Integrating GPT for Agile Frontend Development with TypeScript
Updated on November 28, 2024

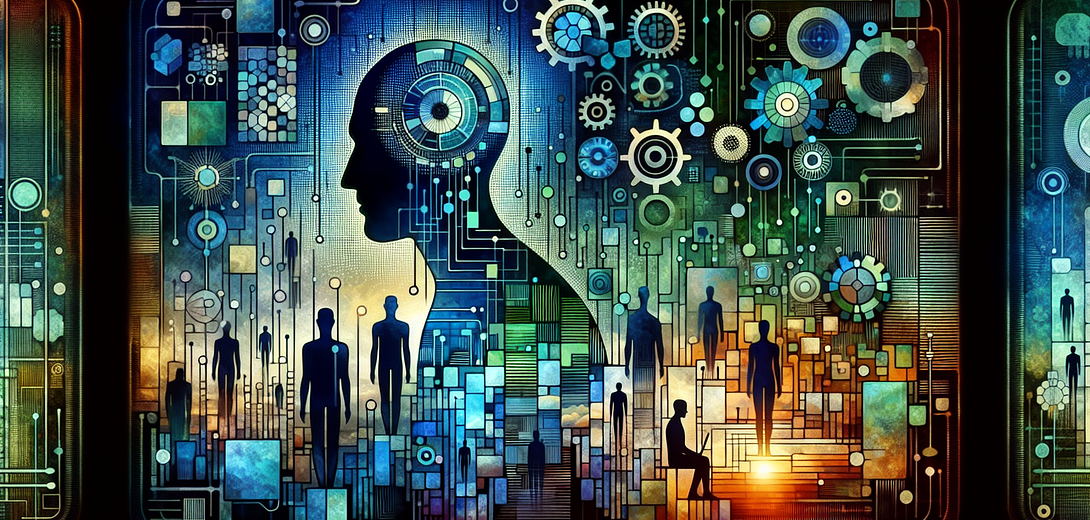
In the realm of agile development, the ability to iterate quickly and deliver high-quality features is crucial. The Cloving CLI tool enables frontend developers to harness the power of GPT-powered AI to enhance productivity, especially when working on TypeScript projects. In this blog post, we’ll explore how to integrate Cloving into your agile frontend development workflow to streamline tasks, improve code quality, and boost your team’s productivity.
Setting Up Cloving
Before diving into development tasks, let’s ensure that Cloving is properly set up in your environment.
Installation
First, install Cloving globally using npm:
npm install -g cloving@latest
Configuration
Next, configure Cloving with your API key and preferred AI model for a personalized experience:
cloving config
Follow the prompts to input your API key and choose the model that suits your project’s requirements.
Initializing Your Project with Cloving
To leverage Cloving’s capabilities, initialize it in your project directory. This step allows Cloving to understand your project’s context, which is especially important for TypeScript projects:
cloving init
This command will analyze your project and create a cloving.json
file, which contains context and metadata about your application.
Enhancing Code Generation with Cloving
One of the key features of the Cloving CLI is its ability to generate code snippets that fit perfectly into your existing project. Let’s see how you can use this feature in your TypeScript frontend development workflow.
Example: Creating a TypeScript Component
Imagine you need to create a reusable button component in your TypeScript application. You can use Cloving’s code generation capabilities as follows:
cloving generate code --prompt "Create a reusable button component in TypeScript" --files src/components/Button.tsx
Cloving will analyze your project context and generate a code snippet similar to this:
// src/components/Button.tsx
import React from 'react';
interface ButtonProps {
label: string;
onClick: () => void;
}
const Button: React.FC<ButtonProps> = ({ label, onClick }) => {
return (
<button onClick={onClick}>
{label}
</button>
);
};
export default Button;
This generated code is ready to use and tailored to fit the style and structure of your existing TypeScript components.
Streamlining Development with Cloving Chat
For complex tasks or continuous interaction, Cloving’s chat feature provides an AI-assisted chat environment. Start a chat session as follows:
cloving chat -f src/components/App.tsx
In this session, you can ask questions, get explanations, or request code changes. The chat can assist with tasks such as refactoring, bug fixing, or implementing features.
Example Chat Interaction
cloving> How can I refactor my Button component to improve its performance?
Certainly! One way to improve performance is by memoizing the component using React.memo. Here's an updated version of your Button component:
...
The ability to tailor your chat session to a particular context within your app, or even to your entire app, is the power of the Cloving CLI.
Generating Unit Tests
Ensuring the reliability of your code is critical in agile development. Cloving can help generate unit tests for your components:
cloving generate unit-tests -f src/components/Button.tsx
Here’s an example of what Cloving might generate:
// src/components/Button.test.tsx
import React from 'react';
import { render, fireEvent } from '@testing-library/react';
import Button from './Button';
describe('Button Component', () => {
it('renders the button with the correct label', () => {
const mockOnClick = jest.fn();
const { getByText } = render(<Button label="Click Me" onClick={mockOnClick} />);
expect(getByText('Click Me')).toBeInTheDocument();
});
it('calls the onClick function when clicked', () => {
const mockOnClick = jest.fn();
const { getByText } = render(<Button label="Click Me" onClick={mockOnClick} />);
fireEvent.click(getByText('Click Me'));
expect(mockOnClick).toHaveBeenCalled();
});
});
Automating Git Commits with Cloving
Another benefit of Cloving is its ability to generate meaningful commit messages for your project’s repository. Instead of creating generic commit messages, you can automate this process:
cloving commit
Cloving will analyze your changes and suggest an informative commit message tailored for your context.
Conclusion
Integrating GPT-powered Cloving into your agile frontend development workflow provides numerous advantages, from generating high-quality TypeScript components to facilitating real-time AI-assisted collaboration. Use Cloving to enhance your productivity, focus on core programming tasks, and drive your projects toward success. Embrace the Cloving CLI tool today and see how it transforms your development experience.
Tips:
- Make effective use of the
--files
option to give Cloving context about your project. - Use the
--interactive
option when generating code for real-time iteration and revision. - Regularly update your Cloving configurations to ensure you are using the optimal AI model for your needs.
By understanding and utilizing Cloving’s features, you can elevate your agile TypeScript frontend development process, resulting in high-quality, scalable, and efficient code.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.