Implementing Efficient Logging Mechanisms in JavaScript Using GPT
Updated on November 06, 2024

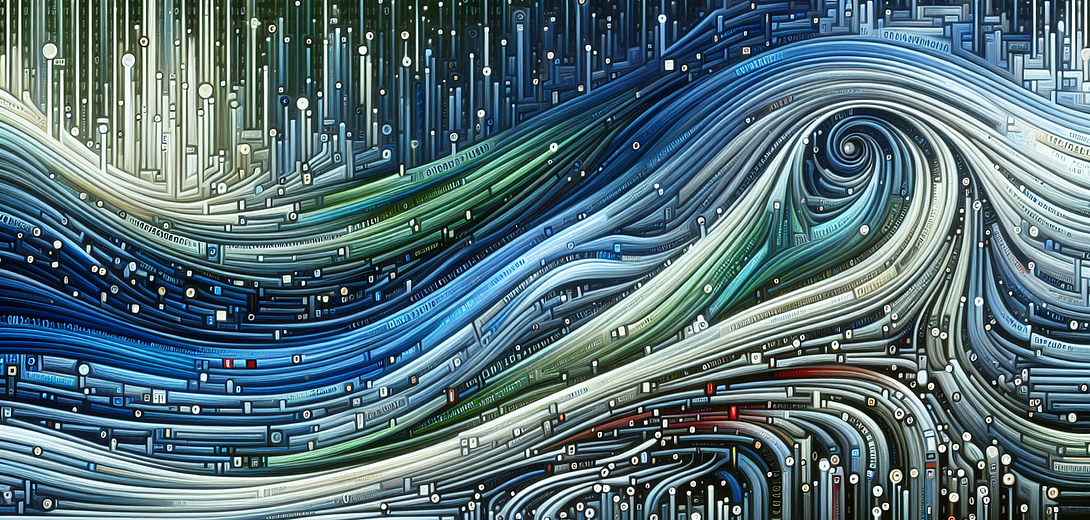
In the modern software development ecosystem, effective logging is paramount to understanding application behavior, debugging, and performance monitoring. The Cloving CLI tool, powered by AI, offers an invaluable asset for programmers seeking to implement efficient logging mechanisms in JavaScript. In this blog post, we’ll dive into practical examples and best practices for integrating AI-powered logging with the Cloving CLI to enhance your applications.
Getting Started with Cloving CLI
Before we delve into creating logging mechanisms, we need to ensure the Cloving CLI tool is seamlessly integrated into our development environment.
Installation
First, install Cloving globally using npm:
npm install -g cloving@latest
Configuration
Next, configure Cloving to utilize your AI model preferences:
cloving config
Follow the on-screen prompts to input your API key and select your preferred models.
Project Initialization
Initialize Cloving in your JavaScript project directory to provide the AI with context:
cloving init
This step generates a cloving.json
file detailing the application’s metadata, allowing Cloving to tailor its responses to your specific development environment.
Implementing an Efficient Logging Mechanism
Once Cloving is set up, we can leverage its powerful code generation and interaction capabilities to implement efficient logging in JavaScript.
Step 1: Basic Logging Setup
Start by generating a simple logging module using Cloving:
cloving generate code --prompt "Create a basic JavaScript logging module" --files src/logging.js
The generated logging module might look like this:
// src/logging.js
class Logger {
constructor(level = 'info') {
this.level = level;
}
log(message, level = 'info') {
if (this.shouldLog(level)) {
console.log(`[${level.toUpperCase()}] ${message}`);
}
}
shouldLog(level) {
const levels = ['error', 'warn', 'info', 'debug'];
return levels.indexOf(level) <= levels.indexOf(this.level);
}
}
export default new Logger();
Step 2: Adding Contextual Information
Efficient logs should include contextual information. Use Cloving’s interactive chat to request enhancements for adding context to your logs:
cloving chat -f src/logging.js
🍀 🍀 🍀 Welcome to Cloving REPL 🍀 🍀 🍀
cloving> Enhance the logging module to include timestamps and request IDs in each log message.
The AI might revise the module as follows:
// src/logging.js
import { v4 as uuidv4 } from 'uuid';
class Logger {
constructor(level = 'info') {
this.level = level;
this.requestId = uuidv4();
}
log(message, level = 'info') {
if (this.shouldLog(level)) {
const timestamp = new Date().toISOString();
console.log(`[${level.toUpperCase()}] [${timestamp}] [${this.requestId}] ${message}`);
}
}
shouldLog(level) {
const levels = ['error', 'warn', 'info', 'debug'];
return levels.indexOf(level) <= levels.indexOf(this.level);
}
}
export default new Logger();
Step 3: Generating Unit Tests
We should now generate unit tests to ensure the logging module works as intended:
cloving generate unit-tests -f src/logging.js
This command will produce unit tests that may resemble the following:
// src/logging.test.js
import logger from './logging';
describe('Logger', () => {
let consoleSpy;
beforeEach(() => {
consoleSpy = jest.spyOn(console, 'log').mockImplementation(() => {});
});
afterEach(() => {
jest.restoreAllMocks();
});
test('logs messages with info level by default', () => {
logger.log('Informational message');
expect(consoleSpy).toHaveBeenCalledWith(expect.stringContaining('INFO'));
});
test('respects logging level precedence', () => {
logger.level = 'warn';
logger.log('This is an info message', 'info');
expect(consoleSpy).not.toHaveBeenCalled();
});
test('includes timestamp and request ID', () => {
logger.log('Another message');
expect(consoleSpy).toHaveBeenCalledWith(expect.stringMatching(/\[INFO\] \[\d{4}-\d{2}-\d{2}T\d{2}:\d{2}:\d{2}.\d{3}Z\] \[.*/));
});
});
Step 4: Integrating and Saving Changes
If satisfied with the generated code, save changes back into your source files:
cloving> save
Leveraging Cloving for Ongoing Updates
For ongoing improvements and additional features, Cloving can be a continuous AI collaborator through its chat feature. It can be used to troubleshoot, improve existing code, or generate entire new functionalities.
Conclusion
Implementing an efficient logging mechanism in JavaScript with the help of the Cloving CLI tool highlights how AI can enhance your development productivity. From automated code generation to interactive feature enhancement, Cloving empowers you to refine your logging setup with sophisticated AI capabilities.
By incorporating Cloving into your workflow, you’ll boost code quality, simplify maintenance, and optimize application insights, all while minimizing manual coding effort. Embrace Cloving and elevate your JavaScript programming experience to new heights.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.