Integrating Redux into Next.js with AI Assistance
Updated on June 26, 2024

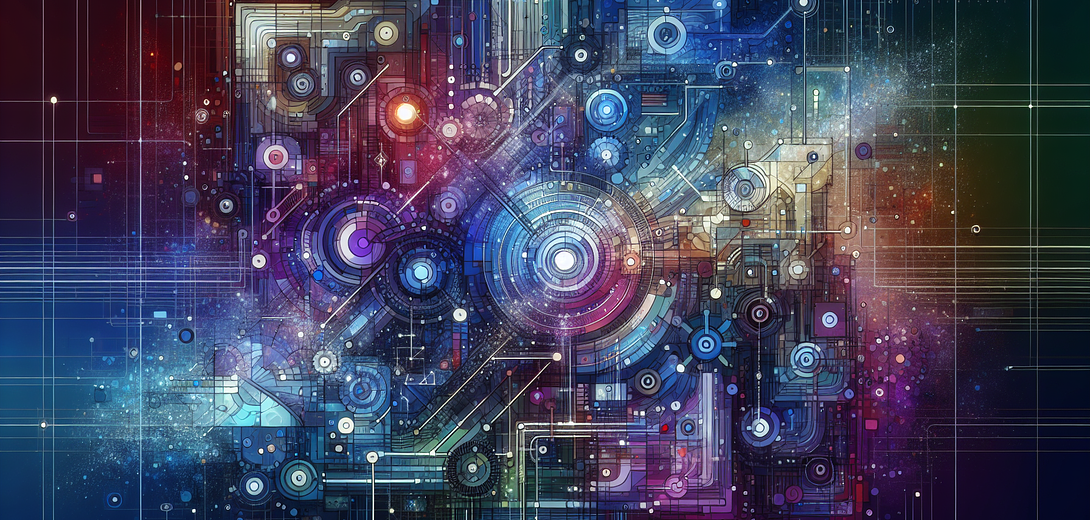
In the dynamic world of front-end development, managing state in complex applications can often be a challenging task.
Redux, a predictable state container for JavaScript apps, has become an essential tool to help developers manage state in a more organized and efficient manner.
But integrating Redux into a Next.js application can be daunting for some. This is where the principles of cloving come in—combining human creativity and intuition with the powerful capabilities of artificial intelligence (AI).
In this post, we’ll explore how AI tools like GPT can assist in integrating Redux into your Next.js applications, making your programming tasks more efficient and effective.
Understanding Cloving
Cloving combines human intuition and creativity with AI’s analytical prowess to achieve common goals. It’s not just about using AI tools; it’s about creating a symbiotic relationship where human and machine strengths are leveraged to solve problems more effectively.
1. Setting Up Redux in Next.js
Setting up Redux in a Next.js application requires a few steps, including installing necessary packages and configuring your store. GPT can simplify this process by providing you with a step-by-step setup guide.
Example:
You can start by requesting GPT for a guide to set up Redux in a Next.js application:
Provide a step-by-step guide to set up Redux in a Next.js application.
GPT will likely respond with something like this:
-
Install necessary packages:
npm install redux react-redux next-redux-wrapper
-
Create the Redux Store:
// store.js import { createStore } from 'redux'; import rootReducer from './reducers'; const store = createStore(rootReducer); export default store;
-
Create a Next.js
_app.js
file to integrate Redux:// pages/_app.js import { Provider } from 'react-redux'; import store from '../store'; const MyApp = ({ Component, pageProps }) => { return ( <Provider store={store}> <Component {...pageProps} /> </Provider> ); }; export default MyApp;
2. Creating Reducers and Actions
Reducers and actions are the heart of Redux. GPT can assist you by generating boilerplate code for reducers and actions, helping you maintain consistency and efficiency.
Example:
You need to create actions and reducers for managing user authentication state. You can prompt GPT:
Generate Redux actions and reducers for managing user authentication state.
