Leveraging AI-Driven Techniques for Efficient JavaScript Promise Management
Updated on March 05, 2025

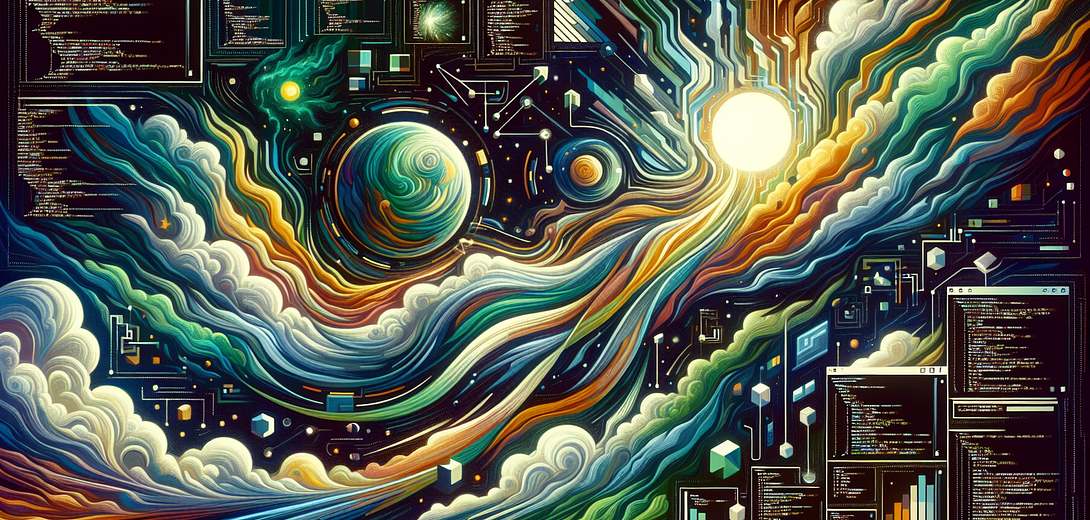
Streamlining JavaScript Promise Management with Cloving CLI
Asynchronous programming in JavaScript allows you to handle tasks like API calls, file I/O, and other time-consuming operations without blocking the main thread. While promises (and async/await) greatly simplify the asynchronous workflow, effectively managing complex promise chains or robust error handling can be challenging. Cloving CLI, an AI-powered command-line interface, can assist by generating, refactoring, and testing promise-based JavaScript code, ensuring your applications maintain reliability and clarity.
In this post, we’ll walk through how Cloving CLI can elevate your JavaScript promise handling with step-by-step instructions and best practices.
1. Setting Up Cloving CLI
Before leveraging AI for JavaScript promise management, make sure Cloving CLI is correctly installed and configured.
1.1 Installation
Install Cloving globally via npm:
npm install -g cloving@latest
1.2 Configuration
Configure Cloving with your API key and preferred model:
cloving config
Follow the interactive prompts to finalize your setup. Once complete, Cloving is ready to seamlessly integrate into your coding environment.
2. Initializing Your JavaScript Project
To enable Cloving’s AI insights, initialize it in the root directory of your JavaScript project:
cloving init
This command creates a cloving.json
file that stores metadata about your project, allowing Cloving to better understand its context and tailor suggestions accordingly.
3. Generating Code for Promise Management
3.1 Example: Refactoring for Error Handling
Let’s say you have a function that fetches data from an external API but lacks robust error handling. By using Cloving’s generate code
command, you can prompt it to refactor your existing code:
cloving generate code --prompt "Refactor this function to properly handle promises using try-catch" --files utils/apiUtils.js
Cloving will take the existing code in utils/apiUtils.js
and refactor it, outputting something like:
// utils/apiUtils.js
export async function fetchData(url) {
try {
const response = await fetch(url);
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
const data = await response.json();
return data;
} catch (error) {
console.error('Failed to fetch data', error);
return null;
}
}
Key Benefits:
- Readability: Async/await plus try/catch structure is cleaner and easier to follow.
- Reliability: Any HTTP or parsing errors are caught and logged.
- Fallback: Returning
null
(or a sentinel value) allows your application to handle errors gracefully.
