Improve Java Data Structures Using AI-Assisted Code Generation
Updated on March 07, 2025

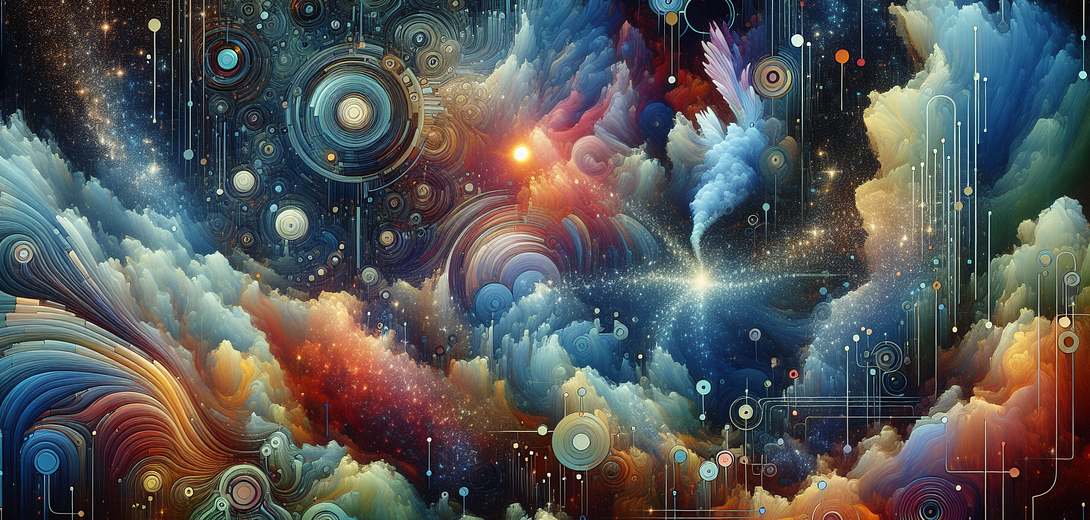
Enhancing Java Data Structures with Cloving CLI
Java offers a rich ecosystem for building robust applications, where data structures are fundamental components that ensure efficient data manipulation and management. However, implementing and optimizing these data structures can be labor-intensive. Cloving CLI, an AI-powered command-line interface, simplifies this process by integrating GPT-based code generation directly into your workflow. This guide will show you how to generate, review, and refine Java data structures using Cloving CLI.
1. Introduction to Cloving CLI
Cloving CLI acts as an AI-assisted pair programmer, helping you:
- Generate boilerplate or custom code for Java data structures
- Refine or extend existing implementations with an interactive AI chat
- Review code for potential improvements and best practices
- Automate repetitive tasks, including the writing of commit messages or test scaffolding
By applying GPT-based intelligence to your codebase, Cloving saves time and ensures consistent quality across your projects.
2. Setting Up Cloving
2.1 Installation
Install Cloving globally via npm:
npm install -g cloving@latest
2.2 Configuration
Run Cloving’s configuration command:
cloving config
You’ll be prompted to enter your API key and choose an AI model (e.g., GPT-3.5, GPT-4). This step enables Cloving to access the AI backend and produce relevant code suggestions.
2.3 Initializing Your Project
Navigate to your Java project’s root directory and initialize Cloving:
cloving init
Cloving analyzes your project’s context (e.g., directory structure, code style), creating a cloving.json
that stores metadata to help the AI produce more accurate outputs.
3. Generating Java Data Structures
3.1 Example: Creating a Binary Search Tree (BST)
Let’s say you need a BST in your Java application. Instead of coding it from scratch, prompt Cloving:
cloving generate code --prompt "Implement a Binary Search Tree in Java" --files src/structure/BST.java
Sample Output:
// src/structure/BST.java
class Node {
int key;
Node left, right;
public Node(int item) {
key = item;
left = right = null;
}
}
public class BST {
Node root;
BST() {
root = null;
}
public void insert(int key) {
root = insertRec(root, key);
}
private Node insertRec(Node root, int key) {
if (root == null) {
root = new Node(key);
return root;
}
if (key < root.key) {
root.left = insertRec(root.left, key);
} else if (key > root.key) {
root.right = insertRec(root.right, key);
}
return root;
}
public void inorder() {
inorderRec(root);
}
private void inorderRec(Node root) {
if (root != null) {
inorderRec(root.left);
System.out.print(root.key + " ");
inorderRec(root.right);
}
}
}
Notes:
- Cloving typically includes methods like
insert
andinorder
traversal. - If you need advanced features (like a
delete
method, balancing logic, or node duplication handling), specify them in your prompt.
