Leveraging GPT for Modular Code Structures in Modern JavaScript Frameworks
Updated on April 21, 2025

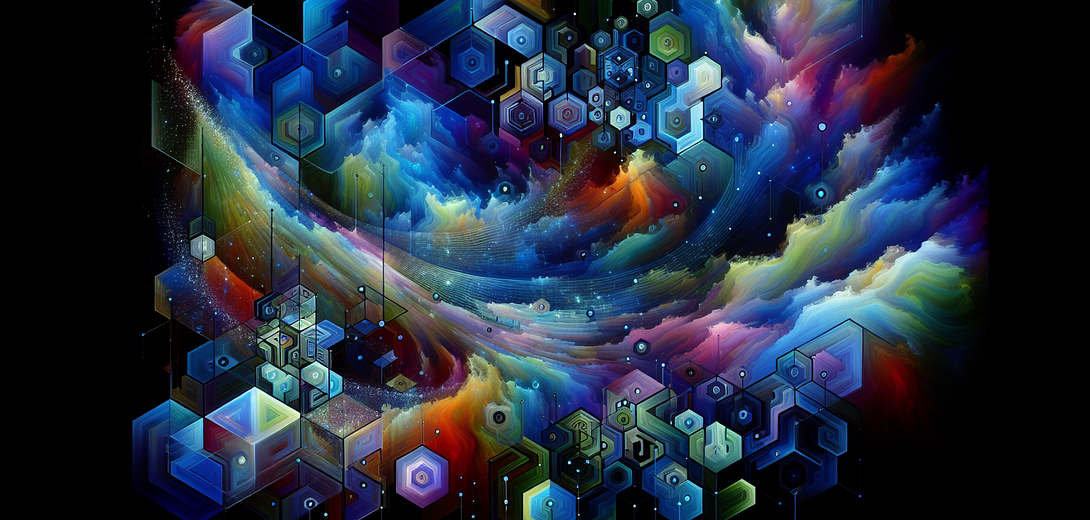
In the ever-evolving landscape of software development, maintaining a modular code structure is critical for managing complexity and ensuring scalability. The Cloving CLI tool, powered by AI, provides developers with the means to leverage GPT-like models to generate, refactor, and improve modular code within modern JavaScript frameworks like React, Angular, and Vue.js. In this post, we’ll explore practical ways to integrate Cloving CLI into your workflow to create robust modular architectures.
Understanding Modular Code Structures
Modular code structures promote the separation of concerns by allowing different parts of an application to be developed, tested, and maintained independently. This is achieved through the use of modules, components, or services that encapsulate specific functionality.
Benefits of Modular Code
- Maintainability: Easier to understand and modify.
- Reusability: Components can be reused across different projects.
- Testability: Small, isolated units are easier to test.
- Scalability: Facilitates scaling of applications with minimal friction.
Using Cloving CLI for Modular Code
The Cloving CLI provides a suite of commands that can assist you in maintaining modularity by generating code, creating unit tests, and performing code reviews. Let’s dive into practical uses of Cloving CLI in modern JavaScript frameworks.
1. Setting Up Cloving for Your Project
Before diving into code generation, set up Cloving in your project environment:
Installation:
Install Cloving globally using npm:
npm install -g cloving@latest
Configuration:
Configure Cloving to use your preferred AI model:
cloving config
Follow the prompts to set up your API key and model preferences.
Initialization:
Initialize Cloving in your project directory:
cloving init
This command will analyze your project and set up necessary configurations.
2. Generating Modular Components
Suppose you’re working on a React project and need a reusable component for a user profile card. Using Cloving, you can seamlessly generate a modular React component:
cloving generate code --prompt "Create a React component for a user profile card" --files src/components/ProfileCard.jsx
Generated Code Example (ProfileCard.jsx):
import React from 'react';
const ProfileCard = ({ name, age, location }) => (
<div className="profile-card">
<h2>{name}</h2>
<p>Age: {age}</p>
<p>Location: {location}</p>
</div>
);
export default ProfileCard;
3. Refining and Revising Components
After generating a component, Cloving’s interactive chat allows you to request further revisions or explanations:
Revise the ProfileCard to include an avatar image and social media links.
Revised Code Example:
import React from 'react';
const ProfileCard = ({ name, age, location, avatarUrl, socialLinks }) => (
<div className="profile-card">
<img src={avatarUrl} alt={`${name}'s avatar`} />
<h2>{name}</h2>
<p>Age: {age}</p>
<p>Location: {location}</p>
<div className="social-links">
{socialLinks.map(link => (
<a key={link.platform} href={link.url}>{link.platform}</a>
))}
</div>
</div>
);
export default ProfileCard;
4. Generating Unit Tests for Components
Ensuring your modular components are testable is crucial for maintaining code quality. Cloving can generate unit tests for your components:
cloving generate unit-tests -f src/components/ProfileCard.jsx
Generated Unit Test Example (ProfileCard.test.jsx):
import React from 'react';
import { render } from '@testing-library/react';
import ProfileCard from './ProfileCard';
test('renders ProfileCard component with name and location', () => {
const { getByText } = render(<ProfileCard name="John Doe" age={30} location="New York" />);
expect(getByText("John Doe")).toBeInTheDocument();
expect(getByText("Location: New York")).toBeInTheDocument();
});
5. Conducting Code Reviews
For maintaining high standards, periodic code reviews are essential. Cloving can assist by providing automated code reviews:
cloving generate review
Review Example:
# Code Review: Modular Component Structure Optimization
## Highlights
- Implemented a reusable ProfileCard component, promoting a cohesive design pattern.
- Included prop validation for enhanced robustness.
- Suggested the addition of a default avatar image to improve usability.
## Recommendations
1. Consider using styled-components for managing CSS styles within the components.
2. Evaluate the need for additional prop types such as `email` or `phone number` for the ProfileCard.
6. Leveraging Cloving Chat for Ongoing Support
For more complex tasks or continuous assistance, leverage Cloving’s chat feature:
cloving chat -f src/components/ProfileCard.jsx
Here, you can interact with Cloving to debug, explore architectural patterns, or refine your code further as needed.
Conclusion
Leveraging Cloving CLI to introduce AI into your development workflow can significantly enhance your ability to maintain modular code structures in modern JavaScript frameworks. This not only boosts productivity but also ensures your code base remains clean, maintainable, and scalable.
By adopting Cloving, you get an AI-powered assistant ready to support your coding endeavors, whether it’s generating code, drafting unit tests, or conducting code reviews. Embrace this tool to optimize your development processes and improve code quality across your projects.
Remember: Cloving augments your skills, helping you achieve more with less effort. Happy coding!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.