Maximizing Scala Application Performance with AI-Powered Code Generation
Updated on January 06, 2025

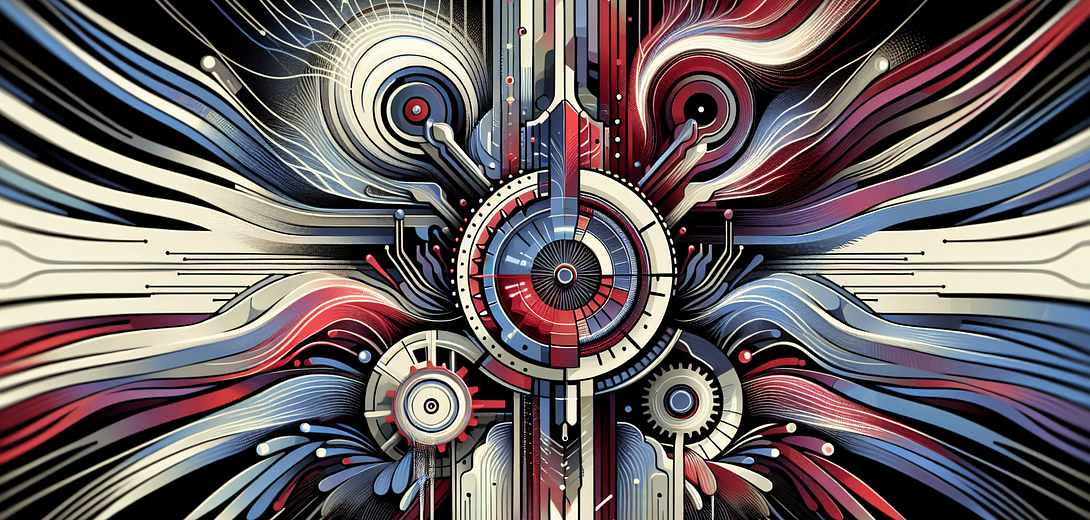
In recent years, Scala has gained popularity for its functional programming features and strong type system, making it an ideal choice for building high-performance applications. However, as applications grow in complexity, maintaining performance can become a challenging task. Enter the Cloving CLI tool - an AI-powered command-line interface that assists developers in optimizing their Scala applications by generating efficient code and enhancing code quality. In this blog post, we will explore how to maximize Scala application performance using Cloving’s powerful features.
Getting Started with Cloving CLI
To begin leveraging the Cloving CLI tool in your Scala projects, you’ll first need to install and configure it.
Installation
Install Cloving globally via npm:
npm install -g cloving@latest
Configuration
Use the config
command to set up Cloving with your API key and preferred AI model:
cloving config
Follow the interactive setup prompts to provide your API key and model preferences.
Initializing Your Scala Project
Initialize Cloving in your Scala project directory to enable context-aware code generation:
cloving init
Executing this command generates a cloving.json
file, containing metadata about your application and its setup.
Unlocking the Power of Cloving CLI
1. Generating Scala Code
Harness the power of AI to generate Scala code snippets that can improve your application’s performance.
Example:
Suppose you need a more performant function to filter primes in a list. Use the generate code
command to achieve that:
cloving generate code --prompt "Efficiently filter prime numbers from a list in Scala"
Cloving will use its AI-generated insights to create a fast and efficient function. An example output might look like:
def isPrime(n: Int): Boolean = {
if (n <= 1) return false
if (n <= 3) return true
if (n % 2 == 0 || n % 3 == 0) return false
var i = 5
while (i * i <= n) {
if (n % i == 0 || n % (i + 2) == 0) return false
i = i + 6
}
true
}
def filterPrimes(numbers: List[Int]): List[Int] = {
numbers.filter(isPrime)
}
2. Optimizing and Reviewing Code
Following code generation, use Cloving to review and optimize existing Scala code for enhanced performance.
Start an Interactive Chat
For complex performance optimizations, start an interactive chat with Cloving:
cloving chat -f src/main/scala/Example.scala
Within the chat, you can request code optimization suggestions or ask Cloving to review specific parts of your code. Simply provide instructions like:
Optimize the List processing logic for better performance
AI-Assisted Code Review
Leverage Cloving’s AI-powered code review feature to identify potential performance bottlenecks and areas for improvement:
cloving generate review
Receive a detailed review highlighting inefficient code patterns, along with suggestions for improvement. For example:
# Code Review for src/main/scala/Example.scala
## Overview
The codebase demonstrates good practices but can be enhanced by optimizing list transformations and employing lazy evaluation.
## Suggestions
1. Replace `map` followed by `filter` with a single `collect` operation.
2. Use Scala's `Stream` for computations that benefit from lazy evaluation.
3. Consider parallelizing computations using Scala's `Futures` for CPU-bound tasks.
3. Generating Unit Tests
Ensure your code changes don’t introduce regressions by generating comprehensive unit tests with Cloving:
cloving generate unit-tests -f src/main/scala/Example.scala
A sample generated test file might include:
import org.scalatest.flatspec.AnyFlatSpec
class ExampleTests extends AnyFlatSpec {
"isPrime" should "correctly identify prime numbers" in {
assert(isPrime(2) == true)
assert(isPrime(4) == false)
}
"filterPrimes" should "accurately filter prime numbers from a list" in {
assert(filterPrimes(List(1, 2, 3, 4, 5, 6, 7, 8, 9, 10)) == List(2, 3, 5, 7))
}
}
Enhancing Productivity with Cloving
Automatic Commit Message Generation
Cloving facilitates better version control practices by generating descriptive commit messages:
cloving commit
Expect concise and informative messages like:
Refactor isPrime function to improve performance
Conclusion
By integrating the Cloving CLI tool into your Scala development workflow, you can significantly enhance both performance and code quality. Utilize its AI-powered code generation capabilities to write efficient code, conduct insightful reviews, and maintain robust unit tests. Cloving empowers you to focus on the creative aspects of programming, transforming how you approach application performance.
Maximize your Scala application performance today with Cloving, your AI-powered assistant in the command line.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.