3.2 Refining the Generated Data Structure
After generation, you can ask Cloving to add or revise features:
Revise the BST to support removing a node
Cloving may append a delete
method to the class, or refactor existing logic to accommodate node removals.
4. Reviewing and Improving Code
4.1 Interactive Chat
For more intricate tasks (e.g., balancing a BST, implementing an AVL or Red-Black Tree), rely on the Cloving chat:
cloving chat -f src/structure/BST.java
In the chat, you can:
- Ask for performance optimizations
- Request code expansions (e.g.,
getHeight
,isBalanced
, etc.) - Inquire about best practices or performance trade-offs
Example:
cloving> Add a method to check if the BST is balanced
Cloving provides a snippet with logic for measuring subtree heights and confirming balance.
4.2 Code Review
Run Cloving’s AI-driven code review to spot potential improvements:
cloving generate review
Cloving produces an overview of potential issues or refinements, such as missing edge cases or suboptimal recursion patterns.
5. Additional Data Structures
Cloving can generate or refine a wide variety of data structures, including:
- LinkedList (Singly or Doubly Linked)
- Stack or Queue
- HashMap or Set implementations
- Heaps (Min-Heap, Max-Heap)
- Graphs with BFS/DFS capabilities
When generating a new structure, simply be explicit in your prompt:
cloving generate code --prompt "Create a Java PriorityQueue using a Min-Heap" --files src/structure/PriorityQueue.java
6. Testing Your Data Structures
6.1 Generating Unit Tests
To ensure reliability, prompt Cloving to produce test stubs:
cloving generate unit-tests -f src/structure/BST.java
Sample Output:
// src/structure/BSTTest.java
import org.junit.Test;
import static org.junit.Assert.*;
public class BSTTest {
@Test
public void testInsertAndInorder() {
BST tree = new BST();
tree.insert(50);
tree.insert(30);
tree.insert(70);
// You might capture output or verify structure
// For demonstration, a simpler approach:
// e.g., ensure the root is 50, left is 30, right is 70
}
}
Notes:
- Expand tests to cover boundary cases, e.g., inserting duplicate keys, removing nodes, etc.
- If you prefer JUnit 5 or a different framework, mention it in your prompt or show Cloving an existing test file.
7. Streamlining Commits
To keep your version control neat, let Cloving propose commit messages:
cloving commit
Cloving inspects your staged changes (like new data structure classes or test updates) and suggests a succinct commit message. You can accept or refine as needed.
8. Best Practices
- Initialize Properly
Always runcloving init
so the AI can parse your existing code style, package structure, etc. - Iterate
If you need advanced features (e.g., balancing a BST), use multiple prompts or the chat feature to refine the code step by step. - Review
Usecloving generate review
to maintain high standards (e.g., check for potential performance pitfalls or missing edge case handling). - Combine with CI/CD
If you have a continuous integration system, consider using Cloving’s test generation to keep coverage up-to-date, especially if your data structures evolve. - Security and Performance
For specialized needs (like concurrency or extremely large data sets), explicitly mention these constraints in your prompt. Cloving can produce concurrency-safe or performance-optimized approaches if guided properly.
9. Example Workflow
- Initialize:
cloving init
in your Java project. - Generate: e.g.
cloving generate code --prompt "Implement a doubly linked list in Java" -f src/structure/DoublyLinkedList.java
. - Refine:
cloving chat -f src/structure/DoublyLinkedList.java
– ask for methods likeremoveAtIndex
,reverseList
, etc. - Test:
cloving generate unit-tests -f src/structure/DoublyLinkedList.java
. - Review:
cloving generate review
to maintain consistency or spot logic gaps. - Commit:
cloving commit
for an AI-generated commit message.
10. Conclusion
Using Cloving CLI to build and refine Java data structures transforms what’s typically a meticulous coding process into a streamlined, AI-assisted workflow. You can generate sophisticated structures (e.g., BST, heaps, graphs) in seconds, ask Cloving for expansions, and maintain robust test coverage.
Remember: Cloving’s output is a starting point – always validate correctness, performance, and domain-specific requirements. With the synergy of AI and Java engineering, you can focus on designing the rest of your application while Cloving handles the repetitive tasks, ensuring a more efficient and enjoyable development experience.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.