Leveraging AI to Enhance C++ Code Concurrency and Performance
Updated on March 31, 2025

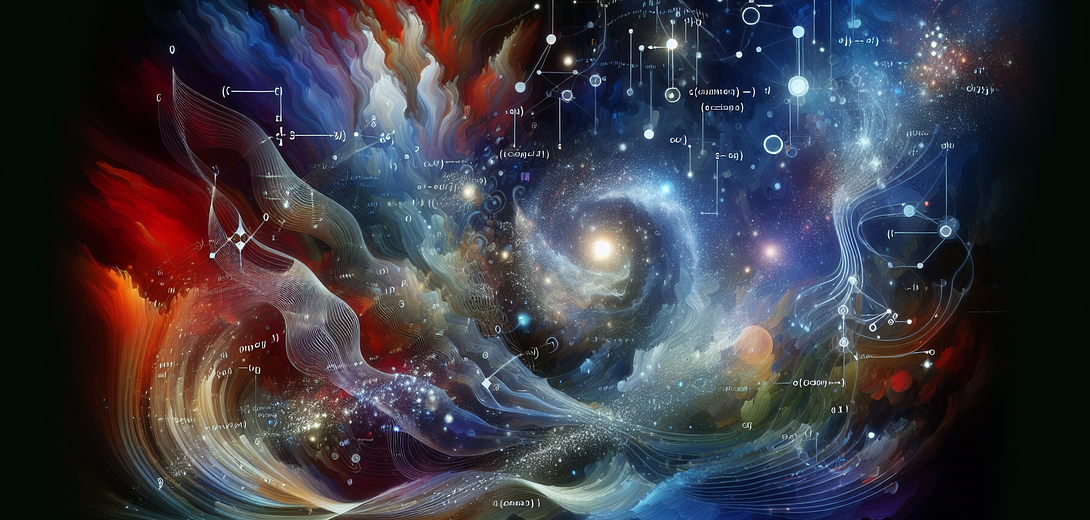
Leveraging AI to Enhance C++ Code Concurrency and Performance
In the realm of software development, C++ remains a favored language for system programming, gaming, and performance-critical applications. However, tapping into its potential, particularly to improve concurrency and performance, can be daunting. Enter the Cloving CLI tool – an AI-powered command-line interface that significantly enhances the productivity and quality of your C++ code. In today’s post, we’ll explore how to leverage Cloving’s features to optimize C++ code concurrency and performance efficiently.
Getting Started with Cloving CLI
Before delving into the specifics of using Cloving CLI for C++ code enhancement, let’s start with setting up the tool in your environment.
Installation:
First, install Cloving globally using npm:
npm install -g cloving@latest
Configuration:
Configure Cloving with your API key and preferred AI models. Use:
cloving config
Follow the prompts to input your API key and model preferences tailored to C++ development.
Initializing Your C++ Project:
Use the init
command to set up Cloving in your C++ project directory:
cloving init
This step ensures Cloving understands your project context, paving the way for effective code analysis and generation.
Utilizing Cloving for C++ Concurrency Enhancement
Generating Concurrency Code:
To enhance concurrency, Cloving can suggest code patterns and structures best suited for multi-threading and parallel execution in C++. Use the generate code
command with specific prompts.
For instance, suppose you’re implementing a parallel sort algorithm:
cloving generate code --prompt "Implement a parallel quicksort using C++ threads" --files src/sort.cpp
This command directs Cloving to utilize the context of existing files and generate a C++ parallel quicksort.
src/sort.cpp
:
Example Output in #include <iostream>
#include <vector>
#include <thread>
// Function to perform partitioning
int partition(std::vector<int> &arr, int low, int high) {
int pivot = arr[high];
int i = low - 1;
for (int j = low; j < high; ++j) {
if (arr[j] <= pivot) {
std::swap(arr[++i], arr[j]);
}
}
std::swap(arr[i + 1], arr[high]);
return i + 1;
}
// Recursive function for quicksort
void quicksort(std::vector<int> &arr, int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
std::thread t1(quicksort, std::ref(arr), low, pi - 1);
std::thread t2(quicksort, std::ref(arr), pi + 1, high);
t1.join();
t2.join();
}
}
The generated code leverages the standard library’s threading capabilities, demonstrating Cloving’s adeptness at multi-threaded solutions.
Reviewing for Performance Optimization:
Once concurrency is introduced, it’s vital to ensure the code remains optimal. Use Cloving’s generate review
command to conduct AI-powered code reviews:
cloving generate review -f src/sort.cpp
Cloving will provide feedback on potential bottlenecks or suggest alternative approaches to enhance parallel execution efficiency.
Interactive AI-Aided Development
Cloving Chat for Continuous Guidance:
For ongoing assistance or complex queries, utilize cloving chat
for an interactive dialog with AI:
cloving chat -f src/sort.cpp
Engage with the AI to gain insights or further refine your multi-threading implementation:
cloving> Can you suggest improvements to reduce thread overhead in the parallel quicksort?
The AI might recommend using thread pools or other concurrency frameworks to strike a balance between thread management and computational efficiency.
Generating and Managing Unit Tests
To ensure robustness, employing unit tests is crucial. Cloving aids in generating unit tests for your multi-threaded functions.
cloving generate unit-tests -f src/sort.cpp
src/sort.test.cpp
:
Example Output in #include <vector>
#include <cassert>
#include "sort.cpp"
void test_parallel_quicksort() {
std::vector<int> arr = {4, 3, 1, 5, 2};
quicksort(arr, 0, arr.size() - 1);
assert(std::is_sorted(arr.begin(), arr.end()));
std::cout << "Test passed!" << std::endl;
}
int main() {
test_parallel_quicksort();
return 0;
}
These tests help verify the correctness of the concurrent algorithms, catching potential race conditions or deadlocks early.
Conclusion
Harnessing the power of the Cloving CLI in your C++ workflows can drastically improve concurrency management and performance optimization. Whether you’re generating efficient parallel algorithms, reviewing code for performance bottlenecks, or implementing robust tests, Cloving streamlines processes by providing AI-driven insights and assistance. By integrating Cloving into your toolkit, you not only enhance your productivity but also deliver high-quality, performant C++ solutions.
Embrace the potential of AI with Cloving and transform your development experience today!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.