Leveraging AI for Advanced TypeScript Code Generation in SPAs
Updated on March 30, 2025

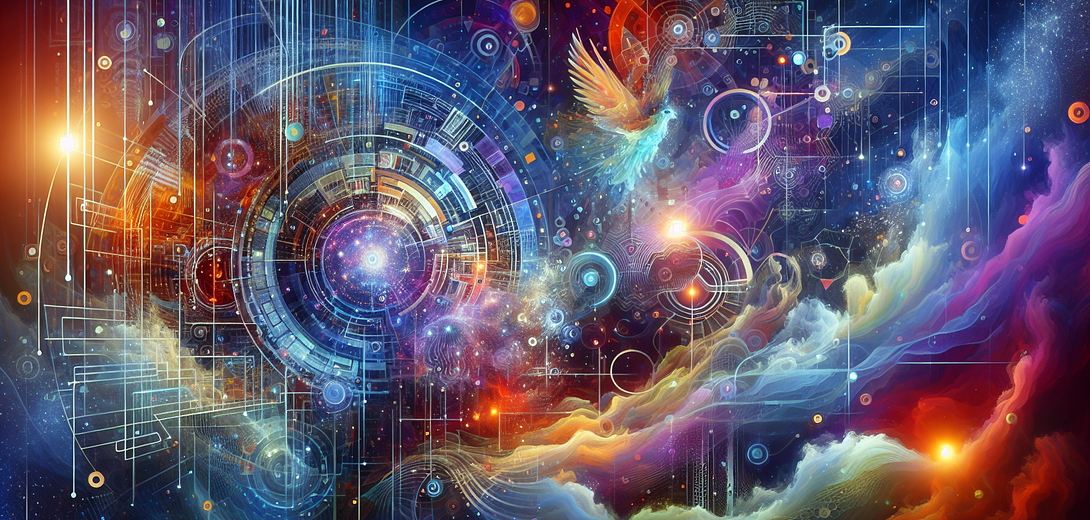
Accelerating TypeScript SPA Development with Cloving CLI
TypeScript has emerged as a vital tool for building Single Page Applications (SPAs), offering static typing and robust tooling. Yet, as your codebase grows, generating, refining, and testing components can become laborious. Cloving CLI, an AI-powered command-line interface, introduces an efficient way to automate these tasks using GPT-based intelligence. In this post, we’ll explore how to integrate Cloving into your TypeScript workflow for faster, more reliable SPAs.
1. Setting Up Cloving for TypeScript
1.1 Installation
Install Cloving globally via npm:
npm install -g cloving@latest
1.2 Configuration
Next, configure Cloving by providing your API key and AI model:
cloving config
Follow the interactive prompts (e.g., selecting GPT-3.5 or GPT-4). This step ensures Cloving can connect to the AI backend and generate code or tests for your TypeScript project.
1.3 Initialization
Within your SPA project root—where you have package.json
or your src/
folder—run:
cloving init
Cloving creates a cloving.json
file with context about your application, enabling more tailored code generation (e.g., React vs. Vue, existing folder structure).
2. Generating TypeScript Components
2.1 Example: User Registration Form
Imagine you need a TypeScript React component for a user registration form. Let Cloving handle the boilerplate:
cloving generate code --prompt "Create a TypeScript React component for a user registration form" --files src/components/UserForm.tsx
Sample Output:
// src/components/UserForm.tsx
import React, { useState } from 'react';
interface User {
username: string;
email: string;
password: string;
}
const UserForm: React.FC = () => {
const [user, setUser] = useState<User>({ username: '', email: '', password: '' });
const handleChange = (e: React.ChangeEvent<HTMLInputElement>) => {
const { name, value } = e.target;
setUser((prevUser) => ({ ...prevUser, [name]: value }));
};
const handleSubmit = (e: React.FormEvent) => {
e.preventDefault();
console.log(user);
};
return (
<form onSubmit={handleSubmit}>
<input name="username" value={user.username} onChange={handleChange} placeholder="Username" />
<input name="email" value={user.email} onChange={handleChange} placeholder="Email" />
<input name="password" type="password" value={user.password} onChange={handleChange} placeholder="Password" />
<button type="submit">Register</button>
</form>
);
};
export default UserForm;
Notes:
- Cloving automatically provides typed props or state.
- If your app uses frameworks like Vue or a different structure, mention them in your prompt.
2.2 Additional Example: Angular or Vue
If your SPA uses Angular or Vue, reference these in the prompt (e.g., “Create a TypeScript Angular component for user registration”), and Cloving will produce the relevant code snippet aligned with that framework’s structure.
3. Refining Code through Interactive Sessions
3.1 Interactive Refinement
After generating a baseline component, you might want advanced validation or specialized logic. Start an interactive session:
cloving chat -f src/components/UserForm.tsx
In the chat, you can ask for expansions, e.g.:
cloving> Add validation logic for password strength and email format
Cloving will propose updated code with additional checks or a third-party library if you mention it.
4. Generating Unit Tests
4.1 Testing Example: React with Jest
To ensure your new component is robust, let Cloving generate test scaffolding:
cloving generate unit-tests -f src/components/UserForm.tsx
Sample Output:
// src/components/UserForm.test.tsx
import React from 'react';
import { render, fireEvent } from '@testing-library/react';
import UserForm from './UserForm';
describe('UserForm', () => {
it('should handle user registration', () => {
const { getByPlaceholderText, getByText } = render(<UserForm />);
fireEvent.change(getByPlaceholderText('Username'), { target: { value: 'testuser' } });
fireEvent.change(getByPlaceholderText('Email'), { target: { value: '[email protected]' } });
fireEvent.change(getByPlaceholderText('Password'), { target: { value: 'password123' } });
fireEvent.click(getByText('Register'));
// Check if console.log or submission logic was triggered
});
});
Notes:
- Cloving references typical React testing patterns with Jest.
- If your code uses Cypress or a different framework, mention that in your prompt.
4.2 Additional Example: Testing Utility Functions
If you have a utils.ts
file, you can instruct Cloving to produce or update tests for your utility methods:
cloving generate unit-tests -f src/utils.ts
5. Optimizing Git Workflow
5.1 Generating Commit Messages
After updating or adding new code, you can skip writing commit messages from scratch:
cloving commit
Cloving reviews your staged changes, proposing a summary (e.g., “Add user registration form component and initial test coverage”). You can accept or refine as needed.
6. Advanced Usage and Tips
- Contextual Project Initialization
Always runcloving init
so the AI can read your tsconfig.json, package.json, or references to frameworks (React, Vue, Angular). - Iterate
For advanced tasks (like API integration, state management with Redux or Vuex, or hooking into your Angular services), use multiple prompts or the interactive chat. - Review
If your app is complex, occasionally runcloving generate review
to get a high-level scan for potential improvements or missing best practices. - Leverage
generate shell
If you also have scripts or deployment steps, mention them in your prompt. Cloving can generate bash scripts for tasks like linting, building, or testing across multiple packages. - Maintain
Cloving’s code output is a foundation—always ensure final correctness, domain specificity, and performance constraints align with your project’s needs.
7. Conclusion
Cloving CLI merges TypeScript with AI to streamline code creation, test generation, and iterative improvements in single-page application environments. From building a React component to refining a complex Vue or Angular module, Cloving handles the repetitive tasks, letting you focus on design, performance, and user experience.
Remember, while Cloving significantly reduces boilerplate and manual coding, your domain knowledge and final review ensure the result fits your architecture, style, and performance goals. Embrace Cloving for TypeScript SPAs, and discover a more efficient and AI-augmented development process.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.