Improving Test Coverage in Your Node Codebase with GPT
Updated on June 26, 2024

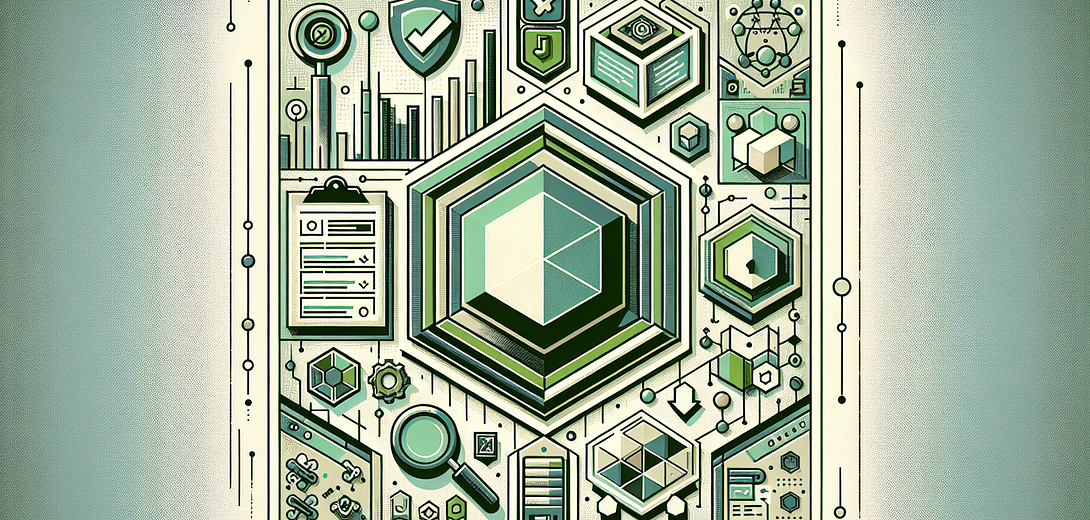
In the dynamic and ever-evolving world of software development, maintaining high test coverage is crucial for ensuring the robustness and reliability of applications. Yet, writing unit tests can be a tedious and time-consuming task for developers.
By adopting the principles of cloving—integrating human creativity and intuition with the powerful capabilities of artificial intelligence (AI)—programmers can streamline and enhance their testing workflows.
In this blog post, we’ll explore how AI tools like GPT can help you improve test coverage in your Node.js codebase, making your programming tasks more efficient and effective.
Understanding Cloving
Cloving combines human intuition and creativity with AI’s analytical prowess to achieve common goals. It’s not just about using AI tools; it’s about creating a symbiotic relationship where human and machine strengths are leveraged to solve problems more effectively.
1. Generating Unit Tests for Functions
Writing unit tests for individual functions can be tedious. GPT can help automate this process by generating relevant test cases based on the given function.
Example:
Suppose you have a calculateDiscount
function in your Node.js application:
function calculateDiscount(price, discount) {
if (price < 0 || discount < 0) {
throw new Error('Price and discount must be non-negative');
}
return price - (price * discount / 100);
}
You can prompt GPT:
Generate unit tests for this Node.js function:
function calculateDiscount(price, discount) {
if (price < 0 || discount < 0) {
throw new Error('Price and discount must be non-negative');
}
return price - (price * discount / 100);
}
GPT will generate tests like:
const assert = require('assert');
const { calculateDiscount } = require('./path/to/your/function');
describe('calculateDiscount', function() {
it('should calculate the discount correctly', function() {
assert.strictEqual(calculateDiscount(100, 10), 90);
assert.strictEqual(calculateDiscount(200, 20), 160);
});
it('should throw an error for negative price', function() {
assert.throws(() => calculateDiscount(-100, 10), /non-negative/);
});
it('should throw an error for negative discount', function() {
assert.throws(() => calculateDiscount(100, -10), /non-negative/);
});
});
2. Improving Existing Tests
GPT can help improve your existing tests by suggesting edge cases and scenario-based tests that you may have overlooked.
