Leveraging GPT for Seamless Debugging in Java Applications
Updated on December 05, 2024

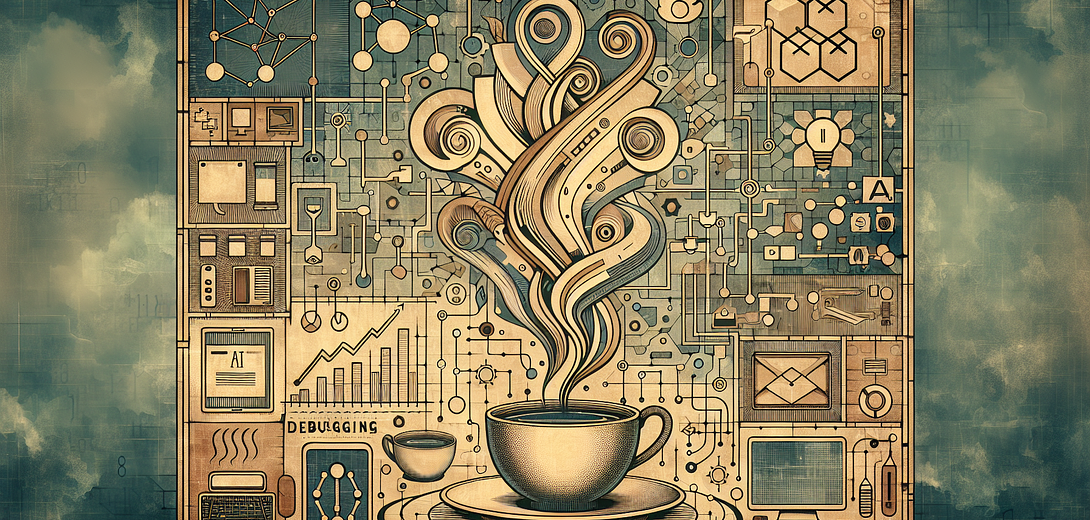
In the world of Java development, debugging can often prove to be a time-consuming and tedious task. However, with the power of AI and the Cloving CLI tool, you can leverage GPT models to transform your debugging workflow into a seamless, efficient process. In this post, we’ll explore how to use Cloving CLI for effective debugging in Java applications, sharing practical examples and best practices to enhance your productivity.
Understanding the Cloving CLI
Cloving integrates AI into your development workflow, offering tools for generating code, reviewing it, and assisting with debugging tasks using sophisticated language models.
1. Setting Up Cloving for Java Projects
Before diving into debugging, ensure Cloving is correctly configured for your environment.
Installation:
Install Cloving globally using npm:
npm install -g cloving@latest
Configuration:
Set up Cloving to use your preferred AI models and API key:
cloving config
Follow the interactive setup prompts to configure Cloving with the appropriate AI models.
2. Initializing Your Java Project with Cloving
Initialize Cloving in the Java project directory so it can understand your project’s context.
cloving init
This will scan the project, allowing Cloving to work efficiently with your Java files.
3. Using Cloving Chat for Debugging Assistance
One of Cloving’s standout features is its interactive chat interface, which can assist with troubleshooting and debugging.
Example:
Suppose you’re encountering an ArrayIndexOutOfBoundsException
in your Java project, and you want assistance with debugging.
cloving chat -f src/main/java/com/example/app/MyApp.java
Once in the chat session, you can describe your issue and get guidance like this:
cloving> I'm getting an ArrayIndexOutOfBoundsException in MyApp.java. Can you help me fix it?
Cloving might respond with an explanation of common causes of this error, suggests examining loop logic or array access, and even highlights the offending code parts.
4. Generating Debuggable Code
Cloving can help suggest corrections by generating revised code snippets. Suppose you need help fixing a null pointer exception:
cloving generate code --prompt "Fix null pointer exception in the processOrder method" --files src/main/java/com/example/app/OrderProcessor.java
The tool analyzes the code and generates a more robust version with potential null checks.
// src/main/java/com/example/app/OrderProcessor.java
public void processOrder(Order order) {
if (order != null) {
// Process the order
} else {
throw new IllegalArgumentException("Order cannot be null");
}
}
5. Generating Unit Tests for Debugging
Unit tests are crucial for verifying that debugging fixes are effective. Cloving can generate tests to ensure your patch is reliable.
cloving generate unit-tests -f src/main/java/com/example/app/OrderProcessor.java
This will produce tests targeting the recently debugged code, verifying it works in various scenarios.
// src/test/java/com/example/app/OrderProcessorTest.java
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class OrderProcessorTest {
@Test
public void testProcessOrder_withValidOrder() {
Order order = new Order(); // assume Order object is correctly created
OrderProcessor processor = new OrderProcessor();
assertDoesNotThrow(() -> processor.processOrder(order));
}
@Test
public void testProcessOrder_withNullOrder() {
OrderProcessor processor = new OrderProcessor();
Exception exception = assertThrows(IllegalArgumentException.class, () -> {
processor.processOrder(null);
});
assertEquals("Order cannot be null", exception.getMessage());
}
}
6. Leveraging AI for Code Reviews
As part of the debugging process, performing code reviews can help catch potential issues early.
cloving generate review
This command will analyze your recent code changes, providing feedback and suggestions that might help pinpoint overlooked bugs or better approaches.
7. Improving Commit Quality with AI
After debugging, ensure high-quality commits using Cloving’s commit message generation:
cloving commit
This helps ensure the commit message reflects the debugging process, providing context for future code evaluations.
Conclusion
By incorporating Cloving CLI into your Java debugging workflow, you can significantly enhance your productivity, streamline tasks, and improve code quality. Leveraging AI to assist with debugging allows you to focus more on crafting elegant solutions rather than getting bogged down by repetitive and time-consuming tasks.
Remember: While Cloving empowers your debugging with AI, it complements but does not replace your problem-solving skills. Treat it as a powerful extension of your development toolkit, augmenting your ability to debug and understand code effectively.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.