Speeding Up Your API Development in Flask with Automated Code Generation
Updated on April 14, 2025

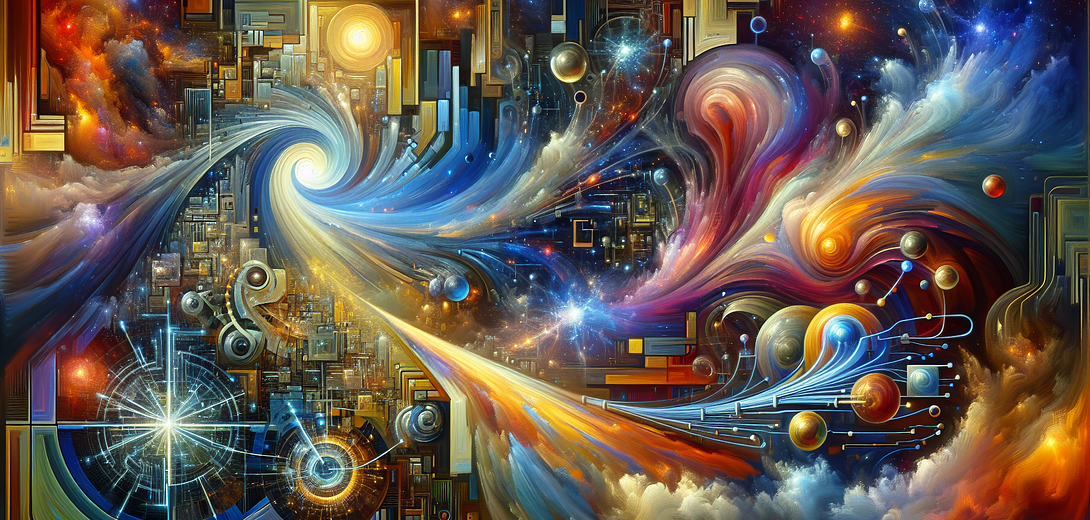
In the fast-moving world of software development, leveraging AI tools to accelerate your workflow is a game-changer. With the Cloving CLI tool, you can streamline your API development in Flask by automating code generation tasks, ultimately improving both speed and code quality. In this post, we’ll explore how to effectively use the Cloving CLI to enhance your Flask API projects.
Introduction to Cloving CLI
Cloving is a command-line interface tool that integrates AI into your development process. It serves as an AI-assisted pair programmer, providing intelligent code suggestions and automating repetitive tasks, enhancing your productivity and code quality.
1. Getting Started with Cloving
Before diving into automated code generation, let’s ensure Cloving is set up and ready to use in your development environment.
Installation:
Install Cloving globally with npm:
npm install -g cloving@latest
Configuration:
Set up your Cloving environment by configuring your preferred AI model:
cloving config
Follow the interactive setup prompts to enter your API key and select the models you wish to use.
2. Initialize Your Flask Project
For Cloving to provide contextually relevant suggestions, initialize it within your Flask project directory:
cloving init
This command generates a cloving.json
file that contains metadata and establishes the context of your project.
3. Automated Code Generation
With Cloving configured, let’s explore how to automate code generation for a Flask API.
Example:
Suppose you need to create a new endpoint in your Flask application for user registration. You can use the Cloving code generation command to achieve this:
cloving generate code --prompt "Create a Flask endpoint to register a new user" --files app.py
The tool will analyze the project context and generate a relevant code snippet for your Flask endpoint. Here’s an example of what it might generate:
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route('/register', methods=['POST'])
def register_user():
data = request.json
username = data.get('username')
password = data.get('password')
# Add logic to store user information securely
return jsonify({"message": "User registered successfully"}), 201
if __name__ == '__main__':
app.run(debug=True)
4. Review and Refine Generated Code
Cloving not only generates code but also lets you review and refine it. After generating the Flask endpoint, you might decide to enhance it with additional features, such as password validation or error handling. Use the interactive features to revise the snippet:
Enhance the register_user endpoint to include password validation and error handling.
5. Generate Unit Tests for Your API
To ensure your endpoints are robust and maintainable, it’s crucial to write unit tests—Cloving can help with that too.
cloving generate unit-tests -f app.py
Cloving will generate unit tests for your Flask application, facilitating test coverage and reliability. For instance:
import unittest
from app import app
class FlaskAPITestCase(unittest.TestCase):
def setUp(self):
self.app = app.test_client()
self.app.testing = True
def test_user_registration(self):
response = self.app.post('/register', json={"username": "testuser", "password": "pass123"})
self.assertEqual(response.status_code, 201)
self.assertIn(b'User registered successfully', response.data)
if __name__ == '__main__':
unittest.main()
6. Interactive Chat for Complex Queries
For complex tasks where you require ongoing guidance, utilize the Cloving chat functionality:
cloving chat -f path/to/file.py
In this interactive REPL, you can ask Cloving for assistance, gain insights, and generate code snippets interactively, all within the context of your project.
7. Generate Meaningful Commit Messages
Once you’ve successfully integrated your new endpoint and tests, use Cloving to create informative commit messages:
cloving commit
Cloving will analyze the changes and provide a well-structured commit message which you can further edit if needed.
Conclusion
Using Cloving CLI to automate code generation for your Flask API projects can significantly enhance your development workflow, reduce redundant tasks, and improve code quality. By leveraging AI-powered tools like Cloving, you can focus more on crafting the logic and functionality of your APIs, while leaving the repetitive chores to the machine.
Remember to adapt Cloving’s capabilities to complement your skills and amplify your productivity. Embrace AI in your development workflow and witness the transformation in efficiency and output quality.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.