Leveraging AI for Efficient Modular Code Structure in Angular
Updated on March 05, 2025

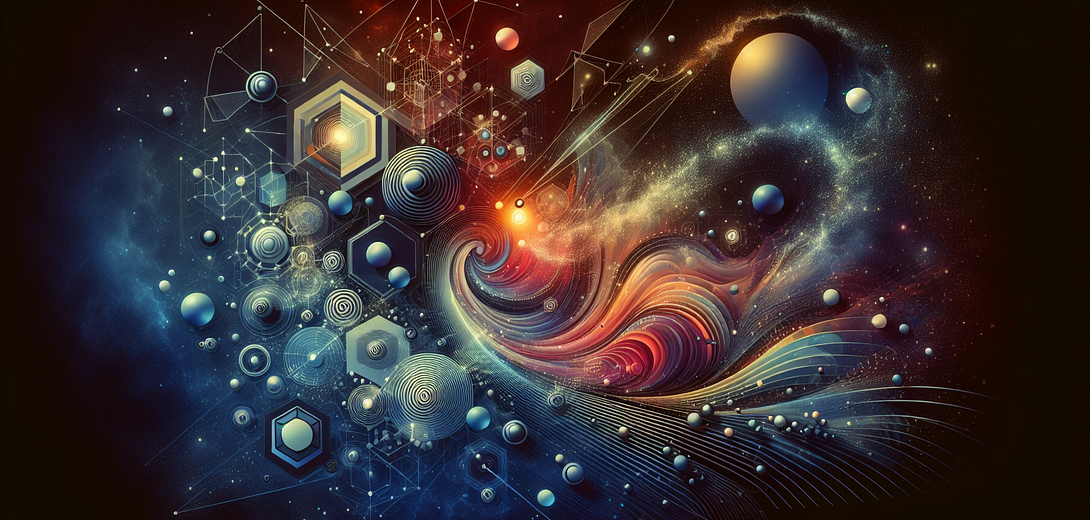
Enhancing Modular Angular Structures with Cloving CLI
Angular is a widely adopted framework for building dynamic, scalable web applications. A critical aspect of maintaining readability, reusability, and maintainability in Angular is implementing a modular code structure. Integrating AI into this process can further elevate your workflow and efficiency. In this post, we’ll explore how the Cloving CLI – an AI-powered command-line tool – can help you seamlessly automate, refine, and test your Angular code modules.
1. Getting Started with Cloving CLI
1.1 Installation and Configuration
- Install Cloving CLI globally via npm:
npm install -g cloving@latest
- Configure Cloving with your API key and chosen AI model:
cloving config
- Follow the prompts to input your API key and select the AI model that best suits your project.
1.2 Initializing Your Angular Project
Let Cloving gather context by initializing it in your project’s root directory:
cloving init
This creates a cloving.json
file that stores project metadata and context details, enabling Cloving to generate accurate, project-specific code.
2. Automating Angular Module Development
Cloving CLI excels at generating boilerplate for Angular components, services, directives, and more. This automation frees you to focus on core logic and architecture.
2.1 Example: Generating an Angular Service
Suppose you need a new service for handling HTTP requests related to a product catalog:
cloving generate code --prompt "Create an Angular service to manage HTTP requests for a product catalog" --files src/app/services/product.service.ts
Sample Output:
// src/app/services/product.service.ts
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class ProductService {
private baseUrl = 'https://api.example.com/products';
constructor(private http: HttpClient) {}
getProducts(): Observable<Product[]> {
return this.http.get<Product[]>(this.baseUrl);
}
}
Notes:
- Cloving identifies typical Angular patterns, e.g., using
HttpClient
. - You can refine the code (e.g., adding other CRUD methods) through Cloving’s interactive chat or direct edits.
