Implementing React Hooks Efficiently with GPT
Updated on July 01, 2024

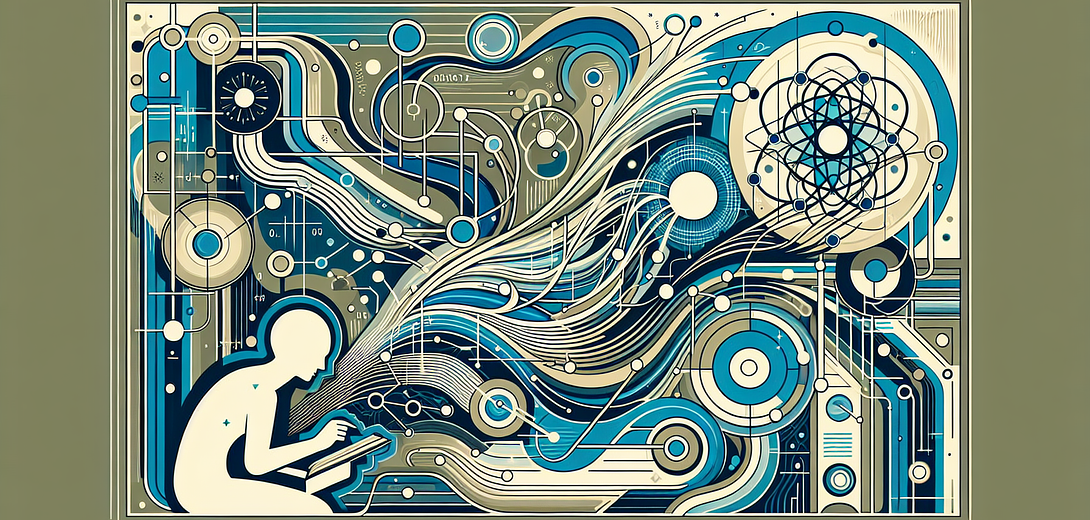
Creating React hooks can often be repetitive and time-consuming, demanding both creativity and precision. By integrating human creativity with the powerful capabilities of artificial intelligence, programmers can streamline their development workflows.
In this post, we’ll explore how AI tools like GPT can assist you in implementing code generation (codegen) for efficient React hook creation, making your programming tasks more efficient and effective.
Understanding Cloving
Cloving combines human intuition and creativity with AI’s analytical prowess to achieve common goals. It’s not just about using AI tools; it’s about creating a symbiotic relationship where human and machine strengths are leveraged to solve problems more effectively.
1. Generating Initial Hook Boilerplate
When starting a new React hook, the initial setup can be tedious. GPT can help by generating the boilerplate code for you, allowing you to focus on the unique logic needed for your specific functionality.
Example:
Suppose you need a hook to manage form state:
Generate a React hook to manage form state, including initial values, field changes, and form submission.
GPT will generate a boilerplate for your form hook:
import { useState } from 'react';
const useForm = (initialValues) => {
const [formValues, setFormValues] = useState(initialValues);
const handleChange = (e) => {
const { name, value } = e.target;
setFormValues({
...formValues,
[name]: value
});
};
const handleSubmit = (callback) => (e) => {
e.preventDefault();
callback(formValues);
};
return {
formValues,
handleChange,
handleSubmit
};
};
export default useForm;
2. Implementing Complex Logic
When creating hooks with complex logic, GPT can assist by providing a starting point or by suggesting snippets of code to include. This speeds up development and ensures that best practices are followed.
Example:
If you need a hook to fetch data from an API and handle loading and error states:
Create a React hook to fetch data from an API endpoint, including loading and error states.
GPT will give you a comprehensive hook:
import { useState, useEffect } from 'react';
import axios from 'axios';
const useFetch = (url) => {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
const fetchData = async () => {
setLoading(true);
try {
const response = await axios.get(url);
setData(response.data);
setError(null);
} catch (err) {
setError(err);
}
setLoading(false);
};
fetchData();
}, [url]);
return { data, loading, error };
};
export default useFetch;
3. Incorporating Best Practices
GPT can help you stay up-to-date with the latest best practices and design patterns for React hooks, ensuring your code is efficient and maintainable.
Example:
To learn more about best practices for creating React hooks, you can ask:
What are the best practices for creating React hooks in 2024?
GPT will provide a comprehensive list of tips such as using custom hooks only when necessary, handling side effects properly, and maintaining state immutability.
4. Generating Unit Tests
Testing ensures that your hooks work as expected. Writing tests for hooks can be cumbersome, but GPT can help generate test cases, saving you time and effort.
Example:
If you have written a hook and need to create tests using React Testing Library, you can prompt GPT:
Generate unit tests for this React hook using React Testing Library: [code snippet of the hook].
GPT will produce relevant tests, ensuring your hook is thoroughly tested.
import { renderHook, act } from '@testing-library/react-hooks';
import useForm from './useForm';
test('should update form values on change', () => {
const initialValues = { name: '' };
const { result } = renderHook(() => useForm(initialValues));
const event = { target: { name: 'name', value: 'John Doe' } };
act(() => {
result.current.handleChange(event);
});
expect(result.current.formValues.name).toBe('John Doe');
});
test('should call callback on form submit', () => {
const initialValues = { name: '' };
const onSubmit = jest.fn();
const { result } = renderHook(() => useForm(initialValues));
const event = { preventDefault: jest.fn() };
act(() => {
result.current.handleSubmit(onSubmit)(event);
});
expect(onSubmit).toHaveBeenCalledWith({ name: '' });
});
5. Documenting Your Hooks
Keeping your code well-documented makes it easier for you and your team to understand and maintain it. GPT can assist by generating clear documentation for your hooks.
Example:
When you need to document a complex hook, you can ask GPT:
Generate documentation for this React hook: [code snippet].
GPT will create detailed documentation, making it clear how to use the hook.
## useForm Hook
This hook manages form state, including initial values, field changes, and form submission.
### Parameters
- `initialValues`: An object that contains the initial values for the form fields.
### Returns
- `formValues`: An object containing the current form values.
- `handleChange`: A function to handle field changes.
- `handleSubmit`: A function to handle form submission.
### Example Usage
```javascript
import useForm from './useForm';
const initialValues = { name: '' };
const MyFormComponent = () => {
const { formValues, handleChange, handleSubmit } = useForm(initialValues);
const submitForm = (values) => {
console.log(values);
};
return (
<form onSubmit={handleSubmit(submitForm)}>
<input type="text" name="name" value={formValues.name} onChange={handleChange} />
<button type="submit">Submit</button>
</form>
);
};
Conclusion
Implementing codegen with AI for efficient React hook creation exemplifies the power of cloving—combining human creativity and intuition with AI’s analytical capabilities. Integrating GPT into your React workflow can enhance your productivity, reduce errors, and keep up with best practices. Embrace cloving and discover how this synergistic approach can transform your programming experience.
Bonus Follow-Up Prompts
Here are a few extra bonus prompts you could use to refine the prompts you see above:
How can I configure GitHub to run these tests automatically for me?
And here is another:
Generate accompanying factories for example data.
And one more:
What are other GPT prompts I could use to make this more efficient?
By leveraging these additional prompts, you can continue to optimize your workflow and maximize the benefits of cloving in your React development projects.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.