Streamlining React App State Management with GPT and Recoil
Updated on April 17, 2025

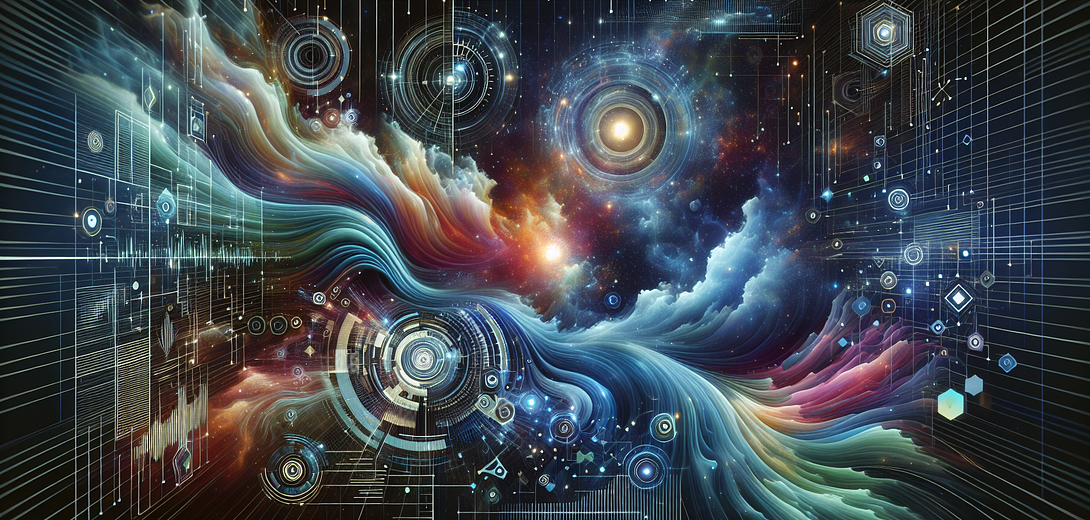
State management is a crucial part of building scalable and maintainable React applications. Traditional approaches can sometimes be cumbersome or inefficient, especially as applications grow in complexity. However, by leveraging the power of AI through the Cloving CLI tool, combined with Recoil’s flexible state management system, developers can enhance their productivity and code quality significantly. In this blog post, we’ll explore how to streamline React app state management using GPT-powered Cloving CLI and Recoil.
1. Getting Started with Cloving and Recoil
First, let’s set up our development environment to utilize Cloving and Recoil.
Installation:
Install Cloving globally using npm:
npm install -g cloving@latest
Then, add Recoil to your React project:
npm install recoil
Configuration:
Configure Cloving to use your preferred AI model:
cloving config
Follow the interactive prompts to set up your AI model, API key, and preferences.
2. Initializing Your React Project with Cloving
To allow Cloving to understand your project context, initialize it within your project directory:
cloving init
This command will create a cloving.json
file containing metadata about your application, enabling Cloving to provide more relevant code suggestions.
3. Generating Recoil State Management Code with Cloving
One of the distinct features of Cloving is its ability to generate code based on project context and explicit prompts.
Example:
Let’s say we want to manage the state for a list of tasks in our React application using Recoil. We can use Cloving to generate the necessary code:
cloving generate code --prompt "Create a Recoil state atom for managing a list of tasks in a React application" --files src/state/atoms.js
Cloving will produce a relevant code snippet:
// src/state/atoms.js
import { atom } from 'recoil';
export const taskListState = atom({
key: 'taskListState',
default: [],
});
This atom will hold our task list, and we can now use it throughout our application by utilizing Recoil hooks.
4. Integrating Generated Code into Your React Components
After generating the state management code, the next step is integrating it into your React components.
Using the Recoil State:
Create a React component that interacts with the taskListState
atom.
cloving generate code --prompt "Create a React component to display and manage tasks using the taskListState atom" --files src/components/TaskList.jsx
Resulting in the following component:
// src/components/TaskList.jsx
import React from 'react';
import { useRecoilState } from 'recoil';
import { taskListState } from '../state/atoms';
const TaskList = () => {
const [tasks, setTasks] = useRecoilState(taskListState);
const addTask = (task) => {
setTasks([...tasks, task]);
};
return (
<div>
<h1>Task List</h1>
<ul>
{tasks.map((task, index) => (
<li key={index}>{task}</li>
))}
</ul>
{/* Add input and button to add tasks */}
...
</div>
);
};
export default TaskList;
In this snippet, useRecoilState
connects our component to the taskListState
atom, facilitating effective state management.
5. Reviewing and Refining Your Code with Cloving
Once your initial implementation is done, you can use Cloving to perform code reviews or generate unit tests, ensuring your code’s reliability and quality.
Code Review:
Invoke Cloving for an AI-guided code review:
cloving generate review --files src/components/TaskList.jsx
The code review will highlight improvements and suggest optimizations, streamlining the refinement process.
Unit Tests:
Generate unit tests for your component to test functionality:
cloving generate unit-tests -f src/components/TaskList.jsx
This command will create tailored unit tests to ensure that your task management logic is robust and bug-free.
Conclusion
By incorporating Cloving CLI’s GPT capabilities with Recoil’s efficient state management, you can vastly improve your workflow and code quality in a React application. This synergy not only streamlines the state management process but also empowers developers with intelligent code generation, reviews, and testing. Embrace Cloving and Recoil to experience a smarter approach to React development, making your applications more dynamic, maintainable, and easier to scale.
Enhance your development process by integrating AI with state management, and witness unprecedented productivity in your coding endeavors!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.