GPT will generate something like:
Actions:
// actions/authActions.js
export const LOGIN_REQUEST = 'LOGIN_REQUEST';
export const LOGIN_SUCCESS = 'LOGIN_SUCCESS';
export const LOGIN_FAILURE = 'LOGIN_FAILURE';
export const LOGOUT = 'LOGOUT';
export const loginRequest = () => ({
type: LOGIN_REQUEST,
});
export const loginSuccess = (user) => ({
type: LOGIN_SUCCESS,
payload: user,
});
export const loginFailure = (error) => ({
type: LOGIN_FAILURE,
payload: error,
});
export const logout = () => ({
type: LOGOUT,
});
Reducers:
// reducers/authReducer.js
import {
LOGIN_REQUEST,
LOGIN_SUCCESS,
LOGIN_FAILURE,
LOGOUT
} from '../actions/authActions';
const initialState = {
isAuthenticated: false,
user: {},
loading: false,
error: null,
};
const authReducer = (state = initialState, action) => {
switch (action.type) {
case LOGIN_REQUEST:
return { ...state, loading: true, error: null };
case LOGIN_SUCCESS:
return { ...state, isAuthenticated: true, user: action.payload, loading: false };
case LOGIN_FAILURE:
return { ...state, loading: false, error: action.payload };
case LOGOUT:
return { ...state, isAuthenticated: false, user: {} };
default:
return state;
}
};
export default authReducer;
3. Unit Testing with AI Assistance
Testing is a crucial part of ensuring your Redux integration functions as expected. GPT can help by generating Jest or any other testing framework test cases for your actions and reducers.
Example:
Ask GPT for test cases for the previously generated authReducer
.
Generate Jest test cases for the authReducer in my Next.js application.
GPT might suggest:
// tests/reducers/authReducer.test.js
import authReducer from '../../reducers/authReducer';
import {
LOGIN_REQUEST,
LOGIN_SUCCESS,
LOGIN_FAILURE,
LOGOUT
} from '../../actions/authActions';
const initialState = {
isAuthenticated: false,
user: {},
loading: false,
error: null,
};
describe('authReducer', () => {
it('should return the initial state', () => {
expect(authReducer(undefined, {})).toEqual(initialState);
});
it('should handle LOGIN_REQUEST', () => {
expect(authReducer(initialState, { type: LOGIN_REQUEST })).toEqual({
...initialState,
loading: true,
error: null,
});
});
// .... More test cases for other actions
});
4. Documentation and Explanation
Understanding and documenting complex state management logic can be challenging. GPT can assist by generating clear documentation for your Redux code.
Example:
When you need to document the authentication flow, you can ask GPT:
Generate documentation for the authentication flow using Redux actions and reducers in my Next.js application.
GPT will create detailed documentation, helping you and your team maintain clarity and consistency.
## Authentication Flow Documentation
### Action Creators
- **loginRequest**: Initiates a login request.
```javascript
loginRequest();
-
loginSuccess: Dispatched when login is successful and receives user data.
loginSuccess(user);
-
loginFailure: Dispatched when login fails and receives an error message.
loginFailure(error);
-
logout: Clears the user state and logs out the user.
logout();
Reducer: authReducer
-
Initial State:
const initialState = { isAuthenticated: false, user: {}, loading: false, error: null, };
-
State Transitions:
- LOGIN_REQUEST: Sets loading to true and clears error.
- LOGIN_SUCCESS: Sets isAuthenticated to true, stores user data, and clears loading.
- LOGIN_FAILURE: Sets error message and clears loading.
- LOGOUT: Clears user data and sets isAuthenticated to false.
## Conclusion
Integrating Redux into a Next.js application is a fundamental step in managing state efficiently. By leveraging the principles of cloving—combining human intuition and creativity with AI's analytical capabilities—you can enhance your productivity, reduce errors, and keep your codebase maintainable.
Embrace AI assistance and discover how this synergistic approach can simplify your development workflow. Happy coding!
## Bonus Follow-Up Prompts
Here are a few extra bonus prompts you could use to refine the prompts you see above:
```plaintext
How can I configure GitHub Actions to run these tests automatically for me?
And here is another:
Generate accompanying dummy data or factories for testing purposes.
And one more:
What are other GPT prompts I could use to make this more efficient?
Integrating Redux in your Next.js applications can be a smoother experience when you use the power of AI to assist you. Enjoy the seamless coding journey!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.