Leveraging AI for Efficient State Management in Vue.js
Updated on July 01, 2024

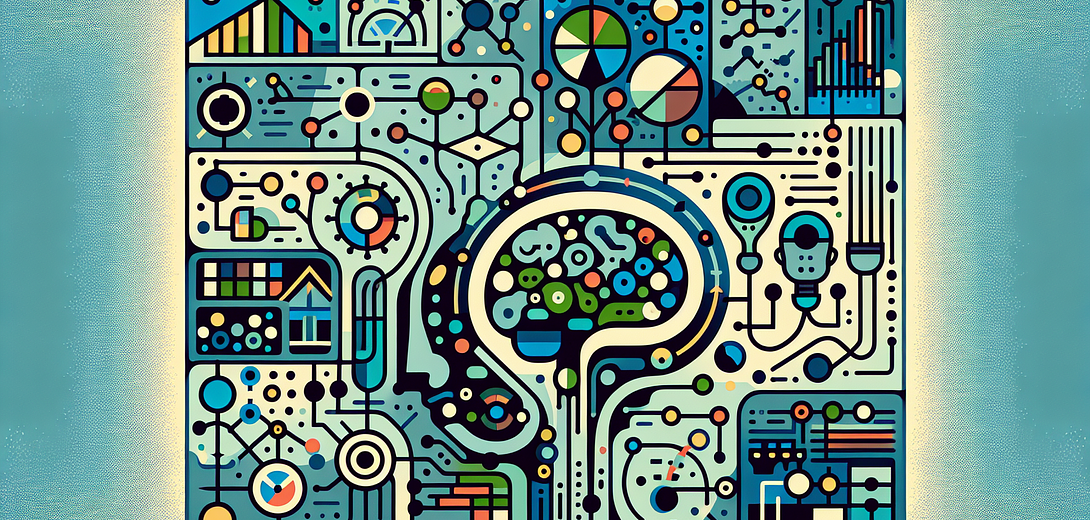
In the dynamic realm of front-end development, managing application state in complex Vue.js projects can become a daunting task. Integrating the principles of cloving—combining human creativity and intuition with the advanced processing capabilities of artificial intelligence (AI)—can significantly enhance your workflow and efficiency.
In this blog post, we’ll delve into how you can incorporate GPT into your daily Vue.js state management tasks using Pinia, making your development process smarter and more effective.
Understanding Cloving
Cloving, in this context, means leveraging AI to augment human skills in managing application state. By combining your intuition and creativity with GPT’s powerful analytical capabilities, you can tackle state management challenges more effectively.
Introduction to Pinia
Pinia is the official state management library for Vue.js, designed to be a simpler and more modern alternative to Vuex. Unlike Vuex, which frequently involves boilerplate code and can be complex to manage, Pinia offers a refined API, ensuring faster setup, better performance, and ease of use. This shift is part of the broader Vue.js ecosystem’s evolution, aiming to make state management more intuitive and efficient.
Pinia supports features like composition API, TypeScript integration, and automatic devtools support, making it ideal for complex applications requiring robust state management. Now, let’s explore how you can leverage AI to enhance your state management practices using Pinia.
1. Automated State Management Suggestions
AI can analyze your Vue.js code to provide optimized state management solutions, helping you sort out complex state interactions and dependencies.
Example:
Imagine you’re building a to-do list application with Pinia and you’ve run into a state mutation issue. You can describe the problem to GPT:
I have a Pinia store for my to-do list app, but the state mutations aren't reflected in the components. Here's the store code:
```javascript
import { defineStore } from 'pinia';
const useTodosStore = defineStore('todos', {
state: () => ({
todos: []
}),
actions: {
addTodo (todo) {
this.todos.push(todo);
}
}
});
How can I fix this to properly update the components?
GPT will analyze your code and might suggest ensuring reactivity or checking if your components are correctly watching the state changes:
Ensure your components are subscribed to the Pinia store and are reactive. Make sure you are accessing the store state properly in your components using computed properties or reactive references.
