Streamlining Enterprise-Level Java Codebases with AI-Powered Refactoring
Updated on April 01, 2025

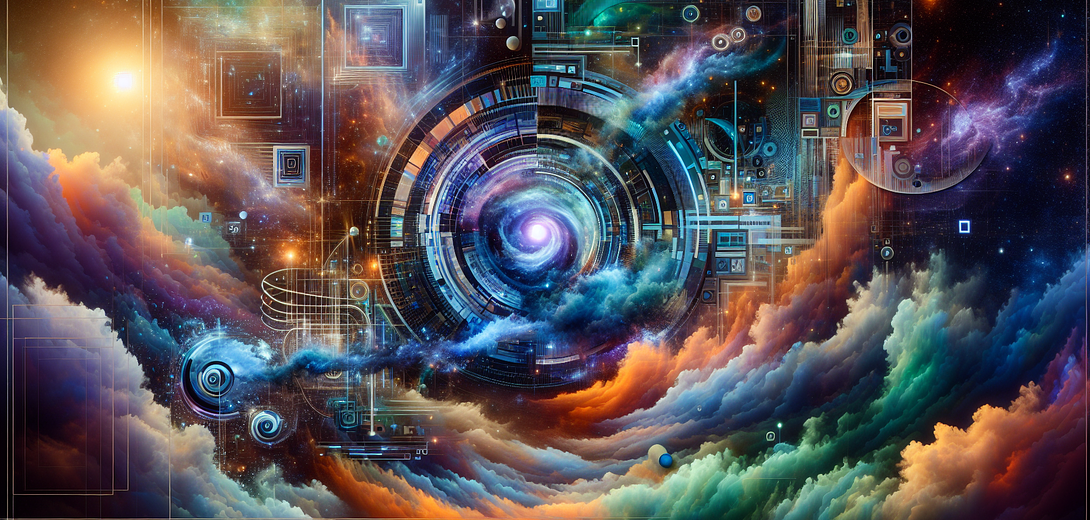
Revolutionizing Enterprise-Level Java Refactoring with Cloving CLI
Enterprise-level Java applications often evolve over years or even decades, accumulating large, complex codebases. Maintaining, refactoring, and modernizing such systems can be daunting, especially when working to preserve critical business logic and domain intricacies. Cloving CLI steps in as an AI-powered command-line interface that helps developers automate and refine the refactoring process. By integrating Cloving, you can quickly restructure old servlets, monolithic EJB-based code, or massive Spring Boot apps for better maintainability and performance.
In this blog post, we’ll explore how Cloving CLI can drastically simplify the Java refactoring journey, offering code generation, code review, and interactive AI-driven improvements suited to even the most sprawling enterprise codebases.
1. Getting Started with Cloving CLI
1.1 Cloving at a Glance
Cloving CLI uses GPT-based models to:
- Generate new Java code or suggest refactorings
- Review your existing code for potential improvements
- Refine your code iteratively via an interactive chat
- Scaffold or expand test coverage
- Draft commit messages reflecting the essence of your changes
By capturing your project’s structure, Cloving tailors its outputs to your established architecture and coding style.
1.2 Installation and Configuration
Install Cloving globally via npm:
npm install -g cloving@latest
Configure Cloving with your API key and AI model (e.g., GPT-3.5, GPT-4):
cloving config
Follow the prompts to finalize your environment and ensure Cloving can link to the AI backend.
1.3 Project Initialization
Inside the root folder of your Java project (e.g., containing pom.xml
, build.gradle
, or your main code directories), run:
cloving init
Cloving creates a cloving.json
, analyzing your file structure, dependencies, and potentially scanning for frameworks like Spring or Jakarta EE. This context ensures more accurate, relevant code generation and refactoring suggestions.
2. AI-Powered Refactoring in Enterprise Java
Refactoring large, legacy Java codebases can be complex, involving code spread across multiple layers (controllers, services, repositories, domain logic) and possibly older frameworks (e.g., EJB, Struts) or archaic design patterns. Cloving offers a structured approach to addressing these challenges by automatically rewriting or restructuring code where needed, guided by your prompts.
2.1 Example: Modernizing a Legacy Servlet
Suppose you have a servlet from the days before Spring adoption, and you’d like to modernize it by moving to a Spring-friendly style or adopting best practices (like separation of concerns, usage of DTOs, or improved error handling). Prompt Cloving:
cloving generate code --prompt "Refactor this Java servlet to follow modern Spring-based design patterns" --files src/main/java/com/example/LegacyServlet.java
Sample Output:
package com.example.controllers;
import org.springframework.web.bind.annotation.*;
import org.springframework.stereotype.Controller;
import org.springframework.http.ResponseEntity;
import com.example.services.LegacyService;
@Controller
@RequestMapping("/legacy")
public class ModernLegacyController {
private final LegacyService legacyService;
public ModernLegacyController(LegacyService legacyService) {
this.legacyService = legacyService;
}
@GetMapping
public ResponseEntity<String> getLegacyData() {
String data = legacyService.fetchData();
return ResponseEntity.ok(data);
}
// Additional refactored endpoints...
