Integrating Jotai with Next.js Using GPT
Updated on June 26, 2024

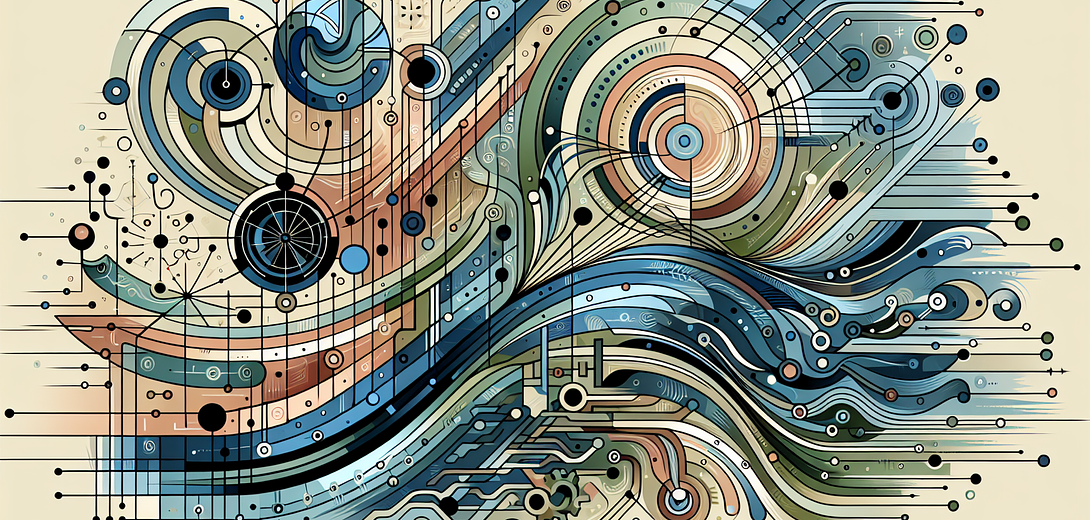
In the ever-evolving landscape of web development, integrating state management libraries like Jotai with frameworks such as Next.js can be both exciting and challenging.
By adopting the principles of cloving—blending human creativity and intuition with the processing capabilities of artificial intelligence (AI)—programmers can streamline this integration process, making their workflows more efficient and effective.
In this blog post, we’ll explore how to integrate Jotai with Next.js using GPT, providing practical examples, tips, and best practices to help you enhance your development workflow.
Understanding Cloving
Cloving is all about the harmonious integration of human ingenuity and AI’s computational strengths to achieve shared objectives. It’s not merely about deploying AI tools; it involves establishing a symbiotic relationship where human and machine abilities are leveraged to solve problems more efficiently.
1. Setting Up Your Next.js Project with Jotai
Before diving into more complex tasks, let’s set up a basic Next.js project with Jotai. With the help of GPT, you can quickly get started by generating the necessary configuration and initial setup code.
Example:
You can ask GPT for the initial setup instructions:
How do I integrate Jotai into a new Next.js project? Provide the necessary steps and code snippets.
GPT might suggest the following steps:
# Create a new Next.js project
npx create-next-app my-next-jotai-app
# Navigate to the project directory
cd my-next-jotai-app
# Install Jotai
npm install jotai
And provide the starting code in your Next.js project:
In pages/_app.js
:
import { Provider } from 'jotai';
import '../styles/globals.css';
function MyApp({ Component, pageProps }) {
return (
<Provider>
<Component {...pageProps} />
</Provider>
);
}
export default MyApp;
2. Creating and Managing State Atoms
Jotai uses atoms to manage state. GPT can help you create and manage these atoms efficiently.
Example:
You can ask GPT to generate a basic state management setup:
How do I create a state atom in Jotai for managing a count variable?
GPT will provide a concise example:
// atoms/counterAtom.js
import { atom } from 'jotai';
export const counterAtom = atom(0);
3. Connecting Atoms to Your Components
Connecting Jotai state atoms to your components allows for reactive state management. GPT can assist in writing this boilerplate code for you.
Example:
Ask GPT how to use the counterAtom in a Next.js component:
How can I use the counterAtom in a Next.js page to display and update the count?
GPT will generate a usage example:
// pages/index.js
import { useAtom } from 'jotai';
import { counterAtom } from '../atoms/counterAtom';
export default function Home() {
const [count, setCount] = useAtom(counterAtom);
return (
<div>
<h1>Count: {count}</h1>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
4. Generating Complex State Logic
Managing more complex state logic can be simplified with GPT’s assistance. Suppose you need to add a derived atom that computes a value based on the counterAtom
.
Example:
Prompt GPT to create a derived atom:
Generate a derived atom for counterAtom that calculates double the count.
GPT will provide:
// atoms/doubleCounterAtom.js
import { atom } from 'jotai';
import { counterAtom } from './counterAtom';
export const doubleCounterAtom = atom((get) => get(counterAtom) * 2);
5. Maintaining Best Practices
Keeping up with best practices ensures your code remains maintainable and scalable. GPT can offer insights and recommendations on best practices with Jotai and Next.js.
Example:
To get best practices for integrating Jotai with Next.js, you can ask:
What are the best practices for integrating Jotai with Next.js in 2024?
GPT might list important points such as:
- Splitting state management logic across separate atom files.
- Utilizing derived atoms for complex state computations.
- Keeping atomic state as minimal as possible.
- Using TypeScript with Jotai for better type safety.
6. Documentation and Explanation
Understanding and documenting your code can be time-consuming, but GPT can help generate clear explanations and documentation.
Example:
Ask GPT to generate documentation for your counter atom setup:
Generate documentation for the counterAtom and doubleCounterAtom setup.
GPT will generate:
### Counter Atom
The `counterAtom` is a simple Jotai atom that holds a numeric state representing the count.
```javascript
import { atom } from 'jotai';
export const counterAtom = atom(0);
Double Counter Atom
The doubleCounterAtom
is a derived Jotai atom that computes double the value of counterAtom
.
import { atom } from 'jotai';
import { counterAtom } from './counterAtom';
export const doubleCounterAtom = atom((get) => get(counterAtom) * 2);
This documentation provides clarity for future reference and for other team members.
## Conclusion
Integrating Jotai with Next.js using GPT exemplifies the power of cloving—blending human creativity and intuition with AI's analytical capabilities. By incorporating GPT into your workflow, you can streamline the integration process, enhance productivity, and stay up-to-date with best practices. Embrace cloving and discover how this synergistic approach can transform your programming experience.
## Bonus Follow-Up Prompts
Here are a few extra bonus prompts you could use to refine the integration further:
```plaintext
How can I configure GitHub Actions to run tests automatically for my Next.js and Jotai project?
Generate example data for testing Jotai atoms and their derived states.
What are other GPT prompts I could use to make my Jotai state management more efficient?
By leveraging these prompts, you can continue to refine and enhance your integration process, making your development workflow even more efficient. Happy coding!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.