2. Code Generation and Completion
When dealing with complex state logic or new Pinia patterns, GPT can assist by generating and completing code snippets for you.
Example:
If you need to structure your store around specific modules and don’t know where to start, you can ask GPT:
Generate a Pinia module for handling user authentication state.
GPT will output a detailed module setup, such as:
import { defineStore } from 'pinia';
export const useAuthStore = defineStore('auth', {
state: () => ({
user: null,
token: null,
isAuthenticated: false
}),
actions: {
setUser(user) {
this.user = user;
this.isAuthenticated = !!user;
},
setToken(token) {
this.token = token;
},
login(user) {
// Simulate API call and commit mutation
this.setUser(user);
this.setToken(user.token);
},
logout() {
this.setUser(null);
this.setToken(null);
}
},
getters: {
isAuthenticated: state => state.isAuthenticated
}
});
3. Learning and Applying Best Practices
Staying updated with best practices for state management in Vue.js can be challenging. GPT can provide timely and relevant insights to help you adopt efficient patterns and avoid common pitfalls.
Example:
You can inquire about the latest trends and best practices:
What are the best practices for managing Vue.js state with Pinia in 2024?
GPT will provide you a list of tips, such as:
- Separate your Pinia stores into modules for better scalability.
- Use Pinia plugins for tasks like state persistence and logging.
- Prefer immutable state updates to maintain a predictable state.
- Implement proper error handling and loading states in your actions.
- Keep your actions simple and synchronous when possible.
4. Automated Test Generation
Writing and maintaining tests is crucial for ensuring the integrity of your state management but can be tedious. GPT can help automate the generation of test cases for Pinia actions and mutations.
Example:
If you’ve written a new Pinia action and need tests for it, you can prompt GPT:
Generate Jest tests for the login action in the auth Pinia store.
GPT will generate a set of test cases, such as:
import { createPinia, setActivePinia } from 'pinia';
import { useAuthStore } from '@/stores/auth';
describe('auth Pinia Store', () => {
beforeEach(() => {
setActivePinia(createPinia());
});
test('login action sets the user and token', async () => {
const authStore = useAuthStore();
const user = { name: 'Jane Doe', email: '[email protected]', token: 'abcd1234' };
authStore.login(user);
expect(authStore.isAuthenticated).toBe(true);
expect(authStore.user).toEqual(user);
expect(authStore.token).toEqual('abcd1234');
});
});
5. Documentation and Explanation
AI can assist in generating clear documentation for your Pinia store, making it easier for team members to understand and maintain the code.
Example:
You can request GPT to generate documentation for your store:
Generate documentation for the auth Pinia store.
GPT will produce detailed documentation:
## auth Pinia Store
### State
- `user`: Object - Holds the user data.
- `token`: String - Stores the authentication token.
- `isAuthenticated`: Boolean - Indicates whether the user is authenticated.
### Actions
- `setUser(user)`: Updates the user data and the authentication status.
- `setToken(token)`: Sets the authentication token.
- `login(user)`: Simulates an API call and commits the user mutation.
- `logout()`: Clears the user data and token.
### Getters
- `isAuthenticated`: Returns the authentication status.
Conclusion
Embracing AI for efficient state management in Vue.js with Pinia is a prime example of cloving, merging human ingenuity with machine precision. By incorporating GPT into your development workflow, you can enhance efficiency, minimize errors, and stay current with best practices. The synergistic combination of human and artificial intelligence can radically transform your programming experience and elevate your productivity.
Bonus Follow-Up Prompts
To further refine and streamline your development process, consider using these additional prompts:
How can I configure continuous integration to automatically test my Pinia store?
And another one:
Generate mock data for testing my Pinia store actions.
One more for further enhancement:
What are some more GPT prompts to help with managing application state in Vue.js?
Note:
Whenever you see [code snippet]
, replace it with an appropriate snippet in the programming language in question.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.