4. Interactive Chat for Real-Time Assistance
Complex promise workflows often require iterative improvements, such as optimizing performance or chaining multiple asynchronous calls in sequence or parallel. Cloving’s interactive chat provides real-time assistance:
cloving chat -f src/app.js
Here, you might say:
Optimize API call chaining using promises and handle any edge cases.
Cloving might suggest converting a promise chain into Promise.all (for parallel execution) or async/await with sequential execution, pointing out potential pitfalls or logging strategies.
4.1 Example: Parallelizing Multiple API Calls
You can use the chat to request a snippet that fetches data from two APIs in parallel:
cloving> Show me how to fetch data from two APIs in parallel and handle any errors.
Cloving may output:
const [users, posts] = await Promise.all([
fetchUsers().catch(err => {
console.error('Failed to fetch users', err);
return [];
}),
fetchPosts().catch(err => {
console.error('Failed to fetch posts', err);
return [];
})
]);
This pattern ensures your application continues gracefully, even if one of the calls fails, with all relevant error handling in place.
5. Generating Unit Tests for Promise-Based Code
Ensuring your asynchronous logic is correct means putting it under test. Cloving helps generate tests that mimic real-world async scenarios.
cloving generate unit-tests -f src/utils/apiUtils.js
Cloving might produce apiUtils.test.js
resembling this:
// src/utils/apiUtils.test.js
import { fetchData } from './apiUtils';
describe('fetchData', () => {
it('returns data when the fetch is successful', async () => {
global.fetch = jest.fn(() =>
Promise.resolve({
ok: true,
json: () => Promise.resolve({ key: 'value' })
})
);
const data = await fetchData('https://api.example.com/data');
expect(data).toEqual({ key: 'value' });
});
it('handles fetch errors gracefully', async () => {
global.fetch = jest.fn(() =>
Promise.resolve({
ok: false,
status: 404
})
);
const data = await fetchData('https://api.example.com/data');
expect(data).toBeNull();
});
});
What this does:
- Mocks the global
fetch
function to simulate successful and failing network requests. - Verifies that the function returns valid data in success scenarios and gracefully handles errors.
6. Best Practices for Promise Management
To further enhance your asynchronous code, keep in mind these best practices:
- Leverage Async/Await: It generally yields cleaner and more readable code than raw
then/catch
chains. - Handle Exceptions Thoroughly: Include fallback logic or default values in case calls fail.
- Use Parallelization Wisely: Decide between sequential calls vs. parallel calls (via
Promise.all
) based on dependencies between tasks. - Add Timeouts: In critical applications, consider implementing request timeouts to prevent indefinite waits.
- Graceful Degradation: If one call fails, be prepared to handle partial success or fallback to cached data.
7. Commit with AI-Powered Descriptions
Maintaining a clear version history is crucial. Let Cloving’s AI craft commit messages that reflect the nature of your changes:
cloving commit
You’ll receive a context-sensitive commit message proposal, such as:
Refactor fetchData function for better error handling and add Jest tests for multiple API scenarios
Feel free to tweak the message before confirming the commit. This ensures your team or future self understands what changed and why.
8. Additional Use Cases for Cloving CLI
While this post focuses on promise management in JavaScript, Cloving can assist in many other areas:
- Refactoring: Transform older code from
then/catch
toasync/await
, standardizing your codebase. - Performance Tuning: Optimize hotspots by suggesting parallelization strategies or caching approaches.
- Error Logging: Add logging hooks or integrate monitoring libraries easily.
- Architecture Consulting: Use Cloving’s chat to discuss advanced patterns like circuit breakers or message queues.
9. Conclusion
By seamlessly integrating Cloving CLI into your development workflow, you can dramatically enhance the way you manage JavaScript promises. From code generation and refactoring to interactive optimization and comprehensive testing, Cloving helps ensure that your asynchronous operations remain robust and maintainable.
Remember: While Cloving provides a powerful AI-driven boost, your domain knowledge and expertise guide the best use of these suggestions. Combine Cloving’s capabilities with your experience for clean, efficient, and reliable asynchronous JavaScript code.
Master these AI-driven techniques with Cloving CLI, and see how much simpler promise management and error handling can become in your next project!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.