Streamlining C++ Application Development with AI and GPT
Updated on March 30, 2025

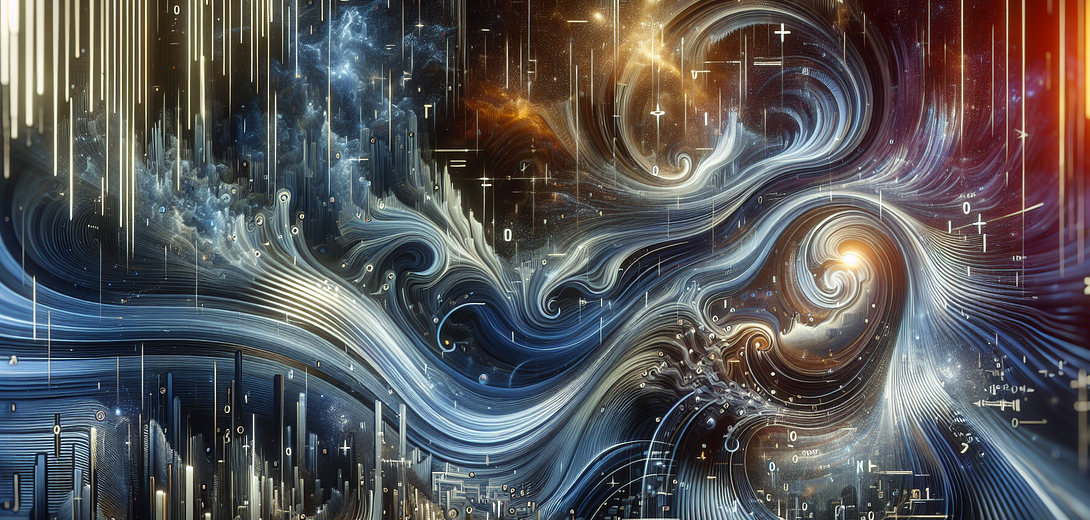
In the evolving landscape of software development, integrating AI into your workflow can drastically enhance efficiency and code quality. Whether you’re a seasoned C++ developer or just starting, leveraging the Cloving CLI, a robust, AI-powered command-line interface, can redefine your development workflow. This post will guide you through using Cloving CLI to streamline C++ application development, offering practical tips and examples that’ll show you how to become more effective in your programming tasks.
Setting the Stage
Before we can dive into the nitty-gritty of using Cloving CLI for C++ development, let’s get everything set up correctly.
Installation
Begin by installing Cloving if you haven’t done so already. You can easily install it globally using npm:
npm install -g cloving@latest
Configuration
Once installed, configure Cloving to use your desired AI model and set up an API key, which you’ll need to access its entire suite of features:
cloving config
Follow the interactive prompts to complete this configuration.
Initialization
Ensure Cloving recognizes your C++ project by initializing it in your project directory:
cloving init
Running this will build a cloving.json
file that holds metadata, providing Cloving with the necessary context for your project.
Enhancing C++ Development with Cloving CLI
With the setup done, let’s explore the ways Cloving CLI can aid C++ development.
1. AI-Driven Code Generation
Suppose you’re implementing a class to handle a mathematical vector in a C++ project. Use Cloving’s code generation feature to create this class with ease:
cloving generate code --prompt "Create a C++ class for a 3D vector with methods for addition and dot product" --files src/vector.cpp
This command leverages your current project context and the model’s AI capabilities to create boilerplate code:
// src/vector.cpp
#include <iostream>
class Vector3D {
public:
float x, y, z;
Vector3D(float x, float y, float z) : x(x), y(y), z(z) {}
Vector3D operator+(const Vector3D& other) const {
return Vector3D(x + other.x, y + other.y, z + other.z);
}
float dot(const Vector3D& other) const {
return (x * other.x) + (y * other.y) + (z * other.z);
}
};
By generating boilerplate code, Cloving saves you from repeatedly writing tedious components.
2. Interactive Code Improvement
You can enhance the generated code further using the interactive mode. For instance, if you want to add a subtraction method and ensure proper memory management, simply continue with:
cloving generate code --interactive
Revise Vector3D class to include subtraction and handle memory management
Here, the AI will assist you in making iterative improvements and ensuring best practices are followed.
3. Seamless Unit Test Generation
Ensuring robust C++ applications involves thorough testing. Use Cloving to generate unit tests for your Vector3D class:
cloving generate unit-tests -f tests/vector_tests.cpp
The AI will create meaningful unit tests, enhancing reliability, without needing you to write every test from scratch:
// tests/vector_tests.cpp
#include "vector.cpp" // Assume the initial header code is present
#include <cassert>
int main() {
Vector3D v1(1.0f, 2.0f, 3.0f);
Vector3D v2(4.0f, 5.0f, 6.0f);
// Test Addition
Vector3D v3 = v1 + v2;
assert(v3.x == 5.0f && v3.y == 7.0f && v3.z == 9.0f);
// Test Dot Product
float dot = v1.dot(v2);
assert(dot == 32.0f);
std::cout << "All tests passed!" << std::endl;
return 0;
}
4. Cloving Chat for Active Assistance
When complex questions or tasks arise, such as integration with other libraries or debugging, leverage the interactive Cloving chat:
cloving chat -f src/vector.cpp
This starts a chat-based session where you can ask the AI for assistance in real time:
cloving> Explain how to integrate this Vector3D class with an existing physics library
The AI will assist with clear explanations and integration strategies, allowing you to progress efficiently.
5. AI-Guided Commit Message Generation
Once you’re satisfied with your progress, make use of Cloving’s commit generation to create informed commit messages that succinctly capture your changes:
cloving commit
The AI analyzes modifications and proposes a commit message, streamlining your version control workflow.
Conclusion
Using Cloving CLI for C++ development can streamline processes, improve code quality, and bolster productivity. By leveraging its AI-driven capabilities, you reduce redundancy, encourage best practices, and make room for focusing on complex, critical tasks. Embrace the future of coding with Cloving, and watch your development efficiency soar. Remember, though Cloving augments your workflow, your expertise and intuitive creativity remain irreplaceable.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.