Streamline Java Spring Functions with AI-Based Code Enhancement
Updated on March 07, 2025

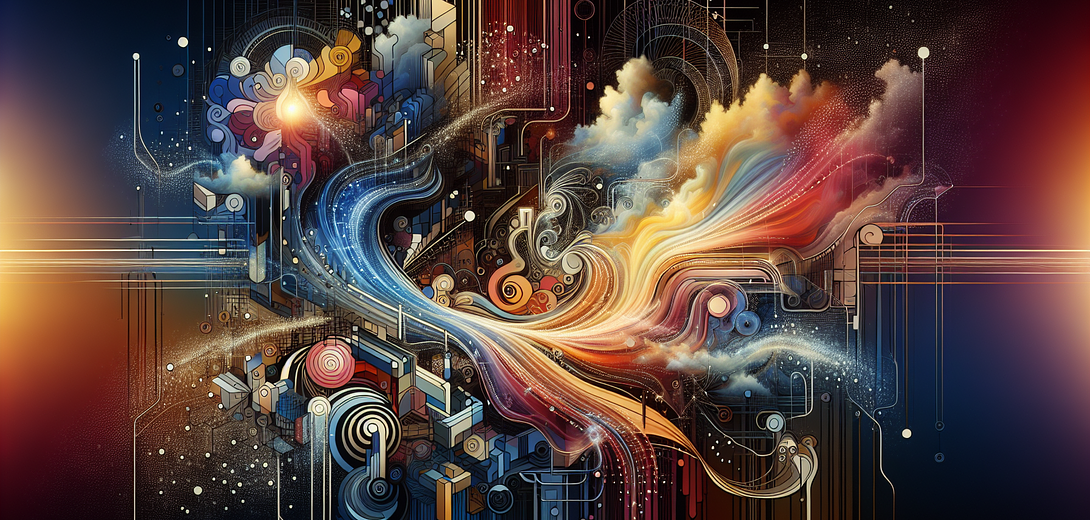
#Boosting Your Java Spring Functions with Cloving CLI
Java Spring is a popular framework for building scalable, high-performance applications. Yet creating robust REST endpoints, services, and tests can be time-consuming. Cloving CLI, an AI-powered command-line tool, streamlines this process by integrating GPT-based solutions directly into your development workflow. Let’s explore how Cloving can help you accelerate your Java Spring projects, from generating new endpoints to refining code and tests.
1. Introducing Cloving CLI
Cloving CLI acts like an AI pair programmer, assisting you with:
- Generating or refining Java Spring code
- Reviewing existing code for quality and best practices
- Building unit tests or integration tests
- Interacting via an AI chat for advanced questions or iterative improvements
- Facilitating Git commits with AI-suggested messages
By tailoring its outputs to your project’s context, Cloving can drastically reduce boilerplate and repetitive tasks.
2. Setting Up Cloving
2.1 Installation
Install Cloving globally via npm:
npm install -g cloving@latest
2.2 Configuration
Configure Cloving by providing your API key and AI model:
cloving config
Follow the interactive prompts (e.g., GPT-3.5, GPT-4). Once complete, Cloving can generate code for your Java Spring environment.
3. Initializing Your Java Spring Project
In the root directory of your Spring project (likely containing pom.xml
or build.gradle
), run:
cloving init
Cloving analyzes your folder structure, discovering relevant Java packages, possibly scanning your existing code to capture style or naming conventions. This creates a cloving.json
that stores metadata for more context-aware generation.
4. Enhancing Java Spring Functions with Cloving
4.1 Example: Creating a User Registration Endpoint
Suppose you need a new REST endpoint for user registration. Prompt Cloving:
cloving generate code --prompt "Create a new endpoint for user registration in a Java Spring application" --files src/main/java/com/example/springapp
Sample Output:
// src/main/java/com/example/springapp/UserController.java
package com.example.springapp;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/api/users")
public class UserController {
private final UserService userService;
@Autowired
public UserController(UserService userService) {
this.userService = userService;
}
@PostMapping("/register")
public ResponseEntity<User> registerUser(@RequestBody User user) {
User registeredUser = userService.saveUser(user);
return new ResponseEntity<>(registeredUser, HttpStatus.CREATED);
}
}
Notes:
- Cloving references typical Spring patterns, like
@RestController
,@RequestMapping
, andResponseEntity
. - If you have a user model or service in your codebase, Cloving can incorporate them or create stubs for new ones if you mention them in your prompt.
