Streamlining TypeScript Code Generation with GPT
Updated on December 25, 2024

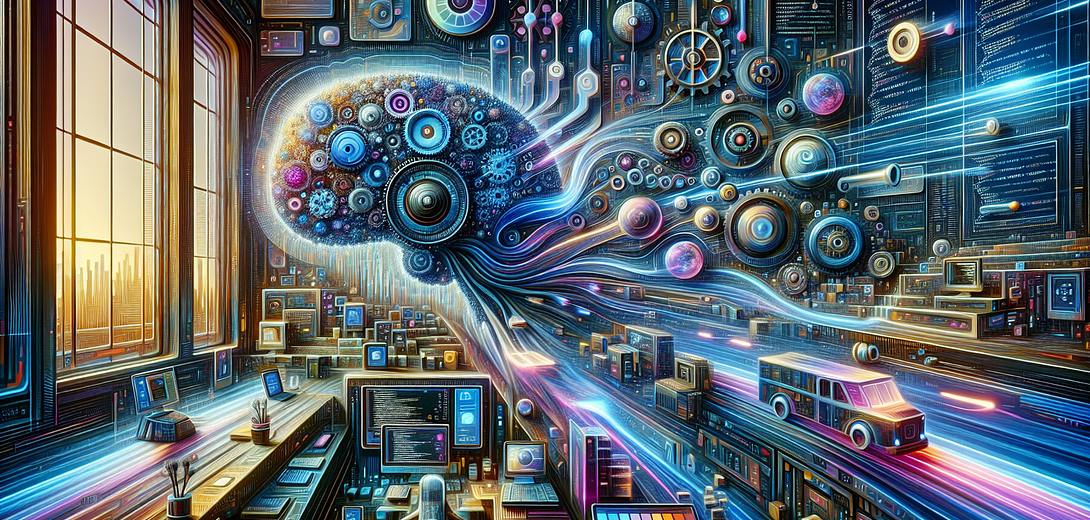
In the evolving landscape of software development, TypeScript has emerged as a popular choice for its enhanced readability and robust type system. Integrating AI in your development workflow can catapult your productivity to new heights, which is precisely what the Cloving CLI tool offers. By leveraging the power of AI, the Cloving CLI streamlines TypeScript code generation, making your workflow more efficient and effective. In this blog post, we will guide you through the various ways you can master TypeScript code generation using the Cloving CLI.
What is Cloving CLI?
Cloving is a command-line tool designed to integrate AI capabilities into your software development processes. Whether you need to generate new code snippets, unit tests, or even meaningful git commit messages, Cloving has got you covered. Let’s dive into how to use this tool effectively for TypeScript projects.
1. Setting Up Cloving
Before we start generating TypeScript code, let’s make sure Cloving is correctly set up.
Installation:
Start by installing Cloving via npm:
npm install -g cloving@latest
Configuration:
To configure Cloving with your API key and preferred models, run:
cloving config
This command will guide you through setting up your API key, selecting models, and other preferences.
2. Initializing Your TypeScript Project
Initializing Cloving within your project directory is crucial as it helps the AI understand your project context.
cloving init
This introduces a cloving.json
file, storing metadata about your application.
3. Generating TypeScript Code
With Cloving, generating TypeScript code becomes a breeze. Suppose you need to create a utility function in TypeScript.
Example:
To generate a function for deep cloning objects, execute:
cloving generate code --prompt "Create a TypeScript function for deep cloning objects" --files src/utils/clone.ts
This command will examine your project context and generate a suitable TypeScript function:
// src/utils/clone.ts
export function deepClone<T>(obj: T): T {
return JSON.parse(JSON.stringify(obj));
}
4. Interactive Code Revision
After generating code, Cloving offers an interactive mode for refining your results. Suppose the initial output needs adjustments; you can interactively revise it using:
cloving generate code --interactive --prompt "Enhance the deepClone function to handle functions and symbolic keys"
Here’s how GPT might revise the deepClone
function to accommodate functions and symbolic keys:
// src/utils/clone.ts
export function deepClone<T>(obj: T): T {
const seen = new WeakMap();
function recursiveClone(item: any): any {
if (item === null || typeof item !== 'object') {
if (typeof item === 'function') {
return item.bind(null); // Clone functions by returning a bound copy
}
return item;
}
if (seen.has(item)) {
return seen.get(item);
}
const result = Array.isArray(item) ? [] : {};
seen.set(item, result);
Reflect.ownKeys(item).forEach(key => {
(result as any)[key] = recursiveClone((item as any)[key]);
});
return result;
}
return recursiveClone(obj);
}
5. Generating Unit Tests for TypeScript Files
Ensure the reliability of your TypeScript code by generating unit tests using Cloving:
cloving generate unit-tests -f src/utils/clone.ts
The output will be a tailored set of unit tests:
// src/utils/clone.test.ts
import { deepClone } from './clone';
describe('deepClone', () => {
it('clones an object deeply', () => {
const original = { a: { b: 2 } };
const cloned = deepClone(original);
expect(cloned).toEqual(original);
expect(cloned).not.toBe(original);
});
it('handles functions correctly', () => {
const func = () => 42;
const clonedFunc = deepClone({ func }).func;
expect(clonedFunc()).toBe(42);
});
it('handles symbol keys', () => {
const sym = Symbol('key');
const original = { [sym]: 'value' };
const cloned = deepClone(original);
expect(cloned[sym]).toBe('value');
});
});
6. Utilizing Cloving Chat for Ongoing Assistance
For more complex project requirements or continuous help, leverage Cloving’s chat feature:
cloving chat -f src/utils/clone.ts
This initiates a chat environment where you can ask for additional features, explanations, or even advice on best practices.
7. Enhancing Your Git Workflow
Cloving also aids in describing your code changes. Generate meaningful commit messages with:
cloving commit
This command provides insightful commit messages based on your project context and changes made.
Conclusion
By incorporating Cloving CLI into your TypeScript development workflow, you harness the potential of AI to accelerate code generation, ensure quality through precise unit tests, and manage projects more efficiently. As you embrace Cloving, you augment your programming skills, making every step of your coding journey more productive and insightful.
Remember, Cloving is there to support you. Use it to bolster your development process, letting AI work as your efficient pair-programmer. Happy coding with TypeScript and Cloving CLI!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.