2.2 Example: Creating a Custom Angular Directive
Directives encapsulate reusable behavior. Here’s how to generate a highlight directive:
cloving generate code --prompt "Create a custom Angular directive for highlighting table rows" --files src/app/directives/highlight.directive.ts
Sample Output:
// src/app/directives/highlight.directive.ts
import { Directive, ElementRef, HostListener } from '@angular/core';
@Directive({
selector: '[appHighlight]'
})
export class HighlightDirective {
constructor(private el: ElementRef) { }
@HostListener('mouseenter') onMouseEnter() {
this.highlight('yellow');
}
@HostListener('mouseleave') onMouseLeave() {
this.highlight(null);
}
private highlight(color: string) {
this.el.nativeElement.style.backgroundColor = color;
}
}
Notes:
- Cloving chooses sensible defaults (e.g.,
'yellow'
as the highlight color). You can adjust or refine further.
3. Refine and Interact with Cloving Chat
For complex or interactive coding tasks, Cloving’s chat feature is invaluable:
cloving chat -f src/app/components/product-list.component.ts
Engage in a two-way conversation with the AI. Examples:
- Ask for performance optimizations.
- Explore advanced module bundling strategies.
- Request code modifications or explanations in real time.
4. Enhancing Code Quality with Unit Tests
Maintaining a modular architecture includes thorough testing to ensure each piece works independently and seamlessly with the rest of the application.
4.1 Generating Unit Tests
Ask Cloving to create test stubs for your newly generated Angular service:
cloving generate unit-tests -f src/app/services/product.service.ts
Sample Output:
// src/app/services/product.service.spec.ts
import { TestBed } from '@angular/core/testing';
import { HttpClientTestingModule, HttpTestingController } from '@angular/common/http/testing';
import { ProductService } from './product.service';
describe('ProductService', () => {
let service: ProductService;
let httpMock: HttpTestingController;
beforeEach(() => {
TestBed.configureTestingModule({
imports: [HttpClientTestingModule],
providers: [ProductService]
});
service = TestBed.inject(ProductService);
httpMock = TestBed.inject(HttpTestingController);
});
it('should retrieve products from the API via GET', () => {
const dummyProducts = [{ id: 1, name: 'Product A' }, { id: 2, name: 'Product B' }];
service.getProducts().subscribe(products => {
expect(products.length).toBe(2);
expect(products).toEqual(dummyProducts);
});
const request = httpMock.expectOne(`${service.baseUrl}`);
expect(request.request.method).toBe('GET');
request.flush(dummyProducts);
});
});
Notes:
- Cloving detects Angular’s HttpClientTestingModule usage.
- You can expand these tests to cover different scenarios or error handling.
5. Streamlining Commit Messages
Frequent commits are part of a modular development process. Let Cloving produce contextual commit messages:
cloving commit
Cloving inspects your staged changes and proposes a succinct commit message, e.g.:
Add product service for handling HTTP requests with basic tests
You can accept or modify it, keeping your commit history concise and informative.
6. Best Practices for Modular Angular Code with Cloving
-
Contextual Prompts
Provide relevant file paths or mention existing code structure to get the most accurate code generation. -
Incremental Development
Generate one service or component at a time, then refine via chat or direct edits. This ensures each piece integrates well with the rest. -
Refine Generated Code
AI-generated code is a starting point. Combine it with your domain knowledge to finalize logic, naming, and architectural decisions. -
Leverage Official Angular Practices
While Cloving helps with boilerplate, continue using best practices like modules per feature, lazy loading, and shared modules for commonly used components/directives. -
Testing Coverage
Cloving’s generated tests are a baseline. Add more scenarios and negative tests to strengthen code reliability. -
Keep an Eye on Performance
Angular apps with multiple modules can get large. Monitor bundle sizes, and adopt strategies like lazy loading or tree shaking.
7. Conclusion
Using Cloving CLI for Angular development significantly streamlines your workflow by automating routine tasks and fostering a more modular code structure. You can generate new services, directives, and components in seconds, engage in interactive code refinement, and ensure quality with automatically generated tests.
Cloving complements your expertise, allowing you to focus on core design and business logic rather than repetitive boilerplate. As you integrate it into your daily Angular workflow, you’ll discover a newfound agility and consistency in architecting your application’s modules and features.
Try Cloving CLI today, and elevate your Angular coding experience through the synergy of AI and thoughtful modular design!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.