How to Integrate Algolia in Your Rails App Using GPT
Updated on June 26, 2024

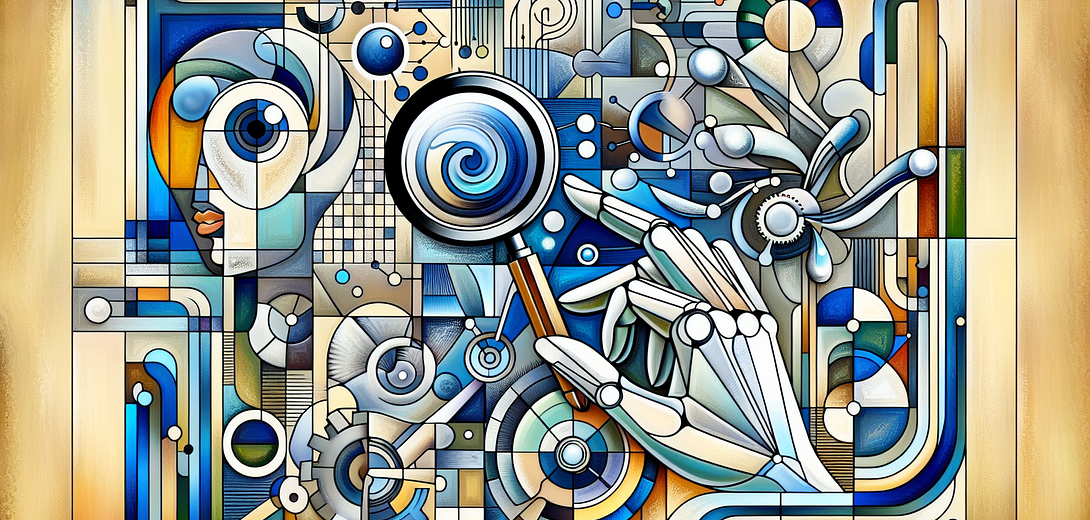
The fusion of human creativity and intuition with the analytical power of artificial intelligence (AI) creates a potent mix known as cloving.
By incorporating GPT, an AI language model, into your workflow, you can streamline your development process, particularly when dealing with the integration of complex services like Algolia.
In this blog post, we will explore how you can leverage GPT to efficiently integrate Algolia into your Rails app, making your development tasks easier and more effective.
Understanding Cloving
Cloving isn’t just about using AI tools; it’s about forming a partnership where human and machine strengths complement each other to achieve a common goal. This blog post will guide you through various ways to integrate Algolia into your Rails app using GPT, demonstrating how cloving can enhance your programming workflow.
1. Setting Up Algolia
Setting up Algolia involves several steps, from creating an account to configuring API keys. GPT can assist by providing step-by-step guidance and code snippets.
Example:
Create an Algolia account and get your API keys. Once you have them, you can ask GPT for help in configuring these keys in your Rails app:
I have my Algolia application ID and API key. How do I configure them in my Rails app?
GPT will respond with detailed instructions:
# Add the 'algoliasearch-rails' gem to your Gemfile
gem 'algoliasearch-rails'
# Run bundle install
$ bundle install
# Create a configuration file: config/initializers/algoliasearch.rb
AlgoliaSearch.configuration = {
application_id: Rails.application.credentials[:algolia][:application_id],
api_key: Rails.application.credentials[:algolia][:api_key]
}
To set the application_id
and api_key
in your Rails secret credentials, use the following:
$ EDITOR="nano" rails credentials:edit
Add your Algolia credentials like this:
algolia:
application_id: 'YourApplicationID'
api_key: 'YourAPIKey'
2. Adding AlgoliaSearch to Models
Integrating Algolia with your models involves adding the necessary configuration to enable indexing. GPT can help you generate the required configurations and methods.
Example:
Suppose you have a Product
model that you want to index with Algolia. You can ask GPT for assistance:
How do I integrate AlgoliaSearch with my Product model in Rails?
GPT will provide the following instructions:
class Product < ApplicationRecord
include AlgoliaSearch
algoliasearch do
# List of attributes to index
attributes :name, :description, :price
# Attributes to be used for search
searchableAttributes ['name', 'description']
end
end
3. Indexing Existing Records
After configuring your model, you need to index existing records. GPT can help by providing the necessary rake tasks or console commands.
Example:
You can prompt GPT for a rake task to index existing Product
records:
How do I index existing Product records with Algolia in my Rails app?
GPT will guide you:
# Create a rake task in lib/tasks/algolia.rake
namespace :algolia do
desc 'Reindex all products'
task reindex_products: :environment do
Product.reindex
puts 'All products have been reindexed.'
end
end
# Run the task:
$ rake algolia:reindex_products
4. Implementing Search Functionality
Once your data is indexed, you’ll need to implement search functionality. GPT can help generate the appropriate controller methods and views.
Example:
To create a search method in your controller, you can ask GPT:
How do I create a search method in my Rails controller to search for products using Algolia?
GPT will provide the following code:
class ProductsController < ApplicationController
def search
if params[:query].present?
@products = Product.search(params[:query])
else
@products = Product.all
end
end
end
For the view:
<!-- app/views/products/search.html.erb -->
<%= form_with(url: search_products_path, method: :get, local: true) do %>
<%= text_field_tag :query, params[:query], placeholder: 'Search products...' %>
<%= submit_tag 'Search' %>
<% end %>
<% @products.each do |product| %>
<p><%= product.name %> - <%= product.price %></p>
<% end %>
5. Testing and Debugging
Testing your search functionality is crucial for ensuring it works correctly. GPT can assist in generating RSpec tests for your search methods.
Example:
To generate RSpec tests for your search method, you can prompt GPT:
Generate RSpec tests for the search method in ProductsController.
GPT will produce the following test cases:
require 'rails_helper'
RSpec.describe ProductsController, type: :controller do
describe 'GET #search' do
let!(:product1) { create(:product, name: 'Apple iPhone') }
let!(:product2) { create(:product, name: 'Samsung Galaxy') }
it 'returns results for a valid search query' do
get :search, params: { query: 'Apple' }
expect(assigns(:products)).to match_array([product1])
end
it 'returns all products when search query is empty' do
get :search
expect(assigns(:products)).to match_array([product1, product2])
end
end
end
Conclusion
Integrating Algolia into your Rails app can be a complex process, but by embracing the principles of cloving and leveraging GPT, you can significantly simplify and enhance your workflow. From initial setup and model configuration to implementing search functionality and testing, GPT can provide invaluable assistance, allowing you to focus on the creative aspects of your development work. Embrace cloving and see how it transforms your programming experience.
Bonus Follow-Up Prompts
Here are a few extra bonus prompts you could use to refine your workflows:
How can I configure GitHub Actions to run tests automatically for this integration?
Here is another:
Generate FactoryBot factories for example data in my tests.
And one more:
What are other useful GPT prompts I could use to make this Algolia integration more efficient?
Using these prompts will further demonstrate the power of cloving, making your integration tasks even smoother and more efficient. Happy coding!
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.