How to Implement i18n in a Vue App Using GPT
Updated on July 03, 2024

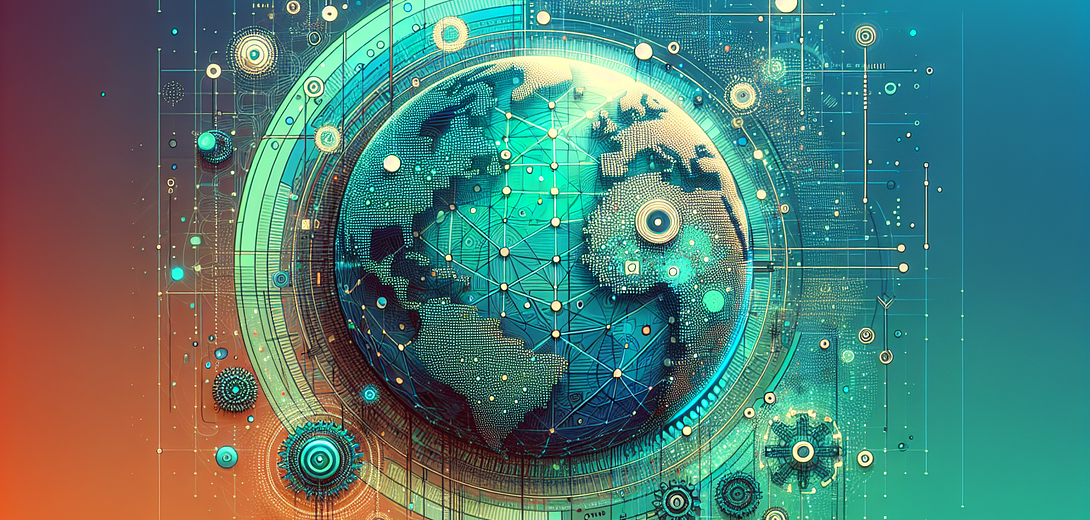
In this blog post, we’ll explore how you can harness the power of GPT (Generative Pre-trained Transformer) to implement internationalization (i18n) in your Vue.js applications. This approach will not only make the process more efficient but will also help you achieve a more robust and scalable solution.
Understanding Cloving in Software Development
Before diving into the technical details, let’s briefly discuss cloving. Cloving is the practice of integrating human intuition and creativity with AI’s analytical prowess to achieve common goals more effectively. The aim is to create a synergistic relationship where both human and machine capabilities are leveraged to solve problems more efficiently.
1. Setting Up i18n in Vue with GPT
Setting up i18n in a Vue.js application typically involves multiple steps, including library installation, configuration, and managing translation files.
Example:
First, let’s start with the installation. You can ask GPT to provide you with the necessary commands and basic setup instructions:
How do I set up internationalization in a Vue application? Provide the installation commands and basic configuration code.
GPT will respond with:
To set up Vue-i18n in a Vue.js application, follow these steps:
1. Install the package:
```bash
npm install vue-i18n
- Initialize it in your Vue application:
// src/main.js
import { createApp } from 'vue';
import App from './App.vue';
import { createI18n } from 'vue-i18n';
import translations from './locales';
const i18n = createI18n({
locale: 'en', // default locale
fallbackLocale: 'en',
messages: translations, // your translation files
});
const app = createApp(App);
app.use(i18n);
app.mount('#app');
2. Managing Translation Files
Creating and managing translation files can be cumbersome. GPT can assist in generating the initial structure and guide you through best practices.
Example:
Let’s add some sample translation files for English and French.
Generate translation files for English and French, including common phrases for a Vue.js application.
GPT will respond with:
Here are sample translation files for English and French:
```js
// src/locales/en.json
{
"greeting": "Hello",
"farewell": "Goodbye",
"home": {
"title": "Welcome to Our Site"
}
}
// src/locales/fr.json
{
"greeting": "Bonjour",
"farewell": "Au revoir",
"home": {
"title": "Bienvenue sur notre site"
}
}
3. Dynamic Translation Switching
Dynamic translation switching is another aspect where GPT can assist. It’s important to switch languages easily based on user actions.
Example:
Prompt GPT to provide a basic implementation of language switching in a Vue component.
Provide a Vue component code example to switch between languages dynamically.
GPT will answer with:
Here's a basic example of a Vue component to switch between languages:
```vue
<template>
<div>
<h1>{{ $t('home.title') }}</h1>
<button @click="changeLanguage('en')">English</button>
<button @click="changeLanguage('fr')">Français</button>
</div>
</template>
<script>
export default {
name: 'LanguageSwitcher',
methods: {
changeLanguage(lang) {
this.$i18n.locale = lang;
}
}
}
</script>
4. Optimizing and Debugging
Cloving is all about leveraging AI for repetitive and error-prone tasks while using human creativity to fine-tune and debug. When running into issues, GPT can assist with debugging and optimization tips.
Example:
If you encounter issues, you might ask GPT for help:
I'm experiencing issues with translations not updating dynamically in my Vue app. Can you help debug this?
GPT will provide helpful diagnostic steps, such as:
To debug translation issues:
1. Ensure your locale files are correctly loaded and structured.
2. Verify that the `i18n` instance is correctly injected.
3. Check browser console for errors and warnings related to `vue-i18n`.
4. Ensure your component correctly calls `this.$i18n.locale`.
Here's a quick checklist:
- Are the locale files correctly imported?
- Is the fallback locale configured?
- Are the bindings `wired` correctly in the Vue components?
Fixing these common issues should resolve the translation update problem.
5. Automatically Extracting Text Components with GPT
To further illustrate how GPT can help with i18n, let’s take a simple “Hello World” Vue component and ask GPT to suggest extracting the text components into translation files.
You can prompt like this:
Here's the `HelloWorld` Vue component:
```vue
<template>
<div>
<h1>Hello World</h1>
<p>Welcome to our application!</p>
</div>
</template>
<script>
export default {
name: 'HelloWorld'
}
</script>
Suggest how to extract the text from this `HelloWorld` component for i18n.
GPT will respond with:
To extract text for i18n from your `HelloWorld` component, follow these steps:
1. Add the text keys to your translation files.
```js
// src/locales/en.json
{
"helloWorld": "Hello World",
"welcomeMessage": "Welcome to our application!"
}
// src/locales/fr.json
{
"helloWorld": "Bonjour le monde",
"welcomeMessage": "Bienvenue dans notre application!"
}
2. Modify the `HelloWorld` component to use the `$t` function for translations.
```vue
<template>
<div>
<h1>{{ $t('helloWorld') }}</h1>
<p>{{ $t('welcomeMessage') }}</p>
</div>
</template>
<script>
export default {
name: 'HelloWorld'
}
</script>
Conclusion
By implementing cloving in your i18n workflow, you can significantly boost your efficiency and effectiveness. GPT offers valuable assistance at every step, from initial setup and configuration to generating translation files and debugging. Embrace this symbiotic relationship between human creativity and AI capabilities, and see how it transforms the way you develop and maintain multilingual Vue.js applications.
Bonus Follow-Up Prompts
Here are additional prompts you could use to refine your work:
Generate best practices for maintaining translation files in a Vue.js application.
And another one:
How can I set up unit tests to ensure translations are correctly loaded in my Vue components?
And one more:
What are some other GPT prompts to help manage large translation projects?
By integrating these prompts into your daily workflows, you’ll harness the true power of cloving, making your development process smoother and more exciting.
Subscribe to our Newsletter
This is a weekly email newsletter that sends you the latest tutorials posted on Cloving.ai, we won't share your email address with anybody else.