Revolutionizing Backend Development Using GPT for GoLang Code Generation
Updated on March 30, 2025

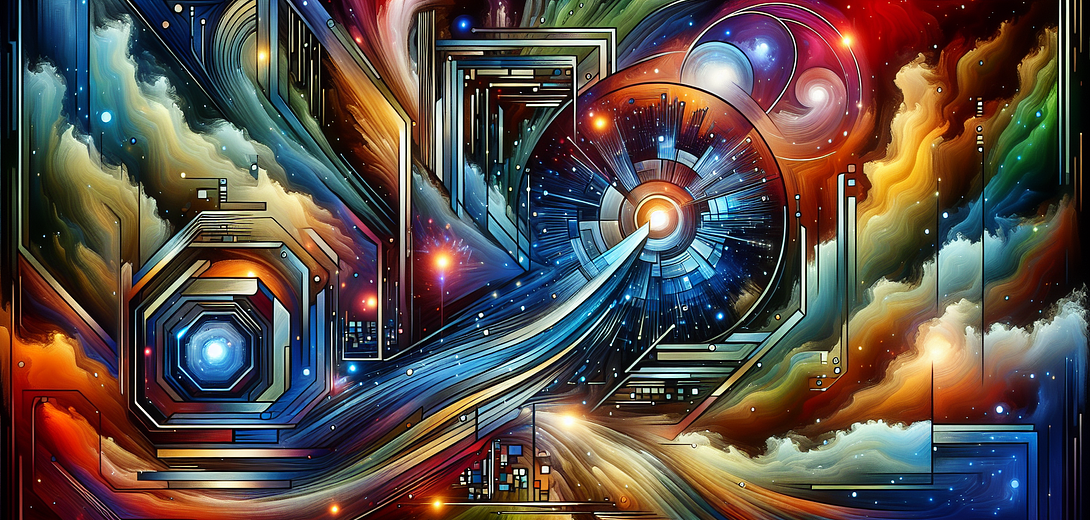
Boosting GoLang Backend Development with Cloving CLI
Go (Golang) has become a powerful choice for building high-performance, scalable backends and microservices. However, creating robust endpoints, managing authentication, and consistently writing unit tests can be demanding. Cloving CLI, an AI-powered tool, brings GPT-based intelligence directly into your workflow to generate, review, and refine Go code. In this guide, we’ll demonstrate how Cloving can streamline your GoLang backend development, saving time and enhancing code quality.
1. Introduction to Cloving CLI
Cloving CLI is an AI-driven command-line interface that helps developers by:
- Generating code in different languages, including Go
- Reviewing existing code for potential improvements
- Refining logic via interactive “chat” mode
- Creating or expanding unit tests for code coverage
- Drafting commit messages that provide clear context
By analyzing your project’s structure and style, Cloving outputs code that aligns with your environment, letting you focus on higher-level design and features.
2. Setting Up Cloving
2.1 Installation
Install Cloving globally using npm:
npm install -g cloving@latest
2.2 Configuration
After installing, configure Cloving by providing your API key and choosing an AI model:
cloving config
Follow the interactive prompts to finalize your environment, ensuring Cloving is ready to generate or refine your GoLang code.
3. Initializing Your GoLang Project
Within the root folder of your Go project (typically containing go.mod
or your main application files), run:
cloving init
Cloving scans your directory, discovering relevant packages, dependencies, or existing structure. It then creates a cloving.json
file, storing metadata to help produce context-aware code.
