Leveraging AI to Scaffold RESTful APIs in Go with GPT
Updated on December 05, 2024

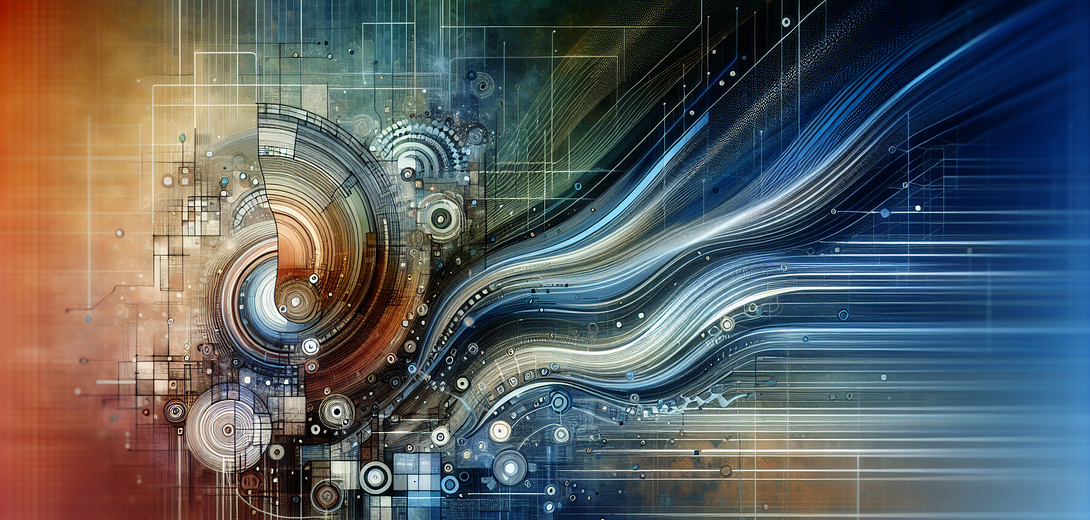
Building RESTful APIs in Go can be a challenging task, especially when balancing ease of use with optimal performance. The Cloving CLI tool comes to the rescue by empowering developers with AI-driven code generation, making the scaffolding of RESTful APIs quicker, efficient, and less error-prone. In this blog post, you’ll learn how to utilize Cloving CLI’s capabilities to speed up the development of RESTful APIs in Go.
Setting Up Cloving CLI
Before harnessing the AI prowess of Cloving CLI, you need to have it installed and configured in your development environment.
Installation
Install Cloving globally using npm:
npm install -g cloving@latest
Configuration
Configure Cloving with your API key and models:
cloving config
Follow the interactive prompts to enter your API key and select your preferred AI models for code generation.
Initializing Your Project
To leverage Cloving’s capabilities, initialize it in your Go project directory:
cloving init
This command will create a cloving.json
file that contains metadata about your project, which is then used by Cloving to understand your project context and assist you more effectively.
Generating RESTful API Scaffolds
Once you’ve set up Cloving, you can start generating RESTful API scaffolds for your Go project.
1. Using Cloving to Generate a RESTful Endpoint
Suppose you wish to create a simple GET
endpoint for retrieving user data. You can use Cloving’s generate code
command:
cloving generate code --prompt "Create a RESTful API endpoint in Go for retrieving user data" --files main.go
This command will evaluate the context of the main.go
file and generate a relevant RESTful API endpoint. Here is what the generated code might look like:
package main
import (
"encoding/json"
"net/http"
"github.com/gorilla/mux"
)
type User struct {
ID string `json:"id,omitempty"`
Name string `json:"name,omitempty"`
Email string `json:"email,omitempty"`
}
var users []User
func GetUserEndpoint(w http.ResponseWriter, req *http.Request) {
params := mux.Vars(req)
for _, user := range users {
if user.ID == params["id"] {
json.NewEncoder(w).Encode(user)
return
}
}
json.NewEncoder(w).Encode(&User{})
}
func main() {
router := mux.NewRouter()
users = append(users, User{ID: "1", Name: "John Doe", Email: "[email protected]"})
router.HandleFunc("/users/{id}", GetUserEndpoint).Methods("GET")
http.ListenAndServe(":8000", router)
}
2. Saving and Reviewing the Code
To save the generated code to your files, use the --save
option:
cloving generate code --prompt "Create a RESTful API endpoint in Go for retrieving user data" --files main.go --save
Once Cloving generates the API endpoint, you can review the code, request explanations, or ask for revisions through an interactive chat session:
cloving chat -f main.go
3. Creating a POST Endpoint
If you want to create a POST
endpoint for adding new users, you can modify the prompt accordingly:
cloving generate code --prompt "Create a RESTful API POST endpoint in Go for adding user data" --files main.go --save
The generated code for a POST
endpoint might look like this:
func CreateUserEndpoint(w http.ResponseWriter, req *http.Request) {
var user User
_ = json.NewDecoder(req.Body).Decode(&user)
users = append(users, user)
json.NewEncoder(w).Encode(user)
}
func main() {
router := mux.NewRouter()
users = append(users, User{ID: "1", Name: "John Doe", Email: "[email protected]"})
router.HandleFunc("/users/{id}", GetUserEndpoint).Methods("GET")
router.HandleFunc("/users", CreateUserEndpoint).Methods("POST")
http.ListenAndServe(":8000", router)
}
With Cloving, adding functionality to your RESTful API is seamless, leveraging AI to ensure your endpoints are created efficiently.
Generating Unit Tests for Your API
To ensure code quality and reliability, you can generate unit tests for your API endpoints:
cloving generate unit-tests -f main.go
This command will create relevant tests that you can directly use or modify for further precision.
Example of a Generated Unit Test
For instance, the generated unit test for GetUserEndpoint
could look like this:
package main
import (
"net/http"
"net/http/httptest"
"testing"
)
func TestGetUserEndpoint(t *testing.T) {
req, err := http.NewRequest("GET", "/users/1", nil)
if err != nil {
t.Fatal(err)
}
rr := httptest.NewRecorder()
handler := http.HandlerFunc(GetUserEndpoint)
handler.ServeHTTP(rr, req)
if status := rr.Code; status != http.StatusOK {
t.Errorf("handler returned wrong status code: got %v want %v", status, http.StatusOK)
}
expected := `{"id":"1","name":"John Doe","email":"[email protected]"}`
if rr.Body.String() != expected {
t.Errorf("handler returned unexpected body: got %v want %v", rr.Body.String(), expected)
}
}
Conclusion
Leveraging Cloving CLI for scaffolding RESTful APIs in Go drastically simplifies the development process. By using AI to generate code, create unit tests, and revise code snippets, developers can focus more on logic and less on boilerplate work. With Cloving, crafting robust and efficient RESTful APIs becomes a straightforward and rewarding experience. Embrace the power of AI-assisted development and transform your programming workflow with Cloving CLI today.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.