Leveraging GPT for Asynchronous Task Management in Python
Updated on January 05, 2025

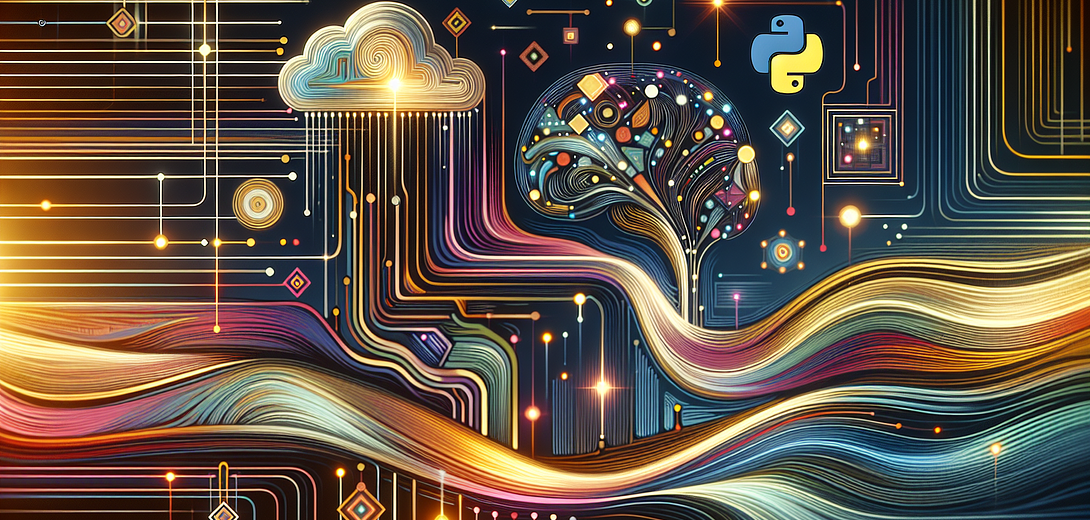
In the world of modern programming, juggling multiple tasks in a non-blocking manner is crucial for efficient application performance. With the rise of AI-driven tools like the Cloving CLI, developers can harness the power of GPT models to manage asynchronous tasks more effectively in Python projects. This tutorial will guide you through integrating Cloving into your Python workflow to optimize asynchronous task handling.
Setting the Stage: Installing and Configuring Cloving
Before diving into asynchronous task management, ensure you have the Cloving CLI set up in your development environment.
Installation
First, install Cloving globally via npm:
npm install -g cloving@latest
Configuration
Set up the Cloving CLI to connect with your AI model through your API key and customize your configuration preferences:
cloving config
Simply follow the interactive prompts to finalize your configuration.
Getting Started: Initialize Cloving in Your Project
Enabling Cloving within your project directory is the first step towards asynchronous task mastery. Use the init
command:
cd your/project/directory
cloving init
This generates a cloving.json
file filled with insights about your project context, ensuring that generated code aligns with your application structure.
Asynchronous Task Management: Unlocking the Potential of Cloving
To tackle asynchronous task management, you’ll utilize Cloving’s capacity for generating and optimizing code snippets with ease.
Use Case: Fetching Data Asynchronously
Let’s say you need to create an asynchronous function that fetches data from multiple APIs concurrently. Cloving can assist you in crafting this with the generate
command:
cloving generate code --prompt "Create an asyncio function that concurrently fetches data from multiple APIs" -f src/fetch_data.py
Example Code Snippet
Cloving might generate the following Python code as a starting point for your async task:
import asyncio
import aiohttp
async def fetch(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
return await response.json()
async def fetch_data_from_apis(api_urls):
tasks = [fetch(url) for url in api_urls]
responses = await asyncio.gather(*tasks)
return responses
This code snippet defines an asynchronous function fetch_data_from_apis
that efficiently gathers data from a list of APIs.
Revising and Enhancing the Code
If you’d like to iterate on the generated code, you can continue the interaction by revising your prompts or using Cloving’s chat feature.
cloving chat -f src/fetch_data.py
In the chat session, request further enhancements:
Can you add error handling to the fetch function?
You’ll receive real-time code revisions, transforming the fetch
function to better manage potential exceptions:
async def fetch(url):
try:
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
return await response.json()
except Exception as e:
print(f"Error fetching data from {url}: {e}")
return None
This improvement ensures robust error management for your asynchronous tasks.
Best Practices: Leveraging Cloving for Ongoing Success
Here are some tips to leverage Cloving effectively for async task management:
-
Utilize Prompts Strategically: Optimize your prompts to generate code snippets tailored for complex asynchronous patterns.
-
Explore Chat for Iterative Development: Use Cloving’s chat mode to tweak and iterate on asynchronously executing tasks; request explanations for better understanding.
-
Commit with Cloving: Document your synchronous to asynchronous code conversions using:
cloving commit
This generates insightful commit messages reflecting your code modifications comprehensively.
-
Generate Reviews for Quality Assurance: Validate your code with Cloving’s code review functionality:
cloving generate review
This ensures adherence to best practices and helps maintain a high standard of code quality.
In conclusion, integrating Cloving with Python for asynchronous task management empowers you to create performant and maintainable code. By leveraging Cloving’s AI capabilities, you can streamline workflows, enhance code stability, and accelerate your development process. Embrace this tech evolution and witness a transformative impact on your projects.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.