4.2 Refining the Generated Code
If you want to add input validation, mention it:
Revise the user registration endpoint to include input validation checks
Cloving might add checks for user.getUsername() != null
, or reference a validation framework (@Valid
, @NotBlank
, etc.).
5. Reviewing and Updating Code
5.1 Interactive Chat Mode
For more advanced or iterative tasks—like integrating a new caching layer or refining error handling—open Cloving chat:
cloving chat -f src/main/java/com/example/springapp/UserService.java
Inside the chat, you can:
cloving> Optimize the UserService for concurrency and add caching for user data
Cloving can then propose changes or expansions (e.g., adding @Cacheable
from Spring Cache, leveraging concurrency patterns, or rewriting loops for improved performance).
5.2 Code Review
Use cloving generate review
to have Cloving scan your project and highlight potential improvements, from missing exception handling to unused imports or potential memory usage concerns.
6. Generating Unit Tests
Testing is key to maintaining a robust Spring application. Let Cloving build baseline tests:
cloving generate unit-tests -f src/main/java/com/example/springapp/UserService.java
Sample Output:
// src/test/java/com/example/springapp/UserServiceTest.java
package com.example.springapp;
import static org.junit.jupiter.api.Assertions.*;
import static org.mockito.Mockito.*;
import org.junit.jupiter.api.Test;
import org.mockito.Mock;
class UserServiceTest {
@Mock
private UserRepository userRepository; // Assume there's a UserRepository
@Test
void testSaveUser() {
UserService userService = new UserService(userRepository);
User user = new User("username", "password");
when(userRepository.save(user)).thenReturn(user);
User savedUser = userService.saveUser(user);
assertNotNull(savedUser);
verify(userRepository, times(1)).save(user);
}
}
Notes:
- If you prefer a certain test style (Junit 4 vs. Junit 5, or different mocking frameworks), mention them in your prompt or show an existing test reference.
- Expand coverage for negative scenarios or advanced logic, as Cloving’s generated tests are a foundation.
7. Integrating Cloving into Your Workflow
7.1 GIT Commit Messages
After editing or adding new functionality, let Cloving craft a commit message:
cloving commit
Cloving’s AI inspects staged changes and suggests a message, e.g., “Add user registration endpoint with input validation and tests.” You can edit or accept it.
7.2 CI/CD Pipeline
You could incorporate Cloving commands in a DevOps pipeline for automatic code generation or test scaffolding. For large teams, ensure to carefully review AI outputs to maintain consistency and handle domain-specific intricacies.
8. Best Practices
- Initialize in the Right Place
Always runcloving init
at your project’s root to give the AI full knowledge of your structure (e.g.,src/main/java
,pom.xml
orbuild.gradle
). - Provide Context
When generating endpoints or classes, mention any details about the model, repository, or style guidelines. - Iterate
For complex tasks (like advanced data flows or specialized caching), rely on the chat environment to refine multiple times. - Review
Usecloving generate review
or your own knowledge to confirm correctness, performance, and alignment with your domain. - Refine Tests
Cloving’s test stubs are helpful, but always expand them for corner cases, negative paths, or concurrency aspects if relevant.
9. Example Workflow
Below is a typical sequence to adopt Cloving in a Spring environment:
- Initialize:
cloving init
in your project root. - Generate:
cloving generate code --prompt "Create a new endpoint for product creation" --files src/main/java/com/example/springapp
. - Refine: Use
cloving chat
to incorporate custom error handling or advanced logic (e.g. concurrency, transaction management). - Test:
cloving generate unit-tests -f src/main/java/com/example/springapp/ProductService.java
. - Commit: Let Cloving craft an AI-generated message with
cloving commit
. - Review:
cloving generate review
if you need a thorough check or are merging a big feature.
10. Conclusion
By integrating Cloving CLI into your Java Spring projects, you can simplify the creation and refinement of new features, improve testing coverage, and maintain consistent code quality. Cloving’s GPT-based assistance reduces boilerplate and helps you keep pace with evolving demands in enterprise Java applications.
Remember: Cloving is here to assist, not replace your expertise. While it handles routine tasks quickly, your domain knowledge and final review ensure correctness, security, and performance. Embrace AI in your development pipeline for a more efficient, streamlined, and enjoyable Java Spring development experience.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.