best Practices for Cloving + WebAssembly
- Initialize: Always run
cloving init
in your project root so the AI can gather context on your modules, tools, or config. - Detailed Prompts: If you have a complex build or specialized usage (e.g., “Use cargo features X, Y for release build”), mention them in your prompt.
- Iterate: Start with a base function, ask Cloving to optimize or expand, then refine code with
cloving chat
for advanced scenarios. - Testing: Combine Cloving’s test generation with a standard test framework (like Rust’s built-in tests or Emscripten’s for C++). Expand coverage beyond the initial AI suggestion.
- Performance: If performance is critical, specify it. E.g., “Optimize for large input arrays” or “Use SSE instructions if possible.” Cloving can produce code or references for advanced optimizations, though you must verify correctness.
10. Example Workflow
Here’s a typical approach to integrating Cloving in a Wasm project:
- Initialize:
cloving init
to gather codebase context. - Generate: e.g.,
cloving generate code --prompt "Write a Rust function to do prime checking for Wasm" -f src/lib.rs
. - Test:
cloving generate unit-tests -f src/lib.rs
. - Iterate: Use
cloving chat -f src/lib.rs
to add optimizations or handle edge cases. - Commit: Summarize changes with
cloving commit
. - Compile: Use your typical toolchain (e.g.
wasm-pack build
orcargo build --target wasm32-unknown-unknown
) to produce the.wasm
.
11. Conclusion
By integrating Cloving CLI into your WebAssembly development, you drastically reduce boilerplate, accelerate code generation, and keep tests aligned with your transformations or expansions. Whether you’re using Rust, C++, or other languages, Cloving can scaffold, refine, and test your Wasm-compatible code, letting you focus on innovation rather than repetitive tasks.
Remember: Cloving is here to augment your expertise. Always review AI-generated code for domain-specific correctness, performance constraints, and style consistency. With Cloving, you can easily harness the full potential of WebAssembly, building next-level performance features for the web faster and more confidently.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.