Streamlining Java Backend Development with GPT
Updated on November 01, 2024

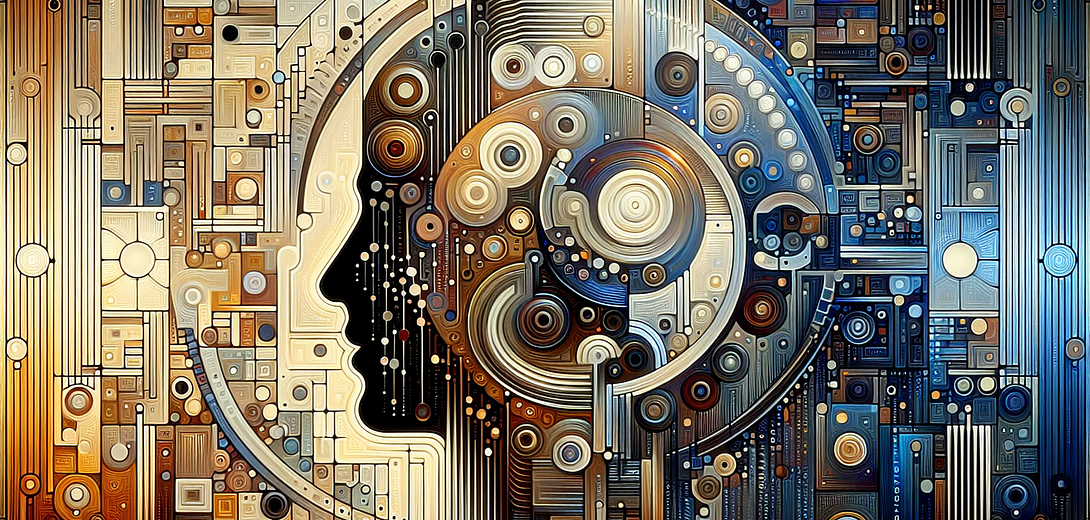
Java backend development can often become complex and time-consuming. However, with the Cloving CLI, an AI-powered command-line interface, you can enhance your productivity and code quality. In this post, we’ll explore how to use Cloving CLI to streamline Java backend development, making your workflow more efficient and effective.
Setting Up Cloving
Installation
The first step is to install the Cloving CLI. It can be easily installed globally using npm:
npm install -g cloving@latest
Configuration
Once installed, configure Cloving with your preferred AI model and API key:
cloving config
Follow the interactive prompts to input your API key and choose the models you wish to use for your projects.
Initializing Your Java Project
To start using Cloving for your Java project, initialize it in your project directory with:
cloving init
This command analyzes your project structure and creates a cloving.json
file, storing metadata about your application and default context settings.
Code Generation with Cloving
Generating Java Classes
Imagine you need a basic REST API endpoint for a book management system. With Cloving, you can generate a Java class using:
cloving generate code --prompt "Create a REST API endpoint for adding a book in a Spring Boot application" --files src/main/java/com/example/library/BookController.java
This command will produce a .java
file resembling:
// src/main/java/com/example/library/BookController.java
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/api/books")
public class BookController {
@PostMapping("/add")
public ResponseEntity<Book> addBook(@RequestBody Book book) {
// Logic to save the book
return ResponseEntity.ok(book);
}
}
Revising Generated Code
After generating the code, use Cloving’s interactive prompt to revise or enhance it further:
cloving> Add a service layer to handle book persistence logic
Cloving will incorporate a service layer while maintaining the context of your application.
Generating Unit Tests
Quality assurance is essential, and unit tests are a vital part of software development. Cloving helps generate unit tests easily:
cloving generate unit-tests -f src/main/java/com/example/library/BookService.java
The command creates a test file, and an example output might be:
// src/test/java/com/example/library/BookServiceTest.java
import static org.junit.jupiter.api.Assertions.*;
import com.example.library.service.BookService;
import org.junit.jupiter.api.Test;
class BookServiceTest {
@Test
void testAddBook() {
BookService bookService = new BookService();
Book book = new Book("1984", "George Orwell");
assertTrue(bookService.addBook(book));
}
}
Interacting with Cloving Chat
For more complex tasks or when you need an AI pair programmer, utilize Cloving chat:
cloving chat -f src/main/java/com/example/library/BookController.java
This opens an interactive session where you can:
- Ask questions about your Java code.
- Get explanations and suggestions.
- Use special commands like
save
,commit
, andreview
.
Example of Interactive Chat Session
🍀 🍀 🍀 Welcome to Cloving REPL 🍀 🍀 🍀
What would you like to do?
cloving> Refactor the BookController to handle errors gracefully
Certainly! Here's a refactored version with error handling:
...
Enhancing Productivity with Cloving
Generating Commit Messages
Cloving can help you craft meaningful commit messages for your projects:
cloving commit
This command generates a descriptive commit message based on your recent code changes.
Using Proxies and Estimating Tokens
To optimize large-team workflows or handle specific deployment environments, use Cloving’s proxy server feature:
cloving proxy
Estimate the number of tokens for billing or performance metrics:
cloving tokens -f src/main/java/com/example/library/*
Conclusion
The Cloving CLI tool brings the power of AI to your Java backend development, enabling you to work more efficiently and produce high-quality code. By seamlessly integrating AI into your workflow, you can automate mundane tasks, improve code quality, and focus on building innovative solutions.
Remember, Cloving is here to assist, not replace, your coding capabilities. Use it wisely to boost productivity and enhance your development process.
Explore Cloving CLI today and transform your Java backend development experience!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.