Optimizing ASP.NET Core Application Development with GPT Assistance
Updated on November 27, 2024

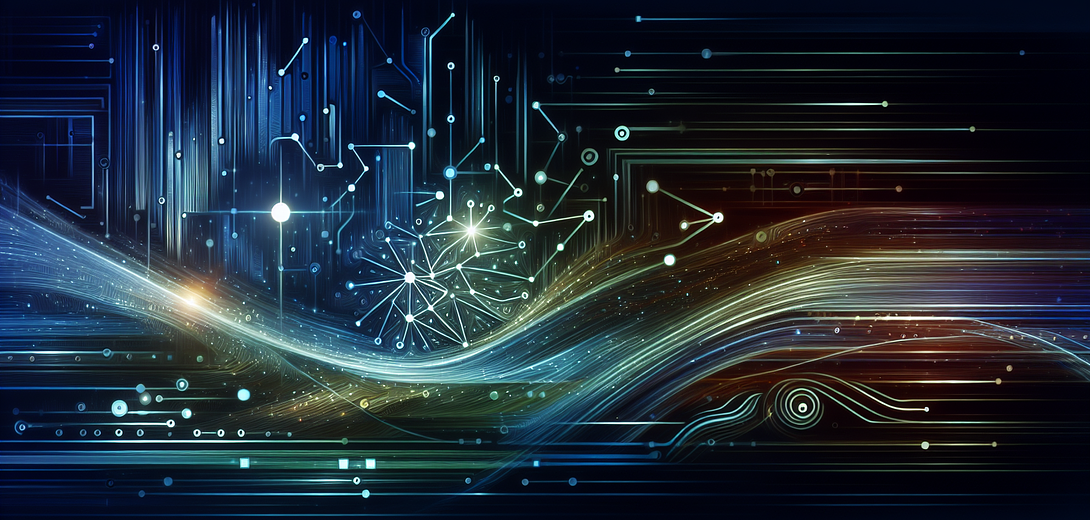
As an ASP.NET Core developer, you’re always looking for ways to enhance productivity and optimize your workflow. The Cloving CLI tool, powered by advanced AI models, offers a suite of capabilities that can streamline your development process and elevate code quality. In this tutorial, we’ll delve into how you can leverage Cloving’s features to supercharge your ASP.NET Core projects.
Getting Started with Cloving CLI
Cloving integrates AI into your development workflow, providing code generation, intelligent chat, shell script automation, and more, directly from the command line.
1. Installation and Configuration
Begin by installing Cloving and configuring it for your environment:
Install Cloving:
npm install -g cloving@latest
Configure Your Setup:
Run the configuration command to set up your API key and preferred AI model:
cloving config
Follow the prompts to complete the configuration.
2. Initializing Your ASP.NET Core Project
To start optimizing your ASP.NET Core application, initialize Cloving in your project directory:
cloving init
This step is crucial as it allows Cloving to understand your project’s context fully, setting the stage for productive interactions.
3. Generate ASP.NET Core Code Snippets
Cloving can generate code snippets tailored to your project’s needs. For example, if you’re building a REST API and need a new controller for managing orders, use the following command:
cloving generate code --prompt "Create an ASP.NET Core controller for managing orders with CRUD operations" --files src/Controllers/OrdersController.cs
Cloving will analyze the existing project structure and generate a relevant controller class. Here’s a sample output:
// src/Controllers/OrdersController.cs
using Microsoft.AspNetCore.Mvc;
using YourNamespace.Models;
namespace YourNamespace.Controllers
{
[ApiController]
[Route("api/[controller]")]
public class OrdersController : ControllerBase
{
[HttpGet]
public IActionResult GetAllOrders()
{
// Retrieve and return all orders
}
[HttpGet("{id}")]
public IActionResult GetOrder(int id)
{
// Retrieve and return order by id
}
[HttpPost]
public IActionResult CreateOrder(Order order)
{
// Create a new order
}
[HttpPut("{id}")]
public IActionResult UpdateOrder(int id, Order order)
{
// Update an existing order
}
[HttpDelete("{id}")]
public IActionResult DeleteOrder(int id)
{
// Delete an order
}
}
}
4. Generating Unit Tests
Ensure your ASP.NET Core application is robust by generating unit tests with Cloving. To create tests for the order controller:
cloving generate unit-tests -f src/Controllers/OrdersController.cs
Cloving will provide unit tests to verify the functionality of your controller methods:
// src/Controllers/OrdersController.test.cs
using Xunit;
using YourNamespace.Controllers;
public class OrdersControllerTests
{
[Fact]
public void GetAllOrders_ShouldReturnAllOrders()
{
// Arrange
var controller = new OrdersController();
// Act
var result = controller.GetAllOrders();
// Assert
Assert.NotNull(result);
}
// Additional test methods
}
5. Utilize Cloving Chat for Troubleshooting and Improvements
For complex queries or when you’re stuck, the interactive chat feature can be invaluable:
cloving chat -f src/Controllers/OrdersController.cs
With chat mode, you can iteratively improve your code, ask for explanations, or seek suggestions for enhancements. The AI assists in real-time, providing insights tailored to your codebase.
6. Optimize Workflow with Shell Scripts and Commit Messages
Automate common tasks with shell scripts generated by Cloving:
cloving generate shell --prompt "Automate the build and test sequence for my ASP.NET Core project"
For AI-generated commit messages that accurately reflect your changes, leverage Cloving:
cloving commit
This command aids in crafting commit messages that capture the essence of your updates, improving team communication and project documentation.
Conclusion
Integrating Cloving CLI into your ASP.NET Core development workflow offers enhanced productivity, quality code generation, and streamlined operations. By using AI-driven capabilities, developers can focus on building innovative solutions while Cloving handles the repetitive and complex coding tasks.
Cloving empowers you to optimize your development process, making it an essential tool for the modern ASP.NET Core developer. Embrace this technology to transform how you build, test, and deploy applications.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.