Implementing Rate Limiting Strategies for APIs Using GPT
Updated on October 10, 2024

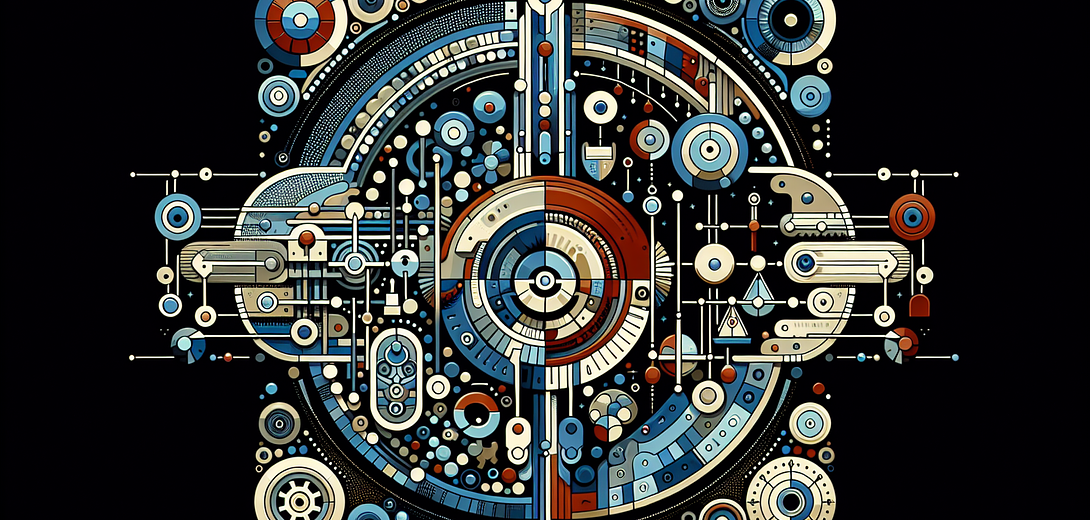
API rate limiting is a fundamental requirement for ensuring that your application remains robust, performant, and secure. By controlling the number of requests a client can make within a specified timeframe, you can prevent abuse, manage traffic, and improve overall service reliability. The Cloving CLI tool, powered by AI, can significantly streamline the process of implementing rate limiting strategies in your API.
In this tutorial, we’ll walk you through how to use Cloving CLI to efficiently implement rate limiting in your APIs, boosting performance and protecting your resources.
Setting Up Cloving for Your API Project
1. Installation and Configuration
Let’s start by installing Cloving CLI and configuring it to assist us in implementing rate limiting.
Installation:
npm install -g cloving@latest
Configuration:
Start configuring Cloving to use your preferred AI settings:
cloving config
Follow the prompts to configure your AI model and preferences. This setup will allow Cloving to understand your project context and generate code that aligns with it.
2. Initializing Your API Project with Cloving
To let Cloving grasp the full context of your API project, initialize it in your project directory:
cloving init
This command will create a cloving.json
file in your project directory, containing metadata that the AI can use to tailor its assistance.
Implementing Rate Limiting with Cloving
3. Generating Rate Limiting Middleware
We’ll be implementing a simple request rate limiting strategy using the Cloving CLI. For this example, let’s assume you’re working on an Express.js-based API.
Use Cloving to Generate Middleware:
cloving generate code --prompt "Create a rate limiting middleware for an Express.js API that limits requests to 100 per hour per IP" --files path/to/api.js
This command prompts Cloving to generate a middleware function for you. You might receive an output similar to the following [code snippet]:
const rateLimit = require('express-rate-limit');
const limiter = rateLimit({
windowMs: 60 * 60 * 1000, // 1 hour
max: 100, // limit each IP to 100 requests per windowMs
message: 'Too many requests from this IP, please try again after an hour',
});
module.exports = limiter;
Integrating the Middleware into Your Application:
const express = require('express');
const limiter = require('./path/to/limiter');
const app = express();
// Apply the rate limiting middleware to all requests
app.use(limiter);
app.get('/api/', (req, res) => {
res.send('Hello, World!');
});
app.listen(3000, () => console.log('API running on port 3000'));
4. Review and Adjust the Generated Code
Use Cloving CLI to review and tweak the generated code as needed. You can request a revision if you need modifications.
To adjust the rate limiting rules to limit requests to 200 per 30 minutes, initiate a cloving chat session specifying the same file as earlier:
cloving chat --file path/to/api.js
🍀 🍀 🍀 Welcome to Cloving REPL 🍀 🍀 🍀
Type a freeform request or question to interact with your Cloving AI pair programmer.
Available special commands:
- save Save all the changes from the last response to files
- commit Commit the changes to git with an AI-generated message that you can edit
- copy Copy the last response to clipboard
- review Start a code review
- find <file-name> Find and add files matching the name to the chat context (supports * for glob matching)
- add <file-path> Add a file to the chat context (supports * for glob matching)
- rm <pattern> Remove files from the chat context (supports * for glob matching)
- ls <pattern> List files in the chat context (supports * for glob matching)
- git <command> Run a git command
- help Display this help message
- exit Quit this session
What would you like to do?
cloving> Adjust the rate limiting rules to limit requests to 200 per 30 minutes.
Certainly! To do this I will modify the code in path/to/api.js
Cloving will provide the adjusted block of code, which you can directly implement in your project.
5. Testing Your Rate Limiting Strategy
After integrating and adjusting your middleware, test it to ensure it behaves as expected.
Use Cloving to Generate Unit Tests:
You can generate unit tests for your middleware to ensure your rate limiting is applied correctly.
cloving generate unit-tests -f path/to/limiter.js
This command generates test cases that simulate various scenarios, giving you confidence that your implementation is correct.
const request = require('supertest');
const express = require('express');
const limiter = require('../path/to/limiter');
const app = express();
app.use(limiter);
test('should limit requests after 100 attempts', async () => {
for (let i = 0; i < 100; i++) {
await request(app).get('/api/').expect(200);
}
await request(app).get('/api/').expect(429).expect('Too many requests from this IP, please try again after an hour');
});
Conclusion
Implementing rate limiting is a critical part of building scalable and reliable APIs. With the help of Cloving CLI, you can efficiently produce robust rate limiting middleware, ensure proper configuration, and validate through automated testing. This significantly reduces development time and enhances the quality and reliability of your applications.
By integrating Cloving into your workflow, you empower your development process with AI-driven insights and code generation, helping you stay ahead in implementing complex features like API rate limiting with ease and precision.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.