}
Key improvements:
- Controller annotation instead of raw servlets
- Spring frameworks for DI and request mapping
- Service usage for business logic, ensuring decoupling
2.2 Handling EJB-based Code
If your legacy app uses EJB or JPA sessions in older style, mention that in your prompt:
cloving generate code --prompt "Refactor an EJB-based user session bean into a modern Spring service" --files src/main/java/com/example/EJBUserSession.java
Cloving might produce a UserService
class with @Service
annotation, converting your EJB methods to Spring-based injection and JPA/Hibernate usage.
3. Advanced Examples and Best Practices
3.1 Extracting Common Utilities to Libraries
Large enterprise apps often have repeated logic for string manipulation, date handling, or security checks. Ask Cloving to identify or extract them:
cloving generate code --prompt "Extract repeated date parsing logic into a reusable utility class" --files src/main/java/com/example/utils/DateUtils.java
Sample Output:
package com.example.utils;
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.time.format.DateTimeParseException;
public class DateUtils {
public static LocalDate parseDate(String dateStr, String pattern) {
try {
DateTimeFormatter formatter = DateTimeFormatter.ofPattern(pattern);
return LocalDate.parse(dateStr, formatter);
} catch (DateTimeParseException e) {
// handle or rethrow
return null;
}
}
}
By generating this utility, you unify date handling in a single location, improving maintainability and consistency.
3.2 Converting Monoliths to Microservices
Some enterprises move from a large monolith to multiple microservices. While Cloving won’t single-handedly design your microservices, it can refactor modules or classes to reflect microservice-friendly patterns:
cloving generate code --prompt "Refactor the Payment module to a standalone Spring Boot microservice" --files PaymentModule.java
Cloving might create a new PaymentApplication
class with a separate pom.xml
or build.gradle
snippet for a dedicated microservice, extracting PaymentService
logic into a service
package and setting up a new controller
.
4. Interactive Code Revision
Cloving offers an interactive chat for iterative refactoring, crucial when dealing with large code changes or complex domain logic:
cloving chat -f src/main/java/com/example/LegacyApp.java
Within this environment, you can:
- Ask for recommended design patterns, concurrency improvements, or bridging legacy frameworks to modern ones
- Iterate on specialized error handling or logging frameworks
- Discuss potential performance bottlenecks
Example:
cloving> Separate data access logic from business logic in LegacyApp by introducing a DAO layer
Cloving might propose moving queries or raw JDBC calls into a distinct LegacyDAO
class and adjusting the LegacyService
.
5. AI-Powered Code Review
5.1 Running a Code Review
After a major refactor or series of changes, you can let Cloving review your code:
cloving generate review --files src/main/java/com/example/RefactoredApp.java
Cloving scans for potential issues, from missing error handling to style inconsistencies, providing an overview:
Potential improvements:
- Avoid repeated try-catch blocks by extracting a utility
- Consider final for constants
- Evaluate concurrency logic in data retrieval
While not a replacement for thorough manual review, it highlights potential areas for further improvements.
6. Checking in Code with AI-Suggested Messages
Cloving can also generate commit messages. After staging your refactored or newly created files:
cloving commit
Cloving reviews changes, suggesting a commit message like: “Refactor EJB-based user session to modern Spring service, and add utility for date parsing.” You can accept or edit it prior to committing.
7. Additional Best Practices
- Initialize Each Codebase
If you maintain multiple large codebases, runcloving init
in each to ensure context-specific code generation. - Iterate in Chat
For complex refactoring tasks, break them down and refine iteratively. Overly large single prompts might yield less precise results. - Review Thoroughly
Cloving’s suggestions are powerful but not infallible. Always confirm code correctness, domain alignment, and performance constraints. - Expand Testing
The initial test scaffolding from Cloving is helpful – add negative cases, concurrency tests, or advanced domain logic coverage to ensure robust reliability. - Keep Security in Mind
Especially for enterprise-level apps dealing with sensitive data, ensure that generated code respects security patterns and uses environment variables or secure parameter management.
8. Conclusion
Maintaining and refactoring enterprise-level Java codebases often requires significant planning and time. With Cloving CLI, you can shorten this refactoring cycle, employing AI-driven code generation to reorganize legacy servlets, transform EJB-based modules, or modularize monolithic structures. Cloving’s interactive chat helps with deeper or more specialized transformations, while its code review capabilities catch missed improvements.
Remember, while Cloving’s GPT-based approach automates repetitive tasks, your domain expertise remains crucial. Always verify correctness, performance, and security. By merging your knowledge with Cloving’s AI-driven solutions, you can transform your large Java codebase into a cleaner, more maintainable, and modernized system.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.