Example:
If you already have a test but want to cover more edge cases, you can prompt GPT:
Suggest additional edge cases and test scenarios for this function:
function calculateDiscount(price, discount) {
if (price < 0 || discount < 0) {
throw new Error('Price and discount must be non-negative');
}
return price - (price * discount / 100);
}
GPT might suggest:
- Test with a price of
0
. - Test with a discount of
100%
. - Test with extremely high prices and discounts.
- Test with
NaN
values for price and discount.
3. Documentation and Explanation
Clear documentation can help your team understand your code better, ensuring that test cases are appropriately covering all scenarios. GPT can assist by generating documentation for your functions and tests.
Example:
You can ask GPT to generate documentation:
Generate documentation for this Node.js function and its tests:
function calculateDiscount(price, discount) {
if (price < 0 || discount < 0) {
throw new Error('Price and discount must be non-negative');
}
return price - (price * discount / 100);
}
const assert = require('assert');
describe('calculateDiscount', function() {
it('should calculate the discount correctly', function() {
assert.strictEqual(calculateDiscount(100, 10), 90);
assert.strictEqual(calculateDiscount(200, 20), 160);
});
it('should throw an error for negative price', function() {
assert.throws(() => calculateDiscount(-100, 10), /non-negative/);
});
it('should throw an error for negative discount', function() {
assert.throws(() => calculateDiscount(100, -10), /non-negative/);
});
});
GPT will generate detailed documentation explaining the function and test cases.
4. Refactoring for Testability
Sometimes, improving test coverage requires refactoring your code for better testability. GPT can suggest refactorings that make your code easier to test and maintain.
Example:
To refactor the calculateDiscount
function for better testability, you can prompt GPT:
Suggest refactorings for this function to improve testability:
function calculateDiscount(price, discount) {
if (price < 0 || discount < 0) {
throw new Error('Price and discount must be non-negative');
}
return price - (price * discount / 100);
}
GPT might suggest:
- Splitting the validation logic into a separate function.
- Returning a standard error object instead of throwing errors.
function validatePriceAndDiscount(price, discount) {
if (price < 0 || discount < 0) {
return { isValid: false, message: 'Price and discount must be non-negative' };
}
return { isValid: true };
}
function calculateDiscount(price, discount) {
const validation = validatePriceAndDiscount(price, discount);
if (!validation.isValid) {
throw new Error(validation.message);
}
return price - (price * discount / 100);
}
5. Code Coverage Analysis
Understanding which parts of your code are not covered by tests is crucial for improving test coverage. GPT can help you interpret code coverage reports and suggest areas to focus on.
Example:
After running a code coverage tool like Istanbul, you get a report. You can prompt GPT:
Analyze this code coverage report and suggest areas for improvement:
(line 1) function calculateDiscount(price, discount) { // 85% covered
(line 2) if (price < 0 || discount < 0) { // 100% covered
(line 3) throw new Error('Price and discount must be non-negative'); // 100% covered
(line 4) }
(line 5) return price - (price * discount / 100); // 80% covered
(line 6) }
GPT will analyze the report and suggest focusing on creating tests for scenarios where the function does not receive a discount, ensuring that such cases are also covered.
Conclusion
Improving test coverage in your Node.js codebase with AI assistance exemplifies the power of cloving—combining human creativity and intuition with AI’s analytical capabilities. Integrating GPT into your testing workflow can enhance your productivity, reduce errors, and increase the robustness of your code. Embrace cloving and discover how this synergistic approach can transform your programming experience.
Bonus Follow-Up Prompts
Here are a few extra bonus prompts you could use to refine your workflow:
How can I configure GitHub to run these tests automatically for me?
Generate accompanying factories for example data.
Make the documentation shorter.
By leveraging GPT in these ways, you can further streamline your development process and ensure your Node.js applications are well-tested and reliable.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.