How to Use GPT for Scala Function Generation
Updated on November 17, 2024

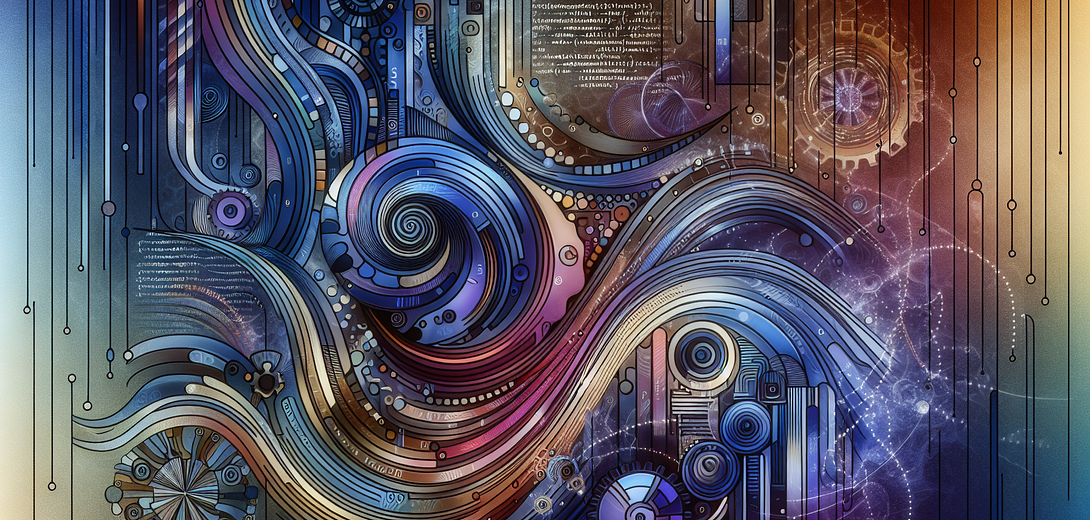
How to Use GPT for Scala Function Generation with Cloving CLI
As Scala developers, leveraging AI to generate functions can significantly boost productivity and help maintain code quality. The Cloving CLI tool offers a seamless way to integrate GPT-powered function generation into your Scala development workflow. In this blog post, we’ll walk through practical steps and best practices for using the Cloving CLI to generate Scala functions efficiently.
Getting Started with Cloving CLI
To begin using Cloving for Scala function generation, you’ll need to ensure it’s correctly installed and configured in your environment.
1. Installation and Configuration
First, install Cloving globally via npm:
npm install -g cloving@latest
Next, configure Cloving to connect with your preferred AI model:
cloving config
Follow the prompts to set up your API key and model preferences.
2. Initializing Your Scala Project
For Cloving to understand your project context, initialize it within your Scala project’s directory:
cloving init
This will create a cloving.json
file that contains metadata about your project and its context.
Generating Scala Functions
Once set up, you can start generating Scala functions using Cloving’s powerful AI capabilities. Here’s how:
3. Practical Function Generation
Suppose you need to create a function that computes the Fibonacci sequence up to a given number. You can achieve this by using the generate code
feature in Cloving:
cloving generate code --prompt "Implement a Scala function to calculate Fibonacci numbers" --files src/main/scala/Fibonacci.scala
Cloving will analyze your request and generate a Scala function based on the provided context. The output may look like this:
// src/main/scala/Fibonacci.scala
def fibonacci(n: Int): List[Int] = {
def fibHelper(x: Int, prev: Int, next: Int): List[Int] = {
if (x <= 0) List()
else prev :: fibHelper(x - 1, next, prev + next)
}
fibHelper(n, 0, 1)
}
4. Interacting with Generated Code
After Cloving generates a Scala function, you can engage with the code by reviewing, revising, or saving it:
- Review: Understand and verify the generated function.
- Revise: Make changes or improvements by communicating directly in the CLI.
- Save: Save to your desired file location.
For example, if you need to revise the Fibonacci function to optimize performance, you can modify your prompt:
Revise the Fibonacci function to optimize its performance
Based on this prompt, the Cloving CLI using your AI model of choice might suggest code that looks something like this:
// Optimized Fibonacci function using memoization
import scala.collection.mutable
def optimizedFibonacci(n: Int): List[Int] = {
val memo = mutable.Map[Int, Int](0 -> 0, 1 -> 1)
def fib(x: Int): Int =
memo.getOrElseUpdate(x, fib(x - 1) + fib(x - 2))
(0 until n).map(fib).toList
}
5. Generating Tests for Scala Functions
Cloving can assist in generating unit tests for the functions you create, ensuring that your code works as expected:
cloving generate unit-tests -f src/main/scala/Fibonacci.scala
This command will produce relevant unit tests for your function, enhancing confidence in its correctness:
// src/main/scala/FibonacciTest.scala
import org.scalatest.funsuite.AnyFunSuite
class FibonacciTest extends AnyFunSuite {
test("Fibonacci of 5") {
assert(Fibonacci.fibonacci(5) == List(0, 1, 1, 2, 3))
}
test("Fibonacci of 0") {
assert(Fibonacci.fibonacci(0) == List())
}
}
6. Continuous Scala Function Enhancement Using Chat
Utilize Cloving’s chat feature for ongoing assistance or when dealing with complex tasks:
$ cloving chat -f src/main/scala/Fibonacci.scala
🍀 Welcome to Cloving REPL 🍀
cloving> Improve the efficiency of the Fibonacci function using memoization
Interacting via chat allows real-time adjustments and nuanced conversations to refine Scala functions promptly.
7. Leveraging Cloving for Git Commits
Optimize your workflow by using Cloving to craft meaningful commit messages. Rather than using git commit
, enter:
cloving commit
This command will auto-generate a commit message reflecting the changes, ensuring clear and concise version history management.
Conclusion
Harnessing the power of GPT through the Cloving CLI for Scala function generation opens up new avenues of efficiency and code quality. By incorporating AI into your development toolbox, you can quickly generate, test, and refine Scala functions to meet your project requirements proficiently. Remember, Cloving is your coding ally, augmenting your skills and fostering a smoother development journey.
Embrace this innovative tool and transform your Scala programming experience today!
Explore the potential of integrating Cloving into your Scala workflow and discover how AI can catalyze your coding productivity.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.