4. Generating GoLang Code with Cloving
4.1 Example: JWT Authentication Function
Imagine you need a function to handle user authentication using JWT. Cloving can scaffold this code for you:
cloving generate code --prompt "Develop a GoLang function for user authentication using JWT" --files auth.go
Sample Output:
// auth.go
package auth
import (
"fmt"
"time"
"github.com/dgrijalva/jwt-go"
)
func GenerateToken(userID string) (string, error) {
signingKey := []byte("mysecret")
token := jwt.NewWithClaims(jwt.SigningMethodHS256, jwt.MapClaims{
"userid": userID,
"exp": time.Now().Add(time.Hour * 72).Unix(),
})
tokenString, err := token.SignedString(signingKey)
if err != nil {
return "", fmt.Errorf("Error signing token: %v", err)
}
return tokenString, nil
}
Notes:
- Cloving typically references known Go JWT libraries (here it uses
github.com/dgrijalva/jwt-go
). - If you prefer a different library, mention it in your prompt (e.g.,
github.com/golang-jwt/jwt
).
4.2 Revising or Enhancing the Code
If you want more advanced logic, such as adding custom claims or changing the signing method, you can prompt Cloving again or open an interactive session to refine the code.
5. Generating Unit Tests
5.1 Test Generation
Ensuring code reliability is crucial. Cloving can create test scaffolding:
cloving generate unit-tests -f auth.go
Sample Output:
// auth_test.go
package auth
import (
"testing"
)
func TestGenerateToken(t *testing.T) {
token, err := GenerateToken("user123")
if err != nil {
t.Errorf("Expected no error, got %v", err)
}
if token == "" {
t.Error("Expected a valid token, got empty string")
}
}
Expand coverage by testing invalid scenarios, e.g., if GenerateToken
fails due to an empty userID or issues with the signing key.
6. Interactive Sessions with Cloving Chat
6.1 The Chat Feature
For iterative tasks—like building advanced microservice features, hooking into a database, or handling concurrency—open Cloving’s chat:
cloving chat -f auth.go
In this interactive environment, you can:
- Ask for concurrency patterns or advanced error handling
- Refine the logic for user roles or additional claims
- Debug unusual errors or performance concerns
Example:
cloving> Add concurrency-safe caching to the token generation if user roles are needed
Cloving might produce code that references goroutines or in-memory caching for user roles, which you can refine further.
7. Streamlining Git Commits
7.1 Generating Commit Messages
After finalizing your new code or updates, use Cloving for AI-driven commit messages:
cloving commit
Cloving reviews your staged changes, offering a summary (e.g., “Add JWT-based user authentication and initial test coverage in auth.go”). You can accept or edit for clarity before committing.
8. Best Practices for Cloving + Go
- Initialize in Each Service
If working on a microservices architecture, runcloving init
in each separate Go service to ensure each codebase is recognized distinctly. - Iterate
Start with a base function and refine it multiple times. Cloving’s chat environment can incorporate new features or handle advanced concurrency as your microservice evolves. - Review
If your code is critical or domain-specific, always manually review AI-generated logic or runcloving generate review
to highlight potential improvements. - Mention Dependencies
If your microservice uses particular frameworks (like Gin, Echo, or standard library only), mention them in the prompt so Cloving can tailor the code accordingly. - Security
Prompt Cloving to adopt best practices, e.g. “Use environment variables for the JWT signing key,” to keep secrets out of code. Validate AI outputs for secure approaches.
9. Example Workflow
Below is a typical approach to adopting Cloving in a Go microservice:
- Initialize:
cloving init
in your Go microservice directory. - Generate:
cloving generate code --prompt "Build a user registration endpoint using the Echo framework" --files user_service.go
. - Test:
cloving generate unit-tests -f user_service.go
for coverage. - Refine: Use
cloving chat -f user_service.go
for iterative improvements, e.g., adding a database or advanced validation. - Commit: Summarize changes with
cloving commit
.
10. Conclusion
Integrating Cloving CLI into your GoLang development workflow provides an efficient, AI-driven method to quickly generate, optimize, and test backend functionality. By offloading repetitive tasks to GPT-based code generation, you can focus on system architecture and innovation while ensuring stable, high-quality code.
Remember: Cloving’s AI suggestions are a foundation—review them for domain-specific correctness, performance constraints, and best practices. Embrace AI-augmented development, and discover how easily you can transform your GoLang microservices with minimal effort and maximum impact.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.