Leveraging AI for Intuitive Backend Architecture in Ruby on Rails
Updated on January 01, 2025

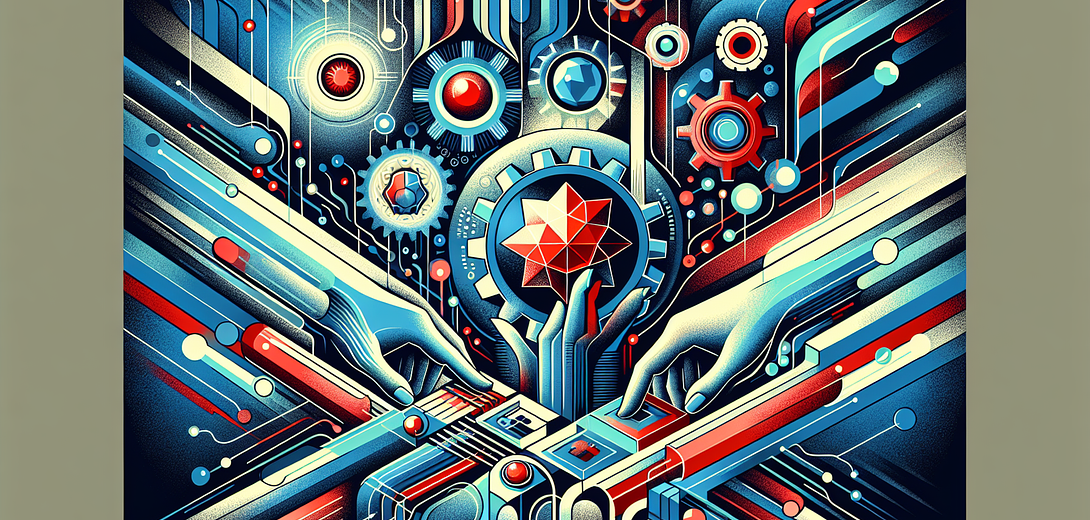
In the ever-evolving landscape of software development, leveraging AI to streamline and enhance coding practices is becoming increasingly essential. One such tool that can redefine your Ruby on Rails development workflow is the Cloving CLI. This AI-powered command-line interface offers innovative features that allow you to begin architecting your backend projects more intuitively and effectively.
In this post, we’ll focus on how to use Cloving to create a robust backend architecture for Ruby on Rails projects by showcasing practical use cases, tips, and best practices.
Introducing Cloving CLI
Cloving is a dynamic CLI tool that integrates AI capabilities directly into your command line, facilitating a seamless coding experience. It provides intuitive code generation, enabling efficient backend architecture, all while focusing on producing high-quality code.
1. Setting Up Cloving
Before diving into backend architecture, let’s get Cloving set up on your system.
Installation:
Install Cloving globally via npm:
npm install -g cloving@latest
Configuration:
To start using Cloving efficiently, configure it with your API key and preferred AI model:
cloving config
Follow the guidelines to set up your preferences.
2. Initializing Your Rails Project
To maximize Cloving’s capabilities, initialize it in your Rails project directory to allow it to analyze your project’s context effectively:
cd path/to/rails/project
cloving init
This command sets up Cloving with the necessary configuration to understand the context of your Ruby on Rails application.
3. Generating Models and Controllers
When building a Rails application, defining models and controllers is crucial. Cloving’s AI can assist by generating Ruby classes and modules based on your prompts.
Example:
Suppose you need a model and controller for a simple Article
resource:
cloving generate code --prompt "Create a Rails model for Article with attributes: title:string, content:text" --files app/models/article.rb
Example Output:
# app/models/article.rb
class Article < ApplicationRecord
validates :title, presence: true
validates :content, presence: true
end
Similarly, generate a controller:
cloving generate code --prompt "Create a Rails controller for handling Article instances" --files app/controllers/articles_controller.rb
Example Output:
# app/controllers/articles_controller.rb
class ArticlesController < ApplicationController
def index
@articles = Article.all
end
def show
@article = Article.find(params[:id])
end
def new
@article = Article.new
end
def create
@article = Article.new(article_params)
if @article.save
redirect_to @article
else
render 'new'
end
end
private
def article_params
params.require(:article).permit(:title, :content)
end
end
4. Generating Unit Tests
To ensure the quality and reliability of your Rails application, use Cloving to generate unit tests:
cloving generate unit-tests -f app/models/article.rb app/controllers/articles_controller.rb
Example Output:
# test/models/article_test.rb
require 'test_helper'
class ArticleTest < ActiveSupport::TestCase
test "should not save article without title" do
article = Article.new(content: 'Some content')
assert_not article.save, "Saved the article without a title"
end
test "should save article with valid attributes" do
article = Article.new(title: 'Valid Title', content: 'Some content')
assert article.save, "Could not save the article with valid attributes"
end
end
5. Collaboration with Cloving Chat
For more complex inquiries or guidance during development, interact directly with Cloving via its chat feature:
cloving chat -f app/models/article.rb
This feature allows you to ask questions, request code explanations, or gather suggestions for improvements in a collaborative session.
6. Crafting Insightful Commit Messages
Cloving quickly generates contextually accurate commit messages to enhance your version control management:
cloving commit
Example Output:
Add Article model and controller with index, show, new, and create methods
Conclusion
Harnessing the power of Cloving CLI in Ruby on Rails projects can greatly elevate your backend architecture by enabling AI-enhanced code generation and testing. It simplifies the coding tasks, allowing you to concentrate more on strategic application design while maintaining code integrity and quality.
By integrating Cloving into your workflow, you unlock the potential to enhance productivity and align with modern development practices efficiently. Start leveraging this tool for your Ruby on Rails applications to experience